gtk guiAs you might’ve noticed, the gtk3-rs projects are getting less and less attention and we intend to deprecate them in one of the future releases. Therefore, we recommend to anyone who didn’t upgrade to GTK4 to do it now. gtk3-rs will get further releases in the foreseeable future to keep up with gtk-rs-core, but no development effort is going to be directed towards it. This is already the de-facto situation for more than a year. Additionally, the GTK3 versions of various externally maintained bindings will most likely not get any further releases.
In addition to gtk-rs, various externally maintained bindings also had a new release. For gstreamer-rs you can find the CHANGELOG of the 0.20 release here. Most bindings maintained as part of GNOME were also updated.
On this note, time to go through the major changes of this release. Enjoy!
Crates
Notable new or updated crates. Posts 
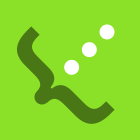
guiRecently I found myself in need of doing GUI with Rust, so I researched and experimented a bit. In this post, I want to share the data I collected, as it might help others. I will mostly compare Tauri, Iced and egui as those seem to be popular choices. I spent quite a bit of time performing tests to assess the speed/performance of these libraries, as a snappy UI experience is something I really value.
Today, 1Password is making Typeshare publicly available as an open-source project to help Rust developers generate consistent type schema across multiple languages.
Decoupling the display code from our business logic gives us cross-platform consistency while also letting 1Password look great on any device. But the frontends are written in a different language, so we use a foreign function interface to communicate with the frontends.
But we needed to ensure the data we gave to the frontend was understood correctly - if the data types between the languages weren’t in sync, it would result in a host of problems. Typeshare was the solution, and today it helps our backend developers rapidly develop new features and fixes without fear of breaking consistency with our display code.
Hello! Ndeewo! Molweni! Салам! Across the world, people are coming online with smartphones, smart watches, and other small, low-resource devices. The technology industry needs an internationalization solution for these environments that scales to dozens of programming languages and thousands of human languages.
Enter ICU4X. As the name suggests, ICU4X is an offshoot of the industry-standard i18n library published by the Unicode Consortium, ICU (International Components for Unicode), which is embedded in every major device and operating system.
In this blog post, I want to talk about Minus, which is a cross-platform, asynchronous terminal paging library written in Rust.
So, I’m ready to announce that I’ve finished working on a new Ruby parser. It’s called lib-ruby-parser. Key features:
1. It’s fast.
2. It has a beautiful interface.
3. It’s precise.
4. It doesn’t depend on Ruby.
This article will explore the thread-per-core model with its advantages and challenges, and introduce Scipio (you can also find it on crates.io), our solution to this problem. Scipio allows Rust developers to write thread-per-core applications in an easy and manageable way.
audioOver the last few weeks I ported the libebur128 C library to Rust, both with a proper Rust API as well as a 100% compatible C API.
We are pleased to announce the next major release for actix-web, v3.0! This is the safest, most stable version yet. We highly recommend everyone upgrade to v3 ASAP to benefit from these improvements. Further, v3 introduces several other improvements with minimal breaking changes.
As of this week, Piet has a new API for creating text layout objects. This supports basic multi-line rich text, and has implementations that work on windows (via DirectWrite) and macOS (via CoreText). Linux support is currently limited by the fact that we’re still using the Cairo ‘toy text’ API; we will get a proper implementation there at some point.
In this post I would like to go over a few particular aspects of this API, and touch briefly on the constraints that led us to these decisions.
We have just released version 0.19.0 of Sequoia. The release includes the low-level crate sequoia-openpgp, a program to verify detached signatures geared towards software distribution systems called sqv, and a commandline frontend for Sequoia implementing the Stateless OpenPGP Command Line Interface called sqop.
error-handlingThis post describes my more-than-a-month long story of refactoring existing error definitions and error handling code in my largest project written in Rust. DICOM-rs is a pure Rust implementation of the DICOM standard, the near-ubiquitous standard for representing and communicating medical imaging data. The refactor hereby described on this project was an immediately apparent improvement, enabling me to track down the cause of a recently discovered bug in one of its crates.
Serialization has always been a strong point of Rust. In particular, Serde was available well before Rust 1.0.0 was released (though the derive macro was unstable until 1.15.0). The idea behind this is to use traits to decouple the objects and (de)serialize from the serialization format — a very powerful idea. Format writers only need to implement Serde’s (de)serializer traits and users can #[derive(Serialize, Deserialize)] to get serialization for their objects, regardless of the format.
Of course, there are format-specific crates, such as protocol buffers, bincode, FlatBuffers, etc. Those can offer good compile-time and runtime performance, but they lock the data into their respective protocols, often with implementations available in other languages. For many uses, and especially in polyglot environments, this is an acceptable tradeoff.
In this guide, we’ll zoom in on both kinds of frameworks, considering API usability and performance.
In our announcement last week, we briefly mentioned this new physics engine we have been working on during the past 5 months. Today we are officially releasing it for the first time: the project Rapier; a set of two 100% rust libraries rapier2d and rapier3d for 2D and 3D physics simulations for games, animation, and robotics.
asyncRust's approach to bringing async code into the language is novel, in that instead of packaging the async system with the language, such as Golang's approach of providing in-built goroutines, it presents an interface to be used by independent crate developers to implement the async runtime for a given process. It also separates the job of the executor or runtime (which polls the futures) and the reactor (which tells the executor when the futures should be polled). Out of this interface, a number of projects have emerged.
async tokioI am pleased to announce the release of async-raft v0.5.0. This release has been a great deal of effort, lots of early mornings and late nights. I am VERY pleased with the quality of the code and how things have come together. The Raft API couldn't be more simple, async/await has absolutely simplified and clarified so many of the interfaces throughout this project, and the many excellent tools available through Tokio have greatly helped with code quality overall.
libz-sys 8 provides Rust bindings to the zlib compression library, for handling DEFLATE/gzip compression. I've just released libz-sys 1.1.0, which provides opt-in support for the high-performance zlib-ng library in zlib-compat mode.
zlib-ng provides 2-3x performance improvements for compression, and ~20% improvements for decompression.
Three years ago, I created Rustsim in order to group all the Rust projects I have been working on and maintaining since 2013. Creating this organization on GitHub was one step further towards securing the future existence and maintenance of these Rust crates, some of them being quite popular today like nalgebra or nphysics. Today, I am going one step further and create my company named Dimforge.
After a little over 6 months, bincode 1.3 is finally here! This version is a big milestone. It brings many new features and bugfixes, as well as introducing new maintainers and a roadmap.
asyncI used to be afraid of async Rust. It's easy to get into trouble!
But thanks to the work done by the whole community, async Rust is getting easier to use every week. One project I think is doing particularly great work in this area is async-std.
Dropbox syncs petabytes of data every day across millions of desktop clients. It is vital that we constantly improve the sync experience for our users, to increase our users’ productivity in their everyday lives. We also constantly strive to better leverage our infrastructure, increasing Dropbox’s operational efficiency. Because the files themselves are being sent to and from Dropbox during sync, we can leverage redundancies and recurring patterns in common file formats to send files more tersely and consequentially improve performance. Modern compression techniques identify and persist these redundancies and patterns using short bit codes, to transfer large files as smaller, losslessly compressed, files. Thus, compressing files before syncing them means less data on the wire (decreased bandwidth usage and latency for the end user!) and storing smaller pieces in the back end (increased storage cost savings!). To enable these advancements, we measured several common lossless compression algorithms on the incoming data stream at Dropbox including 7zip, zstd, zlib, and Brotli, and we chose to use a slightly modified Brotli encoder, we call Broccoli, to compress files before syncing.
Today, we will dive into the Broccoli encoding, provide an overview of the block sync protocol, and explain how we are using them in conjunction to optimize block data transfers. All together, these improvements have reduced median latencies by more than 30% and decreased data on the wire by the same amount. We will talk about the principles we kept in mind and what we have learned along the way. We will also touch upon how our new investments can help us in the future.
I’ve just released a new crate called propane, which is a library for writing generator functions.
The world of networking has undergone monumental shifts over the past decade, particularly in the ongoing move from specialized hardware into software defined network functions (NFV) for data plane1 and packet processing. While the transition to software has fashioned the rise of SDN (Software-defined networking) and programmable networks, new challenges have arisen in making these functions flexible, efficient, easier to use, and fast (i.e. little to no performance overhead). Our team at Comcast wanted to both leverage what the network does best, especially with regards to its transport capacity and routing mechanisms, while also being able to develop network programs through a modern software lens—stressing testing, swift iteration, and deployment. So, with these goals in mind, we developed Capsule, a new framework for network function development, written in Rust, inspired by Berkeley's NetBricks research, and built-on Intel's Data Plane Development Kit (DPDK).
Polars is a DataFrames library implemented in Rust, using Apache Arrow as backend. It's focus is being a fast in memory DataFrame library that only supports core functionality.
The new geos version is now here! As a reminder, geos is a binding of the C++ geos library which provides tools to perform geospatial operations. I'm very proud of this release for a few reasons that I'll now describe.
debuggerAbout 3 weeks ago, we started a new project to create a new modular and composable debugger library for Rust, taking inspiration from projects such as Delve and MDB. In less than 4 weeks, we have already seen some exciting progress!
Virtually all web apps require some form of authentication, especially in an age of ever-increasing awareness around data privacy. Rust has a wide and growing range of high-quality, production-ready crates available for authentication and authorization.
In this guide, we’ll evaluate nine stable, production-ready authentication libraries based on the following criteria:
* Popularity
* Completeness
* Maintenance
* Support for stable RustWe’ll also preview some crates that aren’t yet production-ready but should be on your radar.
pythonThis post is a quick walkthrough of how I wrote a Python library, procmaps, in nothing but Rust. It uses PyO3 for the bindings and maturin to manage the build (as well as produce manylinux1-compatible wheels).
gdbstub is an ergonomic and easy-to-integrate implementation of the GDB Remote Serial Protocol written in Rust.
gdbstub is particularly well suited for emulation, making it easy to add powerful, non-intrusive debugging support to an emulated system. Just provide an implementation of gdbstub::Target for your target platform, and you're ready to start debugging!
database postgresqlPostgreSQL JSONB columns are quite handy, there many times that I use them actually. I usually employ JSONB for (meta)data that even if they get lost, we are fine, we just need to do more manual work.
The usual cases include:
* caching data
* shortening relations, so instead of going table1 -> table2 -> table3 -> table4, you can store the table4 id inside table1 jsonb meta column to shorten the path (and vice versa)
* metadata that we want to store, but don’t deserve a dedicated column.Working lately in Rust, I was trying to figure out how can I use a JSONB column with diesel. It turns out that it’s not that difficult actually!
Persy is an attempt to write a transactional storage engine in Rust. It has been quite some time since the last release and this release pack quite a lot of changes in it, not exactly following the planned path, but still a nice set of changes.
gui windowsNative-Windows-Gui (aka NWG) is a windows GUI library that runs on top of Win32. Its main goal is to provide a rust-first API that is dead simple to use, keeps compile time low, does not bloat the final binary size, and provides a large number of features. As the first stable release, all those goals are met.
NWG also comes with a sister project, Native-Windows-Derive (also released as 1.0) that uses a procedural macro to build a GUI from a struct, removing almost all the boilerplate.
NWG is best suited for small to medium size projects that don't require fancy stuff (like animations or custom drawing).
javaI would like to share a new direction that my j4rs project took after its 0.12.0 release.
Until v0.12.0, j4rs provided to Rust applications the tools to achieve calls to the Java world. This included setting up and manage JVMs, instantiating Java Classes, making calls to Java methods, providing the means for Java to Rust callbacks etc.
The project was following solely a Rust-first approach, giving the Rust applications the ability to take advantage of the huge amount of libraries and tools existing in the Java ecosystem.
This Rust->Java only approach changed in v0.12.0. j4rs can now be used as well in Java projects that want to achieve JNI calls to Rust libraries.
Today is the day luminance-0.40 got released. This is a massive update. Like never before in the luminance ecosystem. A huge amount of features, bug fixes, usability / comfort enhancement, new crates and architecture redesign are gathered in that update. First, let’s talk about the new most important feature: the redesign.
Bastion is a distributed runtime which is inspired from the design principles of Erlang and adapts these into Rust ecosystem. Exactly 1 year passed since the initial release of Bastion. In this one year we’ve done so many amazing things together as Bastion team.
We are proud to announce our 0.4 release on our 1st birthday!
Tokio is an asynchronous runtime for the Rust programming language. It provides the building blocks needed for writing network applications. It gives the flexibility to target a wide range of systems, from large servers with dozens of cores to small embedded devices.
dbuszbus is a Rust crate for D-Bus. In short, zbus allows you to communicate from one program to another, using the D-Bus protocol. In other words, it’s an inter-process communication (IPC) solution. It is a very popular IPC solution on Linux and many Linux services (e.g systemd, NetworkManager) and desktop environments (e.g GNOME and KDE), rely on D-Bus for their IPC needs. There are many tools and implementations available, making it easy to interact with D-Bus programs from different languages and environments.
zbus is a 100% Rust-native implementation of the D-Bus protocol. It provides both an API to send and receive messages over a connection, as well as API to interact with peers through high-level concepts like method calls, signals and properties2. Thanks to the power of Rust macros, zbus is able to make interacting with D-Bus very easy.
asyncAsynchronous I/O in Rust is still very much in its infancy. Async/await, Rust’s solution to the problem, was recently stabilized and so when came the time to implement some peer-to-peer networking code, I reached for the shiny new feature. To my dismay, it created more problems than it solved. Indeed, I quickly regretted going down that path and searched for alternatives. All I was looking for was an easy way to handle between fifty and a hundred TCP connections (net::TcpStream) efficiently to implement the reactor for nakamoto, a Bitcoin client I’ve been working on.
http- 35 PRs merged
- Streamlined middleware error handling
- ResponseBuilder API
- State: Clone
- Extensible Server::listen (TLS support soon!)
Plotly.rs is a plotting library powered by Plotly.js. The aim is to bring over to Rust all the functionality that Python users have come to rely on; with the added benefit of type safety and speed.
audioA few months ago I wrote a new GStreamer plugin: an audio filter for live loudness normalization and automatic gain control.
Its code is based on Kyle Swanson’s great FFmpeg filter af_loudnorm, about which he wrote some more technical details on his blog a few years back. I’m not going to repeat all that here, if you’re interested in those details and further links please read Kyle’s blog post.
From a very high-level, the filter works by measuring the loudness of the input following the EBU R128 standard with a 3s lookahead, adjusts the gain to reach the target loudness and then applies a true peak limiter with 10ms to prevent any too high peaks to get passed through. Both the target loudness and the maximum peak can be configured via the loudness-target and max-true-peak properties, same as in the FFmpeg filter. Different to the FFmpeg filter I only implemented the “live” mode and not the two-pass mode that is implemented in FFmpeg, which first measures the loudness of the whole stream and then in a second pass adjusts it.
Below I’ll describe the usage of the filter in GStreamer a bit and also some information about the development process, and the porting of the C code to Rust.
gtkIf you use Gtk in Rust, you probably will need to write custom widgets. This document will show you how it is possible, and what tasks you need to go through.
At the time of writing this, gtk-rs 0.9.0 is being used. It is a set of Rust bindings for Gtk 3. Your mileage may vary on a later version of Gtk Rust. As an example, the original version of this document used 0.8 and only one section needed to be edited, and largely simplified.
We want to create MyAwesomeWidget to be a container, a subclass of GtkBox.
This is basically a direct followup to Amos/fasterthanlime's blog post Small strings in Rust. If you haven't read that, I'd highly suggest reading it first: it covers the techniques that I've stolen used here, which I won't go over in much detail.
Amos covers two Rust crates that offer the "small string" optimization: small strings (of less than around 22 bytes) can be stored inline rather than on the heap, like the standard String type. If you have a large number of small strings, this can greatly reduce allocation pressure.
Rather than small string optimization, though, for certain use cases an interner is useful. An interner associates a "symbol" with each unique string that you want to manage. You can then very cheaply copy the symbol around and compare symbols, as they're just a single integer (typically 32 bits). If you need the actual contents of the string again (such as for display), you just ask the interner to translate back from your symbol to a string.
I took a look at a few of the top interning crates for Rust (there are quite a few!) as ranked by lib.rs and compared them to see what their allocation behavior was. The testing harness is basically a direct copy of the one Amos used for small strings.
asyncSeveral months ago, on May 1st, I spoke to Stjepan Glavina about his (at the time) new crate, smol. Stjepan is, or ought to be, a pretty well-known figure in the Rust universe. He is one of the primary authors of the various crossbeam crates, which provide core parallel building blocks that are both efficient and very ergonomic to use. He was one of the initial designers for the async-std runtime. And so when I read stjepang’s blog post describing a new async runtime smol that he was toying with, I knew I wanted to learn more about it. After all, what could make stjepang say:
> It feels like this is finally it - it’s the big leap I was longing for the whole time! As a writer of async runtimes, I’m excited because this runtime may finally make my job obsolete and allow me to move onto whatever comes next.
If you’d like to find out, then read on!
This article is brought to you by a shameless nerd snipe, courtesy of Pascal.
In case you've blocked Twitter for your own good, this reads:
> There should be a post explaining and comparing smolstr and smartstring (and maybe others, like smallstr).
Well, I took the bait.
gtkHi everyone, time for a new release! Today, it’s all about improving APIs and providing the GdkX11 bindings.
linux asyncLast time I wrote about ringbahn, a safe API for using io-uring from Rust. I wrote that I would soon write a series of posts about the mechanism that makes ringbahn work. In the first post in that series, I want to look at the core state machine of ringbahn which makes it memory safe. The key types involved are the Ring and Completion types.
warp tide async httpSince I write a lot of articles about Rust, I tend to get a lot of questions about specific crates: "Amos, what do you think of oauth2-simd? Is it better than openid-sse4? I think the latter has a lot of boilerplate."
And most of the time, I'm not sure what to responds. There's a lot of crates out there. I could probably review one crate a day until I retire!
Well, I recently relaunched my website as a completely custom-made web server on top of tide. And a week later, mostly out of curiosity (but not exclusively), I ported it over to warp.
So these I can review. And let's do so now.
guiAn immediate mode GUI library written in Rust. Works anywhere you can draw textured triangles.
chardetng is a new small-binary-footprint character encoding detector for Firefox written in Rust. Its purpose is user retention by addressing an ancient—and for non-Latin scripts page-destroying—Web compat problem that Chrome already addressed. It puts an end to the situation that some pages showed up as unreadable garbage in Firefox but opening the page in Chrome remedied the situation more easily than fiddling with Firefox menus. The deployment of chardetng in Firefox 73 marks the first time in the history of Firefox (and in the entire Netscape lineage of browsers since the time support for non-ISO-8859-1 text was introduced) that the mapping of HTML bytes to the DOM is not a function of the browser user interface language. This is accomplished at a notably lower binary size increase than what would have resulted from adopting Chrome’s detector. Also, the result is more explainable and modifiable as well as more suitable for standardization, should there be interest, than Chrome’s detector
chardetng targets the long tail of unlabeled legacy pages in legacy encodings. Web developers should continue to use UTF-8 for newly-authored pages and to label HTML accordingly. This is not a new feature for Web developers to make use of!
Although chardetng first landed in Firefox 73, this write-up discusses chardetng as of Firefox 78.
pngThe release of png version 0.16.5 was one of the most personally exciting ones recently. It contains APNG support and large decoding improvements that will benefit everyone, both of which have been anticipated and planned for quite a while.
ffiWhen we started to build Ditto as a cross-platform SDK, we understood that it was untenable to create a specific port for each popular programming language. Instead, we opted to build the vast majority of shared code in Rust. Rust bought us a lot of features such as being easier to read, highly performant, and includes a modern build system and package manager. After the common code was built in Rust, we would then expose the primary APIs over a foreign function interface (FFI). For higher level programming languages like Swift, Java, and C#, we would create ergonomic bindings to Rust through calling the FFI C-headers.
While this FFI-strategy sounded straightforward on paper, in practice this didn't turn out so pretty. Our initial efforts primarily revolved around editing a singular C-FFI layer. We knew that C was the lingua franca of cross-language communication and initially assumed this singular layer to be the long term solution to writing FFI code. However, this FFI layer that we created began to grow unnaturally beyond it's initial purpose. As a rapidly iterating startup, non-trivial logic began to creep inside this FFI layer to compensate for the rising idiosyncracies and specific needs of each platform. As more logic began to creep into this FFI layer, we began to write more and more unit tests to guarantee stability of a layer that was only meant for passing functions and data around. As a side effect, we added more unit tests to the #[no_mangle] functions which frequently juggled several C-pointers.
svgI have just released the first version of lorikeet-dash and part of that exercise was to find out a way to draw SVG graphs using rust.
I thought my findings & approach may be useful for others looking to do the same thing and so I have documented the design evolution in this blog.
garbage-collectionI'm excited to announce shredder, a library for Rust that provides a garbage collected smart pointer, Gc. This smart pointer is useful in the same sorts of situations as Rc, but can handle reference cycles.
As a responsible Rust Evangelist, I try to propagate the language in my current job. So, once again, I’ve done a workshop, this time themed about async Rust. The goal was to write a small but non-trivial http service.
And while the exercise went mostly well and both Rust and hyper are perceived in a tentatively-positive way, there were two traps that tripped my colleagues. I’d like to share them here ‒ there might be something that can be done about them and even if not, it might save someone from the same traps.
nostd capnprotoOver the past few years, many people have expressed interest in using capnproto-rust in no_std environments – that is, without pulling in the Rust standard library. Today I’m happy to announce that the latest release, version 0.13.0, supports that.
mongodbI’m happy to announce that today we are releasing version 1.0.0 of the official Rust driver for MongoDB. This release marks the driver as generally available and ready for production usage. It supports MongoDB server versions 3.6 and later. In this blog post, I’ll cover:
* What’s changed in the driver since the initial alpha release
* How to get started with the driver.
* How you can get involved with the project
Welcome to the eleventh edition of This month in rustsim! This newsletter will provide you with a summary of important update that occurred within the rustsim community. This includes in particular updates about the nphysics (physics engine), salva (fluid simulation), ncollide (collision detection), nalgebra (linear algebra), and simba (abstract algebra) crates. This eleventh edition will contain updates for the months of April and May 2020.
linux asyncIn my previous post, I discussed the new io-uring interface for Linux, and how to create a safe API for using io-uring from Rust. In the time since that post, I have implemented a prototype of such an API. The crate is called ringbahn, and it is intended to enable users to perform IO on io-uring without any risk of memory unsafety.
async tokioIn the last blog of this series, I implemented job queue with tmq. I noted back then that tmq is great if you need to interact with other languages, but may be a little overkill if you are just using rust. I wondered what it'd take to build the job queue with a smaller library footprint, using something like tokio-serde instead of tmq. It was successful, and this blog will step through some of the changes needed.
With over 68,842 downloads, one of my most successful Rust projects is a nondescript little program called mdbook-linkcheck. This is a link-checker for mdbook, the tool powering a lot of documentation in the Rust community, including The Rust Programming Language and The Rustc Dev Book.
This tool has been around for a while and works quite well, so when I was fixing a bug the other day I decided it’s about time to extract the core logic into a standalone library that others can use.
Webmaster's job is to protect the website from malicious traffic. One of the practices serving this purpose is the collection and analysis of the website's HTTP access logs. I have developed Rust library and command-line tools to help discern malicious traffic from actual visitors.
windowsRust/WinRT follows in the tradition established by C++/WinRT of building language projections for the Windows Runtime using standard languages and compilers, providing a natural and idiomatic way for Rust developers to call Windows APIs. Rust/WinRT lets you call any WinRT API past, present, and future using code generated on the fly directly from the metadata describing the API and right into your Rust package where you can call them as if they were just another Rust module.
parsingI've recently been writing a bit of parsing code in Rust, and I've been jumping back and forth between a few different parsing libraries - they all have different advantages and disadvantages, so I wanted to write up some notes here to help folks who are undecided choose what libraries and techniques to consider, and also to offer some suggestions for the future of the Rust parsing ecosystem.
asyncI have just spent some time doing an initial async version of lorikeet now that the async/await syntax is stable and the ecosystem has caught up. The major blocker was reqwest, as this is used extensively in the http test. This async version is available now as version 0.11.0. You can also install the cli by running cargo install lorikeet.
Welcome to the tenth edition of This month in rustsim! This newsletter will provide you with a summary of important update that occurred within the rustsim community. This includes in particular updates about the nphysics (physics engine), salva (fluid simulation), ncollide (for collision-detection), nalgebra (for linear algebra), simba, and alga (for abstract algebra) crates. This tenth edition will contain updates for the month of March 2020.
I released wiremock, a new crate that provides HTTP mocking to test Rust applications. You can spin up as many mock HTTP servers as you need to mock all the 3rd party APIs that your application interacts with.
Mock HTTP servers are fully isolated: tests can be run in parallel, with no interference. Each server is shut down when it goes out of scope (e.g. end of test execution).wiremock provides out-of-the-box a set of matching strategies, but more can be defined to suit your testing needs via the Match trait.
wiremock is asynchronous: it is compatible (and tested) against both async_std and tokio as runtimes.
matrixToday, I have a bit of a sad announcement to make: Ruma, the homeserver, won't be developed any more.
To those who have actively been following the project, this probably won't be a big surprise, given the homeserver's readme file was already update do note the missing maintenance and people interested in contributing to the homeserver have now gathered around a new homeserver project by the name of Conduit.
The main reason for ending homeserver development is that nobody who has previously worked on its codebase is currently able to guide and review work on it. The codebase has also bit-rotted a lot, with the web framework it uses being almost un-maintained and a number of its other dependencies having seen major new releases since the most recent commit; it also doesn't build on ruma-client-api, for the simple reason that ruma-client-api simply didn't exist yet when most of the homeserver work happened.
However, the end of the homeserver does not at all mean the end of Ruma, the overall project.
New release for the Rust sysinfo crate! This time, the processes' disk usage was added.
There are a lot of reasons you might want to download all the crates ever uploaded to crates.io, Rust’s package registry: code analysis across the whole public ecosystem, hosting a mirror for your company, or countless other ideas and projects.
The team behind crates.io receives a lot of support request asking what’s the best and least impactful way to do this, so here is a little guide on how to do that!
grpcTonic: 0.2 release with the future in mind! After a few months of work we finally have released tonic 0.2. This release marks the start of a new path for tonic: Generic encoding.
The gtk-rs organization has been created in 2015. The original goal was to bring GTK+ library and other libraries from the GNOME platform to the Rust language. We now provide 19 bindings and that number will certainly increase even more in the future.
As this number continues to increase, our needs are increasing as well. At the same time, various people were asking in the past how they could support us but we could never give a useful answer to that. To solve this problem we decided to open a “collective” on opencollective.
asyncI’m now building the third async runtime and publishing it very soon. While async-std and tokio are conceptually very similar, the new runtime is outside their boxes and approaches problems from a completely new angle.
But don’t worry, this runtime is not intended to fragment the library ecosystem further - what’s interesting about it is that it doesn’t really need an ecosystem! I’m hoping it will have the opposite effect and bring libraries closely together rather than apart.
asyncTokio is a runtime for asynchronous Rust applications. It allows writing code using async & await syntax. The Rust compiler transforms this code into a state machine. The Tokio runtime executes these state machines, multiplexing many tasks on a handful of threads. Tokio’s scheduler requires that the generated task’s state machine yields control back to the scheduler in order to multiplex tasks. Each .await call is an opportunity to yield back to the scheduler. In the above example, listener.accept().await will return a socket if one is pending. If there are no pending sockets, control is yielded back to the scheduler.
This system works well in most cases. However, when a system comes under load, it is possible for an asynchronous resource to always be ready.
templatingliquid v0.20 resolves several planned breaking changes we've been holding off on. This doesn't make us ready for 1.0 yet but this closes the gap significantly.
liquid-rust is a rust re-implementation of the liquid template engine made popular by the jekyll static site generator.
guiAlchemy is a Rust GUI Framework, backed by native widgets on each platform it supports, with an API that's a blend of those found in AppKit, UIKit, and React Native. It supports a JSX-ish syntax (RSX), styling with CSS, the safety of building in Rust, and a familiar API for many developers who build UI on a daily basis. The goal is to provide an API that feels at home in Rust, while striving to provide a visual appearance that's easy to scan and parse. It does not, and will never, require nightly. It's still early stages, but feedback and contributions are welcome.
asyncLast weekend I released parallel-stream, a data parallelism library for async std. It's to streams, the way rayon is to iterators. This is an implementation of a design I wrote about earlier.
The way parallel-stream works is that instead of calling into_stream to create a sequential stream you can call into_par_stream to create a "parallel" stream instead. This means that each item in the stream will be operated on in a new task, which enables multi-core processing of items backed by a thread pool.
Call for help: I'm an undergraduate and while on a vacation, I could and did invest 12 ~ 14 hours to swc per day. But vacation is over and I can't afford to spend so much time. So I want some help from the community.
I welcome the contribution of any way, including developer time contribution or financial contribution.
Roadmap: I'll write in an order that I initially planned to work on. Also, I'll mention progress of the work and the hard parts.
I started writing integration tests for heim recently (better late than never) and the testing code started to bloat up really quick; it’s not very easy to check the logic correctness elegantly with what assertion macros we have in the Rust standard library, especially when we are working with the commonly used Result and Option types.
Once you have started making more abstractions, it is hard to stop, so I ended up with a separate crate called “claim", which provides a lot of assertion macros to supplement what we already have in libstd.
I have been regularly publishing crates over the last year. I only gradually discovered various ways of prepping a crate for release, and I have also found myself wanting all my crates to have similar conventions in a number of areas. When searching through the internets, I haven’t really come across a central resource with general tips and guidelines for publishing crates, so I decided to keep track of all the things I discovered piecemeal, as a reference/checklist for myself and others. If you are an experienced publisher, you probably won’t find many new ideas in here, but if you’re just getting started with Rust, be sure to check it out and save yourself time later.
asyncI’ve just released a new crate called waitmap. This is a concurrent hash map (built on top of dashmap) intended for use as a concurrency primitive with async/await. It extends the API of dashmap by having an additional wait method.
parsingThis is going to be interesting project for me.
I'm gonna build modern parsing library in Rust and I'll share here my experience.
Note - I'm not going to sell you golden solution - I have no final answers. Instead, I'd like to think about these articles as my notes and diary. Hopefully when I finish both you and I will learn something new.
Ok. Let's start from the beginning.
testingKaos is a chaotic testing harness to test your services against random failures. It allows you to add points to your code to crash sporadically and harness asserts availability and fault tolerance of your services by seeking minimum time between failures, fail points, and randomized runs.
Kaos is equivalent of Chaos Monkey for the Rust ecosystem. But it is more smart to find the closest MTBF based on previous runs. This is dependable system practice. For more information please visit Chaos engineering.
Starting from the fixes, with the release of 0.8 was introduced the multi-thread support on indexes, this did actually had a few issue, bringing in the need of 3 hotfixes, that did not completely fix the problem, at the end was needed some disc information change for completely fix the concurrency problem so a new minor release was needed, from my tests this new release fixes all the issue related to concurrency on indexes, so from 0.9 is safe do do concurrent put and remove, in 0.8.x I would suggest not to have two concurrent prepare_commit with changes on the same index.
Performance as well where improved from my tests even though more can be optimized, and all the basic performance should consider that prepare_commit before return the control will make sure that the fsync happened, so the number of transactions that can be done single thread are strictly dependent to the latency of the disc, if you want some numbers feel free to write down your own micro-benchmark, there is nothing better then your code on your machine to verify the performances you need.
Nannou is an open source, creative coding framework for Rust. Today marks one of the biggest milestones for the project since its launch - the release of version 0.13.
This version is particularly special for our community as it opens the floodgates for a lot of pending graphics work and paves the road ahead for running nannou on the web!
heim is a cross-platform Rust crate for retrieving information about system processes and various system details (such as CPU, memory, disks, networks and sensors).
heim has few key goals which define its development and public interface:
* Async-first.
* Cross-platform.
* Any code from heim should just work on all supported platforms. OS-specific things do exist, but API design forces users to pay attention to them.
* Modular design.
* Idiomatic and easy to use.
For those who has never heared about rspotify before, rspotify is a Spotify web Api wrapper implemented in Rust. Today, I am exited to introduce you the v0.9 release I have been continued to work on it for the past few weeks that adds async/await support now!
In the last section, we covered how to get plotting with Ploty using Plotly for Rust paired with our very own workaround. If you continued experimenting with this approach before starting this section you may have encountered some limitations: file size, multiple plots, file size, again.
We're going to improve our workaround so that we can produce many of our nice interactive plots without bloating our notebooks and any HTML files we may save to.
async httpToday Friedel Ziegelmayer (Protocol Labs), Ryan Levick (Microsoft), and myself would like to introduce a new set of HTTP libraries to make writing encrypted, async http/1.1 servers and clients easy and quick:
* async-h1 – A streaming HTTP/1.1 client and server protocol implementation.
* http-types – Reusable http types extracted from the HTTP server and client frameworks: Tide and Surf.
* async-native-tls – A streaming TLS client and server implementation.With these libraries writing a streaming, encrypted HTTP client takes about 15 lines of code.
I'm announcing a "sneak preview" (v0.0.1) release of Veriform: a binary serialization format inspired by Protocol Buffers, but with a number of refinements which make it more useful to things like credentials, signed documents, and "blockchain" use cases.
I was hoping to get it in a slightly more polished state before making an announcement, but after seeing the mincodec announcement yesterday (which contains a number of similar ideas, but also many differences), I thought I'd go ahead and make an announcement, both since it seems there's a lot of interest in binary encodings which support no_std environments, and also because it seems like there's some potential collaboration opportunities between mincodec and Veriform.
Tremor is an early stage event processing system for unstructured data with rich support for structural pattern matching, filtering and transformation.
Graphs pervade science and technology. As such, many kinds of software projects rely on a graph library. This article introduces GraphCore, a new graph library written in Rust.
gtkThe vgtk project started out as a side effect of one of my "must write a text editor" phases, as many things do. It triggers a review of the state of UI development in my current favourite language, and sometimes it triggers a ground-up attempt to construct an ad-hoc, informally-specified, bug-ridden, slow implementation of half of Elm, when I decide the state of the art is insufficient for my tastes. Usually, if the ground is sparsely trodden, I never get further than building some of the developer tooling necessary to build the UI tooling I need to build my text editor.
In this latest case, thanks to the superlative state of Rust's developer tooling—and its low level bindings for at least one sufficiently qualified UI toolkit—I've gotten to the point where I've been able to build UI tooling that appeals to my idea of what UI tooling should look and feel like. So far, I've yet to build the text editor, but I've come to accept by now that it will only ever exist as a Platonic ideal, serving only as a motivating force to get me started building more useful things.
I'll try to introduce vgtk, idea by idea, by way of a tutorial. You'll need to have a working knowledge of Rust to follow along. You shouldn't need to know GTK already, but you may need to be prepared to consult GTK documentation to understand certain things fully.
testingfaux is a traitless Rust mocking framework for creating mock objects out of user-defined structs. faux uses attributes to transform your structs into mockable versions of themselves at compile time.
After quite some effort I'm finally ready to release first version of jlrs, the Julia bindings I've been working on for the past few weeks.
This first version brings many nice features. For example:
- Primitive data and strings can be copied from Rust to Julia.
- N-dimensional arrays containing primitive data can be created; their contents can either be completely managed by Julia, take ownership of data from Rust, or mutably borrow data from Rust.
- All of these can be copied back from Julia to Rust.
- You can acquire handles to arbitrary modules, globals and functions, and call the latter.
- You can include and call your own Julia code.
- If a Julia function returns another function, you can call it.
- You can only use Julia data that is guaranteed to be protected from garbage collection.
sysinfo is crate to get system information, such as processes, processors, disks, components and networks. Now let's go! :)
Time for a new sysinfo release! In this version, multiple things were added, such as the load average and processor information (frequency and vendor ID). The API has also been reworked to give you more control. Let's check it in details!
markdownA couple days ago I released markedit, a small crate for editing unstructured markdown documents. This is a useful enough library that I thought I’d explain the main ideas behind it and potential use cases.
This originally came about when I was at work, preparing our application’s change log before a release for the umpteenth time (we’ve found the keep a changelog format really useful) and on the drive home I started thinking of ways to automate things.
While the 0.22 release series received a few bug fixes and feature releases, the next major version was being worked on in the background, and comes with improvements to error handling, and the buffer and loading interfaces. A more detailed view on the changes is in the following paragraphs.
aws asyncRusoto, an AWS SDK for Rust, is now compatible with std::future::Future in a beta release, v0.43.0-beta.1.
This comes shortly after merging Rusoto’s async/.await pull request, which is likely the biggest change to Rusoto since shattering the mega-crate.
If you are using Rusoto today, please help us test this release, file issues if it doesn’t work, and tell us in Discord or email me if it does.
Three separate contributors brought Rusoto forward to modern Rust, and several others made additional contributions to improve the ergonomics of using the SDK. Thanks so much to everybody working on Rusoto.
redisRedis is a fantastic noSQL database with a beautifully simple design. One of the fundamental responsibilities of the redis server is to encode and decode RESP (Redis Serialization Protocol) messages. For example, when a client issues the command:
SET foo bar
That's encoded by the client and sent to the server as:
*3\r\n$3\r\nSET\r\n$3\r\nfoo$3\r\nbar\r\n
It's not necessary important to understand that RESP message right now, but the server will need to decode that back into something equivalent so it can perform the operation. This blog post will go through my efforts to implement a copyless RESP parser in redis-oxide.
asyncFirstly, congratulation on Rust lang achieving stable async/await syntax! As of the release, async/await is becoming the preferred way to do asynchronous programming instead of using Futures in Rust lang.
In Obsidian Web Framework, we do the same move just like other libraries which enabling async/await syntax in order to provide a better development experience.
templatingHave you ever wanted a string templating engine in Rust that has basically no features? Well, your dreams have now come true! Let me introduce you to Find And Replace (FAR): a string template engine with basically no features. I'll start with a feature comparison with a more popular templating engine.
Basian is a highly-available distributed fault-tolerant runtime
So we had a long way since our project had started in June 2019. Right now, we are evolving from a runtime that enables fault-recovery for your server-side applications to an agnostic runtime, which has its executor but also works with other executors. Since the beginning we can interoperate with async-std without the hassle.
clap is a library which provides the ability to parse command line options. For SortaSecret.com, we have relatively simple parsing needs: two subcommands and some options. Some of the options are, well, optional, while otherwise are required. And one of the subcommands must be selected. We'll demonstrate how to parse that with clap.
testingMock objects are test versions of objects that contain fake implementations of their behavior. For example, if your code accesses your file system, makes network requests, or performs other expensive actions, it is often useful to mock that behavior. Mocking can help make your tests into true unit tests that run quickly and produce the same result every time without relying on external dependencies. For a deeper dive into mocks, read this post.
Last week I wrote about how capnproto-rust might relax its memory alignment requirements and what the performance cost of that might look like. The ensuing discussion taught me that memory alignment issues can be thornier than I had thought, and it strengthened my belief that capnproto-rust users ought be shielded from such issues. Since then, working with the helpful feedback of many people, I have implemented what I consider to be a satisfactory resolution to the problem. Today I’m releasing it as part of capnproto-rust version 0.12. The new version not only provides a safe interface for unaligned memory, but also maintains high performance for aligned memory.
guiAs I’ve worked on my Pushrod library over the past year, one of the major struggles I encountered was trying to figure out how to draw using just the GPU, instead of drawing directly to the on-screen Canvas.
I originally started this project using Piston (thus, the engine analogies), and found that library to be overly complicated for what I wanted. Although it provided all of the features I wanted, it introduced a lot of overhead that was concerning. Not to mention, Piston is a more game-oriented library.
I ultimately settled on SDL2.
Optimath is an experimental const generics based linear algebra library that works without any allocations in no_std and utilizes simd. So now you can do fancy maths on embedded.
Warp is a Rust web server framework focusing on composability and strongly-typed APIs. Today sees the release of v0.2! The most exciting part of this release is the upgrade to std::future, so you can now use async/await for cleaner flow control. Due to how warp encourages composition of filters, this is most noticeable at the “ends” of a filter chain, where an application is doing its “business logic”, converting input into actions and replies. And that’s where most of the app code is! Services In the original release of warp, I wrote: We’d like for warp to be able to make use of all the great tower middleware that already exists. As part of this release, that is now possible! Any Filter which returns a Reply can now be converted into a Service using warp::service(filter).
fuzzingToday, on behalf of the Rust Fuzzing Authority, I’d like to announce new releases of the arbitrary, libfuzzer-sys, and cargo fuzz crates. Collectively, these releases better support writing fuzz targets that take well-formed instances of custom input types. This enables us to combine powerful, coverage-guided fuzzers with smart test case generation.
grpcTonic: 0.1 has arrived! tonic is a gRPC over HTTP/2 implementation focused on high performance, interoperability, and flexibility. It has been a few months since I originally released the 0.1.0-alpha.1 version. Since then there has been a ton of growth and improvements. We’ve seen 32 new contributors, two new guides, 8 more examples and 5 releases. Not only have we seen a lot of growth internally but we’ve also seen a large amount of adoption.
databaseMany years ago I wrote a commercial product that could import a database schema and then generate source code based on the schema. There were many different use cases for this product and it could be used to generate simple Data Access Object (DAO) code or even to generate fully working (although very crude) web applications for data entry. Believe it or not, some companies have schemas with more than 500 tables, so tools like this can dramatically reduce development costs. This type of product isn’t very sexy or modern but it generated decent revenue for a side project at the time, and there are still valid use cases today for this type of tool.
When I started prototyping a generic database tool in Rust a few weeks ago, I discovered that there isn’t a standard way to access databases in Rust and that I would have to write separate code for every single database, and I’m not just talking about MySQL and Postgres (two wouldn’t be so bad), I’m also thinking about distributed query engines such as PrestoDB, Hive, Impala, and Drill, to name just a few. Rather than writing custom integrations for my prototype, I switched gears and started prototyping a Rust version of ODBC/JDBC instead, which I unimaginatively named Rust Database Connectivity, or RDBC for short.
cplusplus ffiThis library provides a safe mechanism for calling C++ code from Rust and Rust code from C++, not subject to the many ways that things can go wrong when using bindgen or cbindgen to generate unsafe C-style bindings.
This doesn't change the fact that 100% of C++ code is unsafe. When auditing a project, you would be on the hook for auditing all the unsafe Rust code and all the C++ code. The core safety claim under this new model is that auditing just the C++ side would be sufficient to catch all problems, i.e. the Rust side can be 100% safe.
elasticsearchThe Rust client is largely generated from the REST API specs of Elasticsearch. As such, the API functions available align with the version of the specs from which they're generated. Elasticsearch strives to adhere to Semantic Versioning, which is reflected in the specs and thus this client.
What this means in practice is that a 7.x Rust client is compatible with Elasticsearch 7.x. Where the client minor version is greater than Elasticsearch minor version, it may contain API functions for APIs that are not available in the version of Elasticsearch, but all other API functions will be compatible. Where the client minor version is less than Elasticsearch minor version, all API functions will be compatible.
The project is still very much a work in progress and in an alpha state; input and contributions welcome!
Hi everyone! Today, sysinfo has received a new update with nice performance improvements on OSX. As a reminder, sysinfo is crate used to get systems' information (Linux, Windows, OSX, Android and raspberry pi are supported).
swc use proc macros extensively. I use proc macro if possible to reduce boilerplates and to avoid rewriting boilerplates when something is changed. Behind the scene, there's a hero crate called pmutil. It provides useful stuffs for proc macros.
async tokioI would like to understand how Tokio works. My interests run to the real-time and concurrent side of things but I don't know much about Tokio itself. Before the introduction of async and stable futures I more or less intentionally avoided learning it, not out of any sense that Tokio was wrong but there's only a finite amount of time to learn stuff and it's a rough business to learn a thing that is going to go out of date soonish.
Anyhow. These are my notes for learning Tokio. I don't have a plan of how to learn it's internals, but, generally, I learn best when I have some kind of project to frame my reading around. Context really helps. I don't have a sense of what I want to build long-term, but an HTTP load generator that can scale itself to find the maximum requests per second a server can handle while still satisfying some latency constraint would be pretty neat. This does mean I need to combine my learning with another library – hyper -- but I've used it before and think I can get away with leaving it as a black-box.
async httpreqwest is a higher-level HTTP client for Rust. Let me introduce you the v0.10 release that adds async/await support!
physicsSalva is a 2 and 3-dimensional particle-based fluid simulation engine for games and animations. It uses nalgebra for vector/matrix math and can optionally interface with nphysics for two-way coupling with rigid bodies, multibodies, and deformable bodies. 2D and 3D implementations both share (mostly) the same code!
I'm Ryan, maintainer of Quicksilver 67 and a relatively new maintainer of Winit, a pure-Rust library for creating and managing windows. We're used by a wide range of desktop and gaming software, from Alacritty and Servo to ggez and Amethest. With an alpha release of Winit's next version (0.20.0-alpha4), we've brought Winit to a new platform: the web!
graphics-2dAbout a year ago, in the lyon in 2018 post on this blog, I mentioned that I was working on a complete rewrite of lyon's central piece, the fill tessellator. I have been working on this for quite a bit. The work-in-progress pull request was created in February 2018. Almost two years in the making, this work made it in version 0.15, the project's biggest release ever.
It was a lot of work. Too much work. Fortunately, I am pretty happy about the result.
videoThe second official release of rav1e was focused mainly on speed. Later I'll write down what we used and what, in my opinion, are the best practices when you have to optimize a codebase like this one.
This crate is my attempt to be the easiest way to create a hardware-accelerated pixel frame buffer. The stated goals and comparison with similar crates can be found in the readme. Below is a discussion of use cases where I imagine pixels will fill an existing need.
TL;DR: It puts pixels on your screen.
Today we have released a library that you can use to implement license key verification for applications written in Rust. The library is freely available on GitHub.
Crossterm 0.14 is released. This release is centered toward an advanced event reading layer. During the rewrite, strict attention has been paid to adaptability, performance, and ease of use. The design has been tuned with several projects (cursive, broot, termimad) in order to obtain a well-designed API.
parsing tutorial nomNom's official documentation includes trivially simple examples (e.g. how to parse a hexadecimal RGB color code) and very complicated examples (e.g. how to parse json). When I first learned nom I found a steep learning curve in between the simple and complex examples. Furthermore, previous versions of nom, and most of the existing documentation, use macros. From nom 5.0 onward macros are soft-deprecated in favor of functions. This tutorial aims to fill the gap between simple and complex parsers by parsing the contents of /proc/mounts, and it demonstrates the use of functions instead of macros.
petgraph is a Rust library which allows you to work with graph data structures. This is the second part on a series about working with graphs in Rust. It covers the internal representation of graphs by petgraph.
dnsA review of preparing Trust-DNS for async/await in Rust
What started as a brief sojourn to learn the new std::future::Future in Rust 1.36, slowly became a journey to fully adopt the new async/await syntax in Rust. The plan had been to merely update to the new Future API, trying to keep the minimum Rust version as low as possible. This was ideally to keep the libraries compatible with more Rust users, but it became aparent that this wasn’t really feasible. For a number of reasons, primarily, all of the underlying libraries Trust-DNS relies upon were moving in this direction, which made the task a fools errand. Additionally, adopting async/await simplified much of the code. This post is the announcement of the 0.18 release, representing a few months of work.
I’m delighted to announce that today we are releasing our first alpha build of the new official Rust driver for MongoDB. In this blog post, I’ll cover: The background on Rust at MongoDB and why we have built a new driver. Show you how to get started with the driver. Share how you can get involved with the project.
async tokioThe release of Tokio 0.2 was the culmination of a great deal of hard work from numerous contributors, and has brought several significant improvements to Tokio. Using std::future and async/await makes writing async code using Tokio much more ergonomic, and a new scheduler implementation makes Tokio 0.2’s thread pool as much as 10x faster. However, updating existing Tokio 0.1 projects to use 0.2 and std::future poses some new challenges. Therefore, we’re very excited to announce the release of the tokio-compat crate to help ease this transition, by providing a runtime compatible with both Tokio 0.1 and Tokio 0.2 futures.
asyncMio 0.7 is the work of various contributors over the course of roughly half a year. Compared to Mio version 0.6, version 0.7 reduces the size of the provided API in an attempt to simplify the implementation and usage. The API version 0.7 will be close to the proposed API for a future version 1.0. The scope of the crate was reduced to providing a cross-platform event notification mechanism and commonly used types such as cross-thread poll waking and non-blocking networking I/O primitives.
Today, we’re introducing the new async-std runtime. It features a lot of improvements, but the main news is that it eliminates a major source of bugs and performance issues in concurrent programs: accidental blocking.
Hello everyone, time for a new release!
This time, this is mostly about stabilization and simplification. It means that gtk-rs is now simpler to use and more complete than ever. Let’s take a tour of what’s new(er)!
This is the 41th iteration on what happened in the last four weeks in the imag project, the text based personal information management suite for the commandline.
Almost half a year since the last update. I could definitively do better, I know. But a lot of stuff has happened.
After a few months of alpha development, the final release of hyper v0.13.0 is now ready!
micromath is a fast, embedded-friendly approximation-based math library with support for basic arithmetic, trig functions, 2D/3D vector types, statistical analysis, and quaternions. Optimizes for speed and code size at the cost of precision.
Hi everyone! Today, sysinfo has received a new update with nice improvements. As a reminder, sysinfo is crate used to get systems' information (Linux, Windows, OSX and raspberry pi are supported).
Tera is a template engine inspired by Jinja2 and the Django template language. It is also similar to Twig and Liquid if you are coming from PHP/Ruby.
In most cases, the upgrade to v1 should be painless: unless you are using the error-chain part of Tera errors it might even work without any changes.
Before going further, a huge thank you to everyone that contributed. Let's look at some of main changes in a bit more details now.
async tokio tutorialIn the previous lesson in the crash course, we covered the new async/.await syntax stabilized in Rust 1.39, and the Future trait which lives underneath it. This information greatly supercedes the now-defunct lesson 7 from last year, which covered the older Future approach.
Now it’s time to update the second half of lesson 7, and teach the hot-off-the-presses Tokio 0.2 release.
Our Rust driver is a fork of the unsupported Rust driver prototype developed by MongoDB labs. The irony of this prototype project is that while it is not officially supported, it remains one of the best Rust drivers available. In other words, if you need MongoDB support in Rust, chances are you’d be using it, which is exactly what we did.
It worked fine initially, and we thought it would get improved over time, except it didn’t. Even worse, when inquiring about the original Rust driver project status, we learned that it would not be maintained because all efforts are being put on a new official Rust driver.
A couple years ago, we released the beginning of the http crate. It’s purpose was to allow a common API for the ecosystem to interact with HTTP types, without those types referring to a specific implementation. We’ve seen great things sprout up since then!
moxie is a small incremental computing runtime focused on efficient declarative UI, written in
Rust. moxie itself aims to be platform-agnostic, offering tools to higher-level crates that work on
specific platforms. Most applications using moxie will do so through bindings between the runtime
and a concrete UI system like the web or a consumer desktop platform.This post has been forming for a while, and I find myself finally getting to it thanks to Raph's
thought-provoking post on reactive UI. I'll discuss a bit of how I view moxie's approach to declarative UI in Rust and how the project fits into the mental model Raph explores in his post.
Lately I was porting a software from tokio/futures-1.0 to async-await. I somehow thought async-std was the successor of tokio and ported everything to async-std. 80% in, I noticed that my hyper dependency requires tokio and that it's not possible to replace tokio with async-std without also replacing hyper. Also, tokio and async-std try to solve the same problem. So I started a journey into the inners of the rust async story to find out if it is possible to use both tokio and async-std at the same time. (tl;dr: it is). I had heard of reactors and executors before, but there was much new stuff to discover.
I found it interesting, so I decided to write it down. The following is a dive into a mixture of async-std-1.0.1 and async-std-master. I guess that tokio does not differ that much in its architectural design. I will skip async-await sugar, Pin and futures. Disclaimer: There may be misconceptions in here.
This blog post describes the building of a streaming HTML rewriter/parser with a CSS-selector based API in Rust. It is used as the back-end for the Cloudflare Workers HTMLRewriter. We have open-sourced the library (LOL HTML) as it can also be used as a stand-alone HTML rewriting/parsing library.
tideToday we're happy to announce the release of Tide 0.4.0 which has an exciting new design. This post will (briefly) cover some of the exciting developments around Tide, and where we're heading.
This is a brief account of how I fulfilled a small feature request a user made against the headless_chrome crate. There’s nothing particularly fancy or interesting about the change, but I thought a write-up might make it easier for others to contribute in future. It could be also be interesting for anyone curious about how Puppeteer and Chrome DevTools work under the hood.
With people reflecting on Rust in 2019 and what they want to see in 2020, error handling has come up again:
Rust in 2020, one more thing
Thoughts on Error Handling in Rust
Error Handling SurveyIt felt like there was interest in moving anyhow into the stdlib. While I feel that a lot of it is ready or near-ready (see below), I feel we have gotten stuck in a rut with the context pattern. I've been told that the pattern is derived from cargo and it feels like we've mostly been iterating on that same pattern rather than exploring alternatives. After framing my concerns with error handling and where we are at with existing solutions, I'll introduce Status as a radical alternative (from the Rust ecosystem perspective) for addressing these problems.
For the past year or more, I've been passively watching the progress of the "async/await" implementation in the Rust programming language. Though I can't speak to the technical details in the Rust compiler, it's been neat to learn new concepts (to me) like Pin, waker, and Future; these aren't things I deal with often as a higher-level developer.
We are very excited to announce Tokio 0.2. This is a ground up rework of Tokio based on async / await and experience gained over the past three years. Add the following to Cargo.toml: tokio = { version = "0.2", features = ["full"] } This is a major update, and as such there are changes to almost all parts of the library.
The Cryptowatch team has recently welcomed Héctor Ramón, also known as hecrj, who is the author of a Rust GUI framework called iced. Héctor has joined us as our first full-time Rust developer. Héctor got a lot of attention for iced when he announced it on reddit in September, and deservedly so. It’s a nicely designed framework inspired by Elm; it provides the necessary abstractions to build a GUI while leaving an open interface for any developer to provide their own component and rendering logic. Since joining our team Héctor has been hard at work continuing to develop iced and prove it out as a cross-platform GUI framework for Rust.
It seems one of the duties maintainers have is making sure their libraries are known. A library nobody uses just dies of loneliness. One of the way is to write blog posts about the libraries, so here we go. This one is about Signal Hook and its new support for cleaning up the signals.
A walkthrough of the recent bitfield behavior I implemented in `bitvec`.
Rust's error handling is a pleasure to use thanks to the Result type. It ensures Rust's error handling is always correct, visible, and performant. And with the addition of the ? operator in Rust 1.13, and the addition of return types from main in Rust 1.26 Rust's error handling has only kept improving.
However it seems we haven't quite reached the end of the road yet. The Crates.io ecosystem has seen a recent addition of a fair number of error-oriented libraries that try and improve upon the status quo of Rust's error handling.
This post is a survey of the current crates.io error library landscape.
fontsToday YesLogic is open-sourcing the Allsorts font parser, shaping engine, and subsetter for OpenType, WOFF, and WOFF2 under the Apache 2.0 license. Allsorts was extracted from the Prince HTML to PDF typesetting and layout tool and is implemented in Rust.
svgCSS length values have a number and a unit, e.g. 5cm or 6px. Sometimes the unit is a percentage, like 50%, and SVG says that lengths with percentage units should be resolved with respect to a certain rectangle. The process of converting that kind of units into absolute pixels for the final drawing is called normalization. In SVG, percentage units sometimes need to be normalized with respect to the current viewport (a local coordinate system), or with respect to the size of another object (e.g. when a clipping path is used to cut the current shape in half). One detail about normalization is that it can be with respect to the horizontal dimension of the current viewport, the vertical dimension, or both.
Serde is one of the most popular Rust crates, and deservedly so. If you aren’t familiar, Serde describes itself as “a framework for serializing and deserializing Rust data structures efficiently and generically.” What is most impressive to me is how robust the Serde data model has proven to be, allowing it to support human readable protocols like JSON and YAML, but also binary formats like Bincode. Its really a bonus that Serde does this while remaining exceptionally performant.
This blog posts dives into how Serde (along with the ecosystem of Serde data formats) is able to pull this off. To limit the scope of this post I am going to focus on Serde serialization to JSON, and skip any discussion of deserialization. If you are interested in deserialization (or a different data format) I believe you will be able to perform a similar analysis yourself after reading this post.
Well, here it is. The (by some, at least) long awaited Palette 0.5.0 release. This one has been brewing for quite some time while waiting for the ecosystem to really support one of its main additions1. That addition is #[no_std] support.
First, what is Palette? It’s a Rust library for working with colors and color spaces. It uses the type system to prevent mistakes, like mixing incompatible colors or working with non-linear RGB. It encodes the color spaces and their meta data (such as RGB primaries and white point) into the types to help making color processing less error prone and hopefully more accessible to those who don’t want to dive into the rabbit hole that is colors in computing.
Bastion is a highly-available, fault-tolerant runtime system with dynamic dispatch oriented lightweight process model.
gui gtkWhen talking to various people at conferences in the last year or at conferences, a recurring topic was that they believed that the GTK Rust bindings are not ready for use yet.
I don’t know where that perception comes from but if it was true, there wouldn’t have been applications like Fractal, Podcasts or Shortwave using GTK from Rust, or I wouldn’t be able to do a workshop about desktop application development in Rust with GTK and GStreamer at the Linux Application Summit in Barcelona this Friday (code can be found here already) or earlier this year at GUADEC.
After an epic amount of refactoring, librsvg now does all CSS parsing and matching in Rust, without using libcroco. In addition, the CSS engine comes from Mozilla Servo, so it should be able to handle much more complex CSS than librsvg ever could before. This is the story of CSS support in librsvg.
asyncasync-std is a port of Rust’s standard library to the async world. It comes with a fast runtime and is a pleasure to use. We’re happy to finally announce async-std 1.0. As promised in our first announcement blog post, the stable release coincides with the release of Rust 1.39, the release adding async/.await. We would like to thank the active community around async-std for helping get the release through the door.
linuxToday I’m releasing a library called iou. This library provides idiomatic Rust bindings to the C library called liburing, which itself is a higher interface for interacting with the io_uring Linux kernel interface. Here are the answers to some questions I expect that may provoke.
What is io_uring? io_uring is an interface added to the Linux kernel in version 5.1. Concurrent with that, the primary maintainer of that interface has also been publishing a library for interacting with it called liburing.
Welcome to the eighth edition of This month in rustsim! This monthly newsletter will provide you with a summary of important update that occurred within the rustsim community. This includes in particular updates about the nphysics (physics engine), salva (fluid simulation), ncollide (for collision-detection), nalgebra (for linear algebra), and alga (for abstract algebra) crates. This eighth edition will actually contain updates for the past three months (I got sick between the second and third month so I did not get the time to write a new post then. Sorry!)
When I announced momo, I wanted its users to save both binary size and compile time while keeping their code simple. I succeeded in the first goal, but failed regarding the second. The reason was that momo requires syn and quote which (unless you have other proc macros in your dependencies) add their own compile time to yours.
This repository contains implementations, unit tests, and benchmark code for the "2-level rotated array" structure, first published in Munro and Suwanda's 1979 paper "Implicit Data Structures for Fast Search and Update" (which also introduced the much better-known beap data structure). This structure is further developed and discussed in "Implicit Data Structures for the Dictionary Problem" (1983) and "Succinct Dynamic Data Structures" (2001). (The latter generalizes the idea to the dynamic array abstract data type, rather than a sorted array.)
The theoretical advantage of a 2-level rotated array over an ordinary sorted array is that it provides the same search performance (O(log n)), with much better insert and delete performance (O(√n), compared to O(n) for a sorted array), in exactly the same amount of space (i.e., no more than the data itself).
The overflower crate contains a helper library and procedural attribute macro to allow specifying how overflow should be handled. Since its inception, it has relied on specialization to change just the integer arithmetics while leaving the other operations basically unchanged.
A Rust library for compiling and matching regular expressions. It uses a hybrid regex implementation designed to support a relatively rich set of features. In particular, it uses backtracking to implement "fancy" features such as look-around and backtracking, which are not supported in purely NFA-based implementations (exemplified by RE2, and implemented in Rust in the regex crate).
This post can serve as a step by step migration guide from spirit 0.3 to spirit 0.4. If you already have an application using the crate, read on.
If you haven’t heard about the spirit library yet, it is a library to help you manage your configuration in an application and have it reloaded at runtime. It allows you to have the changes applied automatically and also to manage lifetime of the application. You can read more about it here. In that case, a migration guide won’t help you much, but I’m planning on having a tutorial how to start with the library soon.
On Wednesday night, I released version 0.16.0 of Rookeries, my developer/designer friendly static site generator! What makes this release exciting is that I added cross-platform support for macOS and FreeBSD! Also I setup an cross-platform installer, that works on all 64-bit x86 Linux distros including Windows Subsystem for Linux (WSL) 2.0. And I plan on supporting Linux, macOS (OS X) and FreeBSD as first-class systems going forward.
By compiling macros ahead-of-time to Wasm, we save all downstream users of the macro from having to compile the macro logic or its dependencies themselves. Instead, what they compile is a small self-contained Wasm runtime (~3 seconds, shared by all macros) and a tiny proc macro shim for each macro crate to hand off Wasm bytecode into the Watt runtime (~0.3 seconds per proc-macro crate you depend on). This is much less than the 20+ seconds it can take to compile complex procedural macros and their dependencies.
async tokioWe’ve been hard at work on the next major revision of Tokio, Rust’s asynchronous runtime. Today, a complete rewrite of the scheduler has been submitted as a pull request. The result is huge performance and latency improvements. Some benchmarks saw a 10x speed up! It is always unclear how much these kinds of improvements impact “full stack” use cases, so we’ve also tested how these scheduler improvements impacted use cases like Hyper and Tonic (spoiler: it’s really good).
databaseIn the last couple of months just right after the release of Persy 0.6 the development speeded up a bit, a few people started to play with persy and some downstream projects as well, first reporting few critical issue that produced the 3 hotfix 0.6.1,0.6.2,0.6.3 and then starting contributing back.
httpreqwest alpha.await reqwest is a higher-level HTTP client for Rust. I’m delighted to announce the first alpha release that brings async/await support!
Riker is a framework for building modern, concurrent and resilient systems using the Rust language. Riker aims to make working with state and behavior in concurrent systems as easy and scalable as possible. The Actor Model has been chosen to realize this because of the familiar and inherent simplicity it provides while also providing strong guarantees that are easy to reason about. The Actor Model also provides a firm foundation for resilient systems through the use of the actor hierarchy and actor supervision.
With mda-rs I wanted to create an experience as close as possible to using an interpreted domain specific language, the approach follow by typical MDAs, while still having the performance and power of a full, compiled language at the fingertips. One aspect of this experience was providing an API that feels like natural fit for the intended purpose. The other aspect was providing a straightforward way to build a custom MDA. For this second aspect, the simplicity of Rust's cargo was one of the reasons I decided to use Rust for this project.
Bastion is a fault-tolerant runtime for Rust applications. After receiving plenty of good feedback from the community and a long-running development stage, now Bastion is 0.2.0!
This archiver does deduplication, encryption, compression, data verification, and (ideally) supports backing up to untrusted storage, including cloud storage (though currently it only supports a local storage backend).
static_assertions is a library designed to enable users to perform various checks at compile-time. It allows for finding errors quickly and early when it comes to ensuring certain features or aspects of a codebase. The macros it provides are especially important when exposing a public API that requires types to be the same size or implement certain traits.
Introducing Tauri-Apps, an open-source project to help you make native apps with any framework with the power of Rust.
If you need to validate complex data structures at runtime in the programming language Rust then our semval library may empower you to enrich your domain model with semantic validation.
It’s been a month and a half since the heim public announcement, so it’s about time to sum up the work done and make a roadmap for next development iteration. As a quick reminder: heim is the Rust cross-platform async library for system information fetching — CPU, memory, disks, networks, you name it.
An implementation of the Raft distributed consensus protocol using the Actix actor framework. Blazing fast Rust, a modern consensus protocol, an outstanding actor framework. This project intends to provide a backbone for the next generation of distributed data storage systems (SQL, NoSQL, KV, Streaming &c) built with Rust.
I’m pleased to announce the release of Criterion.rs v0.3, available today. Version 0.3 provides a number of new features including preliminary support for plugging in custom measurements (eg. hardware timers or POSIX CPU time), hooks to start/stop profilers, a new BenchmarkGroup struct that provides more flexibility than the older Benchmark and ParameterizedBenchmark structs, and an implementation of a #[criterion] custom-test-framework macro for those on Nightly.
rubyArtichoke is a platform for building MRI-compatible Ruby implementations. Artichoke provides a Ruby runtime implemented in Rust that can be loaded into many VM backends.
asyncWe are excited to announce a beta release of async-std with the intent to publish version 1.0 by September 26th, 2019. async-std is a library that looks and feels like the Rust standard library, except everything in it is made to work with async/await exactly as you would expect it to. The library comes with a book and polished API documentation, and will soon provide a stable interface to base your async libraries and applications on. While we don't promise API stability before our 1.0 release, we also don't expect to make any breaking changes.
Effectively developing systems and operating them in production requires visibility into their behavior at runtime. While conventional logging can provide some of this visibility, asynchronous software — like applications using the Tokio runtime — introduces new challenges.
tracing is a collection of libraries that provide a framework for instrumenting Rust programs to collect structured, context-aware, event driven diagnostics. Note that tracing was originally released under the name tokio-trace; the name was changed to reflect that, although it is part of the Tokio project, the tokio runtime is not required to use tracing.
async httpToday we're happy to announce Surf, an asynchronous cross-platform streaming HTTP client for Rust. This project was a collaboration between Kat Marchán (Entropic / Microsoft), Stjepan Glavina (Ferrous Systems), and myself (Yoshua Wuyts). Surf is a friendly HTTP client built for casual Rustaceans and veterans alike.
async tokioWe’re pleased to announce the release of the first Tokio alpha with async & await support. This includes updating all of the Tokio crates to use std::future instead of futures 0.1. It also includes adding async fn versions of the APIs.
parsersTree-sitter is a parser generator tool and parsing library. It generates portable parsers that can be used in several languages including Rust. Tree-sitter grammars are available for several languages. This is a game changer because it lowers the barrier to entry for writing language tooling. You no longer need to write your own parser. With Tree-sitter, you can now simply use an existing parser.
One of our clients helps companies in becoming GDPR-compliant. A goal is to recognize sensitive pieces of user data in a big pile of registrations, receipts, emails, and transcripts, and mark them to be checked out later. As more and more data is collected by companies, finding and eliminating sensitive data becomes harder and harder, to the point where it is no longer possible for mere human employees to keep up without assistance.
Welcome to the seventh edition of _This month in rustsim_! This monthly newsletter will provide you with a
Lately, I’ve been wanting to re-write demoscene-like applications. Not in the same mood and way as I usually did, though. Instead, I want to build small things for people to play with. A bit like small and easy to use audiovisual experiences (it can be seen as small video games for instance, but focused on the artistic expression as some games do).
The thing is, the kind of program we want generates its own inputs based on, mostly, the speed at which the hardware it’s running on is able to render a complete frame. The faster the more accurate we sample from that continuous function. That is actually quite logical: more FPS means, literally, more images to sample. The difference between two images will get less and less noticeable as the number of FPS rises. That gives you smooth images.
The “challenge” here is to write code to schedule those images. Instead of taking a parameter like the time on the command-line and rendering the corresponding image, we will generate a stream of images and will do different things at different times. Especially in demoscene productions, we want to synchronize what’s on the screen with what’s playing on the audio device.
httpOne of my more recent projects that I have been putting a lot of effort into is a Rust HTTP client called cHTTP, which I introduced on this blog over 18 months ago. Here I want to share an update on the direction of the project, and also give some detail on what months of late evenings and weekends produced in version 0.5 just published today.
Thanks to the diligent work of Laurent Mazare on his tch-rs crate, the Rust community can now enjoy an easy access to the powerful Torch neural net framework. Being personally an avid user of both Rust and Torch, stumbling on this repo has been nothing but a belated birthday present. In this post, I would like to dive into two examples to present its most fundamental functionalities.
Earlier this month we released Abscissa: our security-oriented Rust application framework. After releasing v0.1, we’ve spent the past few weeks further polishing it up in tandem with this blog post, and just released a follow-up v0.2.
We are proud to announce the release of our software codenano, available at https://dna.hamilton.ie/codenano/. Here, we give an introduction to what codenano can and can not do. The source code for codenano is hosted on a github repository: https://github.com/thenlevy/codenano, along with a short tutorial.
Codenano allows one to design and visualise DNA nanostructures specified using code, all in your browser. Codenano also has the ability to compute some simple interactions between DNA bases in order to help the user design DNA nanostructures that are feasible according to some simple criteria.
asyncIf you are familiar with Python ecosystem, probably you had heard about psutil package — a cross-platform library for retrieving information about system processes and system utilization (CPU, memory, disks, network and so on). It is very popular and actively used package, which has analogs in other languages: gopsutil for Golang, oshi for Java, you name it. Rust, of course, is not an exception here: we do have psutil, sysinfo, sys-info and systemstat crates.
Now, despite the tremendous work that had been done already by the authors of these crates, I’m excited to announce what I’ve been working on for the past three months: “heim” project — library for system information fetching.
memory-management🌵 CactusRef is a single-threaded, cycle-aware, reference counting smart pointer [docs] [code]. CactusRef is nearly a drop-in replacement for std::rc1 from the Rust standard library.
I am a big fan of simplifying the existent code in futures-rs using async/await syntax. My goal was to rewrite the combinators in such a way that even a newbie can understand what was going on. However I met several issues with Stream combinators because it was a little bit hard to construct an impl Stream without defining a struct with a ::poll_next method. So I used Stream::unfold that can create streams from a closure.
glam is a simple and fast Rust linear algebra library for games and graphics. mathbench is a set of unit tests and benchmarks comparing the performance of glam with the popular Rust linear algebra libraries cgmath and nalgebra. The following is a table of benchmarks produced by mathbench comparing glam performance to cgmath and nalgebra on f32 data.
asyncI recently migrated a small/medium-sized crate from Futures 0.1 to 0.3. It was fairly easy, but there were some tricky bits and some things that were not well documented, so I think it is worth me writing up my experience.
How to write a well behaved C-API library in Rust.
Today we'd like to introduce async-log, a general-purpose crate that extends the standard log crate with asynchronous metadata. This is a first step in introducing full-fledged asynchronous tracing capabilities to Rust.
markdownA simple tool to display static or dynamic Markdown snippets in the terminal, with skin isolation. Based on crossterm so works on most terminals (even on windows).
javascriptA blog about learning computer science concepts with practical projects
swym is a transactional memory library that prioritizes performance. It’s not lock-free, but it does have progress guarantees. This post will explore some of the recent work on swym’s progress promises, as well as some comparisons with non-blocking algorithms. I’m not an expert on schedulers or OS’s, so please correct me if anything is wrong. It might benefit swym!
parsers nomnom, the Rust parser combinators library, is now available at version 5. This is the most mature version of nom. This is the one that feels “done”. This is the parser library that I wanted when I started nom 5 years ago. It’s here at last. nom 5 is a complete rewrite of the internal architecture, to use functions instead of macros, while keeping backward compatibility with existing macros based parsers, and making the error type completely generic.
gtkWelcome everyone to this whole new gtk-rs release! Time to check what was added/updated in this new version.
guiI’m one of the maintainers of Winit, the main pure-Rust window creation library. Even if you haven’t used it directly, you’ve probably heard of projects that depend on it - Servo and Alacritty being the best-known applications that depend on our codebase. If you’ve done any graphics programming in Rust using Glutin (or dependent projects including gfx-rs, Glium, and Amethyst) we’ve been the ones making the windows actually show up on your desktop.
This announcement details the major changes since Winit 0.19. Also, we are looking for new contributors! If you are interested in working on the foundations of Rust’s GUI story, now is a great time to join the project.
The base of Rust users and contributors is growing steadily. The amount of libraries (aka crates) at http://crates.io is growing quickly; the overall “noise” is increasing. Some libraries might not be maintained any longer 🙁
This annotated catalogue shall help the Rust-users to find specific, popular, mature Rust crates. This list is WIP (Work In Progress), reflecting my personal shortlist. The ordering in the table top-down doesn’t express any preference.
Today I’ll be continuing my series of posts on the rust implementation of the Mercurial version control system I’ve been working on. In this post I’ll be focusing on what I learned this week about the rust module system as well as a few helpful crates I discovered to aid in command-line argument parsing and error handling.
This is partly an announcement of a new crate folks might find useful, partly a call for participation and help and partly a journal like story how the crate came to being. Read on (or not) or skip to the parts that seem interesting to you.
Actix web 1.0.0 is released - a small, pragmatic, and extremely fast web framework.
Recently Gorka Irazoqui Apecechea and me proudly published a VRF crate as an open source project under the MIT license. The library is a fast, flexible and general-purpose Verifiable Random Function (VRF) library written in Rust, which follows the IETF standard draft written by Sharon Goldberg, Moni Naor, Dimitris Papadopoulos, Leonid Reyzin, and Jan Včelák.
Orkhon is Rust framework for Machine Learning to run/use inference/prediction code written in Python, frozen models and process unseen data. It is mainly focused on serving models and processing unseen data in a performant manner. Instead of using Python directly and having scalability problems for servers this framework tries to solve them with built-in async API.
j4rs stands for “Java for Rust” and allows effortless calls to Java code, from Rust. Some time ago, on a need to call Java code from Rust, I started the j4rs project. The main idea was to implement a crate that would give the ability to its users to make calls to Java easily, so that they can benefit from the huge Java ecosystem.
testingWe’re proud to announce mockiato! For the last few months, we tackled the issue of creating a usable mocking library. Our primary goals were: Ease of use: The mocks are written in idiomatic Rust and don’t rely on custom macro syntax. Maintainability: The entire code base strives to follow the rules of Clean Code and Clean Architecture as specified by Robert C. Martin. Strict expectation enforcement: Mockiato catches unexpected behavior as soon as it happens instead of returning default values.
During my work at Adgear, I’ve been working for a while on an async IO library for Rust. This post will present this new library. This library takes a very different approach than most other async IO libraries in Rust: it is actually inspired by the Pony programming language. So, it does not use futures, it does not use async/await: it just provides simple trait
My crate offers a powerset enum - an enum that can be parametrized to any subset of it's variants. I also included a macro for doing this parametrization by providing the types of the variants - so for example Error![std::io::Error, serde_json::Error] would generate a subset of the enum that can only have IO and JSON parsing errors.
With these crates in a basic but usable state, I went to write papers, Rust code to gather data from the above sources, and inject them into Wikidata. I wrote a Rust trait to represent a generic source, and then wrote adapter structs for each of the sources. Finally, I added some wrapper code to take a list of adapters, query them about a paper, and update Wikidata accordingly.
gnome gtkLast week, I went to the fifth Rust+GNOME hackfest which was in Berlin again. My goal for this hackfest was to fix this issue I opened nearly three years ago. The problem is that sometimes you want to create a widget or an object and set some properties at construction time. This might be needed when you want to set construct-only properties. For instance, you might want to create a webkit2gtk::WebView with a WebContext and a UserContentManager at the same time. That’s why a constructor was manually written for this use case.
Custom completion behavior is configured using a special bash built-in called complete. complete can be used to designate either a bash function or any other command as the completion script for a particular command. When the user requests completions for a command, complete will run specified code, passing in as args information about what the user has already typed, and expecting as output the completion suggestions. Typically these completion scripts are written in bash, but we’ll look at how it is possible to write them in Rust.
In this tutorial, we’ll see how to generate a GNOME library using the gir crate. A few things to note first: It only works on GObject-based libraries. You need .gir files.
A little explanation about those requirements: the gir crate needs .gir files to generate the library API. You can generally find them alongside the library header files (as you can see here for example, look for “.gir”).
The .gir files “describes” the library API (objects, arguments, even ownership!). This is where the gir crate comes in: it reads those .gir files and generates the Rust crates from them. You can learn more about the GIR format here.
A beginners guide to using StructOpt for parsing command line arguments.
Today we're announcing paw, a first step by the CLI Working Group to make command line applications more first class in Rust.
We introduce a procedural macro paw::main that allows passing arguments to fn main, and a new trait ParseArgs that must be implemented by the arguments to main. This allows passing not only the classic std::env::Args to main but also, for example, structopt instances.
In general, using phrase falls into 3 steps: Counting n-grams, Exporting scored models, Significant term/phrase extraction/transform N-gram counting is done continuously, providing batches of documents as they come in. Model export reads all n-gram counts so far and calculates mutual information-based collocations - you can then deploy the models by shipping the binary and data/scores_* files to a server. Labeling (identifying all significant terms and phrases in text) or transforming (eager replace of longest found phrases in text) can be done either via the CLI or the web server. Providing labels for documents is not necessary for learning phrases, but does help, and allows for significant term labeling also.
Hello people. It’s been weeks I have started to work on luminance-1.0.0. For a brief recap, luminance is a graphics crate that I originally created in Haskell, when I ripped it off from a demoscene engine called quaazar in order to make and maintain tiner packages. The Rust port was my first Rust project and it became quickly the default language I would develop graphics applications in.
At Pixability my team and I recently created a pretty neat AWS Batch driven system. AWS Batch can can handle almost any task seamlessly and it’s pretty easy to manage with the console. This is great already, but I was curious to see what it would look like to monitor Batch with Rust. Watchrs was inspired by this question and currently provides basic functionality to do so. In this post we will be briefly going over how the main components of watchrs were built and how to use them all together.
Below are 6 Rust macros that are worth taking a look at to improve your project. Rust macros are a great feature that can reduce code boilerplate and be a time saver for programmers. They also offer flexibility for developers to use metaprogramming to add new features to the language and package them in a way that is easy to integrate into code. They are one of the more powerful features of the language and this led me to search github and cargo to see what was out there. Below are some interest macros that are not as well known.
today, I’m going to talk about the splines crate. And more specifically, the splines-1.0.0-rc.1 release candidate I uploaded today on crates.io. Maybe you’re wondering what a spline is, in the first place. A spline is a mathematic curve that is defined by several polynomials. You can picture them mentally by several small and simple curves combined to each others, giving the curve an interesting shape and properties. Now why we want splines is easy to understand: imagine a curve, something smooth and a bit complex (maybe even with loops). Now, imagine you want to make an object move along that curve. How do you represent that curve and how to you “make something advance along it?”
This provides a high-level binding to emacs-module, Emacs's support for dynamic modules.
I’ve just crossed a 20K LOC in one of my bigger Rust projects, and thought about pausing for a moment and sharing some great Rust libraries that I’ve used.
Rust’s futures ecosystem is currently split in two: On the one hand we have the vibrant ecosystem built around futures@0.1 with its many libraries working on stable Rust and on the other hand there’s the unstable std::future ecosystem with support for the ergonomic and powerful async/await language feature. To bridge the gap between these two worlds we have introduced a compatibility layer as part of the futures@0.3 extension to std::future. This blog post aims to give an overview over how to use it.
The vision of the Async Ecosystem WG is to refine the async Rust experience until it matches the quality and ease of working with today's std. There are a lot of components in that vision, including async/await syntax and borrow checker integration. Today, though, we'd like to introduce another component: Runtime, a crate that makes working with async code feel closer to working with std, and a stepping stone toward ecosystem standardization.
AV1 is a modern video codec brought to you by an alliance of many different bigger and smaller players in the multimedia field. rav1e: The safest and fastest AV1 encoder, built by many volunteers and Mozilla/Xiph developers. crav1e: A companion crate, written by yours truly, that provides a C-API, so the encoder can be used by C libraries and programs. This article will just give a quick overview of the API available right now and it is mainly to help people start using it and hopefully report issues and problem.
There’s a dearth of blog posts online that cover the details of implementing a custom protocol in tokio, at least that I’ve found. I’m going to cover some of the steps I went through in implementing an async version i3wm’s IPC.
MeiliES is an Event Sourcing database that uses the RESP (REdis Serialization Protocol) to communicate. We use the Redis protocol to simplify clients implementation. The portability problematics are resolved by using a full Rust implementation (we are using Sled as internal storage).
Zola gets the beginning of multi-lingual support and perf improvements.
Sonic is a fast, lightweight and schema-less search backend. It ingests search texts and identifier tuples that can then be queried against in a microsecond's time.
barrel makes writing migrations for different databases as easy as possible. It provides you with a common API over SQL, with certain features only provided for database specific implementations. This way you can focus on your Rust code, and stop worrying about SQL.
Over the past few months, I’ve implemented hmmm, a Rust library for Hidden Markov Models (HMMs). HMMs are a well-established statistical machine learning technique for modeling sequences of data. They have been applied to problems like speech recognition and bioinformatics. They are called “hidden” because each discrete time step is associated with a hidden state. Below, I’ll briefly discuss some challenges that I ran into while implementing this library.
After the librsvg team finished the rustification of librsvg's main library, I wanted to start porting the high-level test suite to Rust. This is mainly to be able to run tests in parallel, which cargo test does automatically in order to reduce test times. However, this meant that librsvg needed a Rust API that would exercise the same code paths as the C entry points. At the same time, I wanted the Rust API to make it impossible to misuse the library.
pulldown_cmark, a fast pull parser for the CommonMark markdown standard written in Rust, has just seen its 0.3 release. It marks a milestone for renewed CommonMark test compliance and performance. In this blog post, we'll have a quick look at the goals of the rewrite, how they were achieved and what's next for the crate.
Persy is a single file storage engine, all the data, referencing structures and logs are kept in a single file. Persy support read-committed transactions, using copy on write to guarantee high concurrency and isolation, the data consistency is guaranteed by a transaction log that recover the operations in case of crash. Persy provide segments to organize records of different kinds and allow the scan on a single segment. Persy provide index implementation that can be used to associate any simple value to another value or a record reference. In Persy a record is a simple Vec<u8>, the content of the record is ignored by Persy, is just stored and retrieved on request.
Last time we defined a minimum viable implementation for mutagen statement removal: Remove only function call statements whose results are not returned from the surrounding block and whose AST do not contain any Assign expressions.
This time in our “Mutating Rust” series, we want to tackle the most complex mutation so far: Statement removal. Now why do I think this is complex? It’s just removing the statement (or, as we bake our mutations into the code, activating at runtime, putting it behind an if), right?
This is the second post in a series detailing the issues, progress, and design decisions used in clap v3. This post details removing the "stringly typed" nature of clap.
Hey rustaceans! With a little help from your feedback just a few hours ago, I'm proud to present to you all Starling, a Binary Indexed Merkle tree! I've been working on this data structure for the last few months, and I think it is ready now for the community to have a look. Let me explain what it is and why it might be useful for your project.
I have a problem. I don't know what to call it, but there is this itch I have when I find something that can obviously be made more efficient. The way I get about scratching that itch these days is by writing some Rust. I just got such an itch when looking at static site generators for this blog, Hugo (written in Go) is pretty much state-of-the art. In Rust land we have Zola, which is feature-rich, mature (for v0.5), and definitely fast enough for most users, and yet it is not as fast as it could be. Being written in Rust is, by itself, not a guarantee of top performance. The implementation matters. After some looking around I've narrowed down a problem I want to tackle (for now) to one area: template engines.
Welcome to the fourth edition of This month in rustsim. This monthly newsletter will provide you with a summary of important update that occurred within the rustsim community. This includes in particular updates about the nphysics, ncollide, nalgebra, and alga crate.
After the previous two posts this post will go step by step through how the mail crate can be used to create mails based on a handlebars template and send them to a Mail Submission Agent (MSA).
This post is to announce a new Rust library for low-level text layout, called “skribo” (the Esperanto word for “writing”). This has been a major gap in the Rust ecosystem, and I hope the new crate can improve text handling across the board.
A new version packed with new features and improvements: Blink leds without blocking main thread and adjust brightness. ⚙️ Work with servo motors and adjust motor speed. 〜 Work with software PWM.
We’ve been working steadily to get Wasmer to execute WebAssembly modules on the server-side as fast as possible. TL;DR — We got 100x improvement on startup time on Wasmer 0.2.0.
My new pet project for reading batteries information in Rust.
I’m super excited about WebAssembly! It’s fast, (can be) small, and extremely portable. In fact, I wouldn’t be surprised if in the near future most web developers write code that eventually gets compiled to Wasm.
But currently there’s not a huge selection of resources showing how to get started with WebAssembly, and I couldn’t find any tutorials that worked with create-react-app. Most focus on writing and compiling a module, but rush over the details of actually using Wasm code. What follows is a basic setup for a React app using WebAssembly that should serve as a good foundation for more complex applications.
The error handling features within Rust are some of my favorite things about the language. This system works great when you are in a function which returns a Result and you want to exit at the first error you come to. However, it can be challenging if your goal is to try a few failure-prone things and return each of the errors, rather than just the first error. This is the problem multi_try attempts to solve.
We encrypt these secrets in so-called ansible vaults. Kuberwave also has the need to access these secrets. Because our staff is already comfortable with using these vaults, we’ve decided to also employ them for our Kubernetes setup. For this I created ansible-vault-rs, a library that can decrypt ansible vaults. Note that it can not create or edit vaults, because I have no need (yet) for this functionality.
It’s time for a new release! Main adds/changes this time are: We added the generation of the Atk crate. We now generate functions taking callback as parameters. We improved the channels handling in GLib. The whole new GString type! The minimum Rust version supported is now the 1.31. The minimum version of all libraries has been changed to GNOME 3.14. The maximum version of all libraries has been upgraded to GNOME 3.30. Added subclassing support in GLib. Even more bindings generated. Let’s see those in details.
161 votes and 6 comments so far on Reddit
In 2018 my activity on the project has varied depending on the time and energy I have had left after work and other activities. As it turns out, working on getting WebRender shipped in Firefox is at the same time amazing and very demanding, and what's left of my brain after a good day of work isn't always up to some of the ambitions I have planned for lyon. Fortunately I am not the only one who contributed to the project, and while progress was slow on the most ambitious plans, I did spend some time on smaller features and polish.
I'll get to these big plans towards the end of this post. In the mean time let's look at some of the highlights of what changed in lyon in 2018.
I’ve released the ieee802154 crate, a partial implementation of the IEEE 802.15.4 standard, earlier this week. IEEE 802.15.4 is a standard for low-rate wireless personal area networks. It is used as the basis for higher-level protocols like 6LoWPAN, Zigbee, or Thread. The ieee802154 crate is only a partial implementation of this standard, but I hope it can be used as a basis for future work by extending it as required.
I’m pleased to announce the release of metered-rs, a crate that helps live measurements of code, inspired by Coda Hale’s Java metrics, with the philosophy that measuring program performance at runtime is valuable, and independent from benchmarking.
The mail crate is a modular Rust library for creating, modifying and then encoding mails. It also has bindings to our new crate new-tokio-smtp to allow sending mails asynchronously, as well as bindings to handlebars for creating mails from templates. It currently does not support parsing mails, but is designed in a way that decoding capabilities could be easily added in the future (contact me if that is something you'd be interested in working on!). At 1aim, we are already using mail in production.
This is a follow-up post to Lock-freedom without garbage collection from 2015, which introduced Crossbeam, a Rust library that implements efficient lock-free data structures without relying on a tracing garbage collector.
This is the first part in a three part blog post about (e-)mails and how to create, encode and send them using the mail crate (a library).
Serde serializable and deserializable trait objects. This crate provides a macro for painless serialization of &dyn Trait trait objects and serialization + deserialization of Box<dyn Trait> trait objects.
The recent release of Flutter 1.0 was quite exciting for me. I’m not much of anGUI person nor do I make a lot of mobile apps but after looking through some examples, I started to like their take on UI frameworks. In particular, the three aspects mentioned above seemed to be handled very well!
Back to Rust. I wondered how to adopt a similar API under the strict eyes 👀 of the borrow- and typechecker — which resulted in the experimental UI framework paw (in progress..)
Thanks to Mozilla and Igalia I have the opportunity to work on Servo, adding it HTML5 multimedia features. First, with the help of Fernando Jiménez, we finished what my colleague Philippe Normand and Sebastian Dröge (one of my programming heroes) started: a media player in Rust designed to be integrated in Servo. This media player lives in its own crate: servo/media along with the WebAudio engine. A crate, in Rust jargon, is like a library. This crate is (very ad-hocly) designed to be multimedia framework agnostic, but the only backend right now is for GStreamer. Later we integrated it into Servo adding an initial support for audio and video tags.
I'm announcing a new library for procedural macro authors: proc-macro-rules (and on crates.io). It allows you to do macro_rules-like pattern matching inside a procedural macro. The goal is to smooth the transition from declarative to procedural macros (this works pretty well when used with the quote crate).
Let’s talk about Layout. Layout is fundamental to any UI application. The layout engine is what takes a set of rules and figures out where to place elements on the screen. This sounds simple enough but as UIs become increasingly complex we rely more on the layout engine to be able to create these UIs as easily as possible. Not only should it be easy to build these UIs but the engine performing this work is also required to do so at minimum cost as it runs possibly on every frame (for example when performing layout animations).
Integrate Ruby with your Rust application. Or integrate Rust with your Ruby application. This project allows you to do either with relative ease.
These days, we hardly think about URLs. Popular content management tools default to so-called “pretty” slugs, and even here, on secretfader.com, I remove stop-words to ensure the tidiest, most SEO-friendly URLs possible.
It would be easy to forget the struggles that led to best practices of today’s web. However, to my generation of internet hackers, URLs riddled with ampersands and question marks were entirely normal. In those days, assuming URLs would be comprised of hyphenated alphanumeric characters was obviously unsafe; instead, we learned the official standard for parsing and constructing URLs.
generic-array is a method of achieving fixed-length fixed-size stack-allocated generic arrays without needing const generics in stable Rust.
This is yet another library for writing parsers in Rust. What makes this one different is that I've combined some existing academic work in a way that I think is novel. The result is an unusually flexible parsing library while still offering competitive performance and memory usage.
Announcement of version 0.2 of smithay, now providing the fundamentals of a wayland compositor.
err-derive A failure-like derive macro for the std Error. The source code is mostly copied from failure-derive.
A project to make Postgres extensions in Rust easy, you might learn how to use macro_rules, attribute macros, allocators and some FFI in this post.
Recently there has been some discussion about interprocedural borrowing conflicts in rust. This is something I’ve been fighting with a lot, especially while working on my SAT solver varisat. Around the time Niko Matsakis published his blog post about this, I realized that the existing workarounds I’ve been using in varisat have become a maintenance nightmare. Making simple changes to the code required lots of changes in the boilerplate needed to thread various references to the places where they’re needed.
While I didn’t think that a new language feature to solve this would be something I’d be willing to wait for, I decided to sit down and figure out how such a language feature would have to look like. I knew that I wanted something that allows for partial borrows across function calls. I also prefer this to work with annotations instead of global inference. While trying to come up with a coherent design that fits neatly into the existing type and trait system, I realized that most of what I wanted can be realized in stable rust today.
A year ago, Tokio was a very different library. It includes the (now deprecated) tokio-core which provided a future executor, I/O selector, and basic TCP/UDP types in a single library. It also included tokio-proto, but we won't talk about that. Over the past year, Tokio has grown to become Rust's asynchronous I/O platform. It has been adopted by a number of large companies to build apps.
I think it’s part of the human condition to ignore perfectly good advice when it comes our way. A bit over a month ago, I was dispensing sage wisdom for the ages: I had a really great idea: build a custom allocator that allows you to track your own allocations. I gave it a shot, but learned very quickly: never write your own allocator. I proceeded to ignore it, because we never really learn from our mistakes. There’s another part of the human condition that derives joy from seeing things explode. And that’s the part I’m going to focus on.
My favorite way to explore Lambdas is to build Alexa skills because of the immediate feedback: you write a little code, and a home device talks to you. It’s a peek into the long promised of world of easy service composition.
Unfortunately, Rust didn’t have complete Alexa skill request/response handling (there is a crate from 2 years ago that handled only the basics), so I wrote one called alexa_sdk. (It’s basically a struct plus serde wrapper around the Alexa JSON spec, with some helpers.
In short, when writing a daemon or a service, we have the „muscle“ of the application ‒ whatever we write the daemon for. And we have a whole lot of infrastructure around that: logging, command line parsing, configuration. And while there are Rust libraries for all that, one needs nontrivial amount of boilerplate code to bridge all this together. Spirit aims to be this bridge.
encoding_rs is a high-decode-performance, low-legacy-encode-footprint and high-correctness implementation of the WHATWG Encoding Standard written in Rust. In Firefox 56, encoding_rs replaced uconv as the character encoding library used in Firefox. This wasn’t an addition of a component but an actual replacement: uconv was removed when encoding_rs landed. This writeup covers the motivation and design of encoding_rs, as well as some benchmark results.
During my work life, I spend a lot of time working with MapReduce-style workflows, particularly with Hadoop infrastructure. A lot of this work is spent with larger amounts of data in order to implement the batch layer of the Lambda architecture. Due to this, the largest concern is that the behaviour is consistent across both the batch layer and the realtime layer - naturally you wouldn't want sporadic behaviour across the two. The easiest way to do this is to share code across the layers, to avoid having to keep implementations in sync. We have recently been working with Rust, which has been a little difficult to integrate with Hadoop MapReduce flows due to the fact it's mainly written in Java. It's because of this that I began to work on a small library named Efflux. It's designed as a very small interface to the MapReduce pattern, and implemented in Rust to allow us to share code across the batch layer easily.
A couple of weeks ago we had the fourth GNOME+Rust hackfest, this time in Thessaloniki, Greece. We held the hackfest at the CoHo coworking space, a small, cozy office between the University and the sea. Every such hackfest I am overwhelmed by the kind hackers who work on [gnome-class], the code generator for GObject implementations in Rust.
Less than 2 weeks ago, I was working on improving the integration of Rust with GNOME libraries at the fourth Hackfest, which happened this time in Thessaloniki.
A recent project has led me to have a go at writing an XML parser. I thought I’d document my experiences using pest to implement a lexer using the EBNF-esque formal grammar.
In this post, I present a wait-free thread-local storage using the Rust language.
This is the second article about my experience at supporting pest in my glsl crate – without, for now, removing the nom parser.
This is the first article out of a (I guess?!) series of upcoming articles about… parsing. More specifically, I’ve been writing the glsl crate for a while now and the current, in-use parser is backed with nom. nom is a parser combinator crate written originally by @geal and there has been a lot of fuzz around nom vs. pest lately.
Soooooooooooo. Because glsl is written with nom in its third iteration and because nom is now at version 4, I decided it was time to update the parser code of glsl. I heard about the comparison between pest and nom and decided to write an implementation with pest.
This is the first article of a series about how writing a pest looks like is fun compared to writing the nom parser. I’ll post several articles as I advance and see interesting matter to discuss and share.
Gutenberg changes name to Zola and gets a big release to celebrate.
Hello everyone! I would like to share an update on an open source project which I have been developing for a little while now. It is a Rust project called Wither which attempts to “provide a simple, sane & predictable interface into MongoDB, based on data models”. This post is about the 0.6 release of this crate, and I would like to dive into some of the aspects of developing this release which I really enjoyed or which I found interesting.
Mundane is a cryptography library written in Rust and backed by BoringSSL. It aims to be difficult to misuse, ergonomic, and performant (in that order). It was originally created to serve the cryptography needs of Fuchsia, but we’ve decided to split it off as a general-purpose crate.
I realized I kept copy and pasting (or re-writing) functions to take user input or read simple files into Rust variables. So I’ve been working on a Rust library that attempts to make these tasks easier.
In contrast to many other ECS, iteration in Pyro is fully linear. Different combinations of components always live in the same storage. The advantage is that iteration is always fully linear and no cache is wasted. The storage behind the scene is a SoA storage.
For many of my use cases for running Rust applications, storing configuration in file format is less attractive as I’m typically running Rust inside docker containers and container orchestrators typically encourage the use of standard interfaces like the env for configuration. So I pondered 🤔, “What if I could treat my program’s env parameterization with the same level of typing I treat my functions and enclosing types with while getting everything one get’s from using serde for free?” That would be the bee’s knees 🐝 . Enter: envy.
Recently, I wrote ndarray-csv, a Rust crate for converting between CSV files and 2D arrays. There are already crates for CSV and arrays, so how exciting could this possibly be? Actually, there was a lot more to it than I had thought: although it started out as a two-hour project, I ended up rewriting the entire thing three times!
Rust SGX SDK, maintained by Baidu X-Lab, is a convenient framework to develop secure trusted computing applications for Intel SGX enclaves. Based on it, developers can easily build trusted SGX enclaves with memory safety guarantees. This adds an extra strong (and strongest ever) security layer over the SGX isolation, further keeping attackers away from the secrets in enclave even if they compromised the privileged software environment (operating system, hypervisor, etc.). Rust SGX SDK thus means a lot to privacy protection and trusted computing on public cloud platforms and blockchains.
Today I realized a new crate called pin-cell. This crate contains a type called PinCell, which is a kind of cell that is similar to RefCell, but only can allow pinned mutable references into its interior. Right now, the crate is nightly only and no-std compatible.
How is the API of PinCell different from RefCell? When you call borrow_mut on a RefCell, you get a type back that implements DerefMut, allowing you to mutate the interior value.
pest is a general purpose parser written in Rust with a focus on accessibility, correctness, and performance. It uses parsing expression grammars (or PEG) as input, which are similar in spirit to regular expressions, but which offer the enhanced expressivity needed to parse complex languages.
After releasing the Rusty ECMA Script Scanner (RESS) 0.5, my next big effort in the Rust+Javascript space is to increase the amount of documentation. This post is an effort to clarify what RESS does and how someone might use it.
Merlin is a small Rust library that performs the Fiat-Shamir transformation in software, maintaining a STROBE-based transcript of the proof protocol and allowing the prover to commit messages to the transcript and compute challenges bound to all previous messages. It also provides a transcript-based RNG for use by the prover, generalizing “deterministic” and “synthetic” nonces to arbitrarily complex zero-knowledge protocols.
orion is another attempt at cryptography implemented in pure Rust. Its main focus is usability. This is in part achieved by providing a thorough documentation of the library. High-level abstractions are also provided, which are an attempt at guiding the users towards safe usage of the lower-level functionality of the library.
After almost 6 months, a new release of the GStreamer Rust bindings and the GStreamer plugin writing infrastructure for Rust is out. As usual this was coinciding with the release of all the gtk-rs crates to make use of all the new features they contain.
Sonnerie is a time-series database. Map a timestamp to a floating-point value. Store multiple of these series in a single database. Insert tens of millions of samples in minutes, on rotational media.
Spirit is a crate that cuts down on boilerplate when creating unix daemons, with support for live configuration reloading.
Recently I've been working again in the rust port of libgepub, libgepub is C code, but in the rust-migration branch almost all the real functionality is done with rust and the GepubDoc class is a GObject wrapper around that code. For this reason I was thinking about to use gnome-class to implement GepubDoc. Gnome-class is a rust lib to write GObject code in rust that's compatible with the C binary API so then you can call this new GObject code written with gnome-class from C. So, libgepub is the excuse to start to implement GIR in gnome-class.
Access Control Lists (ACLs) are an integral part of the Microsoft Windows security model. In addition to controlling access to secured resources, they are also used in sandboxing, event auditing, and specifying mandatory integrity levels. They are also exceedingly painful to programmatically manipulate, especially in Rust. Today, help has arrived — we released windows-acl, a Rust crate that simplifies the manipulation of access control lists on Windows.
In this blog post I’d like to present toykio, a simple futures executor intended for learning about how executors with an event loop work. Toykio only provides a very minimal feature set: An event loop and TCP streams and listeners. However, it turns out that due to the fact that futures are composable, this is enough to build complex clients and servers.
A compatibility layer between 0.3 an 0.1 was developed. It is now possible to convert an 0.3 future into an 0.1 future and vice versa. Similar conversions for streams and sinks are also supported. Additionally, it is now possible to run 0.3 futures and async functions on Tokio’s executor. We have a dedicated blog post coming up that explains this in more detail.
The short version is, Tower Web 0.2 was just released and regular Rust attributes are now used instead of magic comments. The doc comment is replaced with #[get("/")]. This is thanks to Rust macro wizard David Tolnay. I also thought that it would be best to immediately push out 0.2 and then we can all pretend 0.1 didn’t happen.
We’ve just published a rust port of our PrettySize.NET library, now available via cargo and github. Like its .NET predecessor, PrettySize-rs aims to provide a comprehensive API for dealing with file sizes, covering both manipulation and human-readable formatting.
With a brand new tutorial and a ton of new features, including prefabs, controller support, MP3 audio, localisation and an even better ergonomics!
I’ve been working with diesel and serde. I use diesel for my postgres datastore, and serde for serializing/deserializing data to the web. Recently I came across a situation where I needed to define my type in diesel as well as implement deserialize in serde. The example below is a fairly simple so it makes for a good example to share so others can learn (and so I can remember how all this works next time I need it).
Gutenberg 0.4.0 is out with custom taxonomies, image processing, improved shortcodes and more.
intl_pluralrules is a Rust crate, built to handle pluralization. Pluralization is the foundation for all localization and many internationalization APIs. With the addition of intl_pluralrules, any locale-aware date-, time- or unit-formatting (“1 second” vs “2 seconds”) and many other pluralization-dependent APIs can be added to Rust.
This past fall, three former GnuPG developers began working on a new OpenPGP implementation in Rust called Sequoia. As it’s starting to shape up and become useful, I feel now is a good time to announce the project to the larger Rust community, and hopefully get some feedback before our first release.
Over the past several months, I’ve been working a web framework in Rust. I wanted to make use of the new hyper 0.12 changes, so the framework is just as fast, is asynchronous, and benefits from all the improvements found powering Linkerd. More importantly, I wanted there to be a reason for making a new framework; it couldn’t just be yet another framework with the only difference being I’ve written it. Instead, the way this framework is used is quite different than many that exist. In doing so, it expresses a strong opinion, which might not match your previous experiences, but I believe it manages to do something really special.
I’m super excited to reveal warp, a joint project with @carllerche.
In this post I'd like to introduce a serdebug helper which is a drop-in replacement for #[derive(Debug)] with some of the advanced features that serde can provide.
Using C libraries in a portable way involves a bit of work: finding the library on the system or building it if it's not available, checking if it is compatible, finding C headers and converting them to Rust modules, and giving Cargo correct linking instructions. Often every step of this is tricky, because operating systems, package managers and libraries have their unique quirks that need special handling.
Fortunately, all this work can be done once in a build script, and published as a <insert library name>-sys Rust crate. This way other Rust programmers will be able to use the C library without having to re-invent the build script themselves.
Several new changes while working towards 0.3.
I’m excited to announce the brand new website/user-guide for the nphysics2d and nphysics3d crates: pure-rust 2D and 3D real-time physics engines with rigid bodies and joints! Online wasm-based demos are also provided (see for example the Multibody joints 34 demo).
Welcome to the inaugural post of the new futures-rs blog!
After several months of work, we’re happy to announce an alpha release of the new edition of future-rs, version 0.3. The immediate goal of this work is to support async/await notation (with borrowing) in Rust itself, which has entailed significant changes to the futures crate.
wayland-rs is a set of crates providing generic APIs to manipulate the Wayland protocol, successor of X11 for linux windowing.
Here I am finally, after having hinted at the possibility and finally taken the time to write and merge quite an epic pull request, I can finally say it: wayland-rs is now a pure rust implementation of the protocol, rather than a crate of bindings to the wayland system C libraries.
Thirty times faster than Handlebars, half the features! I am introducing a new templating engine for Rust that is designed to be robust and very fast! Why Zapper? Runtime templating is amazing, since you can reload templates on the fly or even allow users to provide their own templates, yet runtime templating engines are rarely fast. Templates that are statically compiled into your application can be super fast, but are completely inflexible. Recompiling and restarting your application just to change a template is especially boring. Zapper combines the flexibility of runtime templating with great performance!
Better HTTP Upgrades with hyper It’s been possible to handle HTTP Upgrades (like Websockets) in hyper if you made use of the low-level APIs in the server and client, but it wasn’t especially nice to...
In one of my recent blog posts, I talked about Event Sourcing with Aggregates in Rust. In that post, I was just beginning to explore how the Rust language and its strongly typed native data structures would allow me to express event sourcing concepts and primitives. I have now created an initial version of an Event Sourcing crate that you can explore on crates.io
Hi. I’m Bradlee. I’ve mostly been a lurker in Rust for a while, making a couple small contributions here and there. So launching dtparse feels like nice step towards becoming a functioning member of society. But not too much, because then you know people start asking you to pay bills, and ain’t nobody got time for that.
But I built dtparse, and you can read about my thoughts on the process. Or don’t. I won’t tell you what to do with your life (but you should totally keep reading).
pythonI've been writing some code in Rust recently, and I thought it would be cool if I could take some of this Rust code and provide it as a native extension that I can call from Python. It turns out there are some amazing tools like PyO3 that make it easy to write fully featured Python extensions in Rust, with considerably less effort than writing a CPython extension manually.
To test out PyO3 I wrote a small Python extension in Rust, and I thought I would share some of the tips and tricks I encountered in getting this going. This post aims to serve as a quick tutorial showing how to write extensions in Rust, talking about why you might want to use something more powerful than just exposing a C library called using CFFI, and how PyO3 lets you write Python aware extensions in Rust.
Compressing your files is a good way to save space on your hard drive. At Dropbox’s scale, it’s not just a good idea; it is essential. Even a 1% improvement in compression efficiency can make a huge difference. That’s why we conduct research into lossless compression algorithms that are highly tuned for certain classes of files and storage, like Lepton for jpeg images, and Pied-Piper-esque lossless video encoding. For other file types, Dropbox currently uses the zlib compression format, which saves almost 8% of disk storage.
We introduce DivANS, our latest open-source contribution to compression, in this blog post.
I’ve been working on a project called Thruster recently, and needed a way that a developer could reasonably use templates. Thruster is a middleware based web server written in Rust (get it, th-rust-er? I’m working on my tight 5 for amateur night at The Apollo,) and as such, I needed a way to load HTML templates and insert variables into them in a performant way. Rather than poking around the numerous existing libraries and choosing one made by someone I don’t know — stranger danger! — I decided to make it myself. This article is about that journey, the unbelievably thrilling adventures of writing my first proc_macro_derive in Rust.
This is a post about an interesting testing technique which feels like it should be well known. However, I haven’t seen it mentioned anywhere. I don’t even have a good name for it, I’ve semi-discovered it in the wild. If you know how this thing is called, please leave a comment!
I gave a talk about lyon at RustFest Paris. This post is the introduction of the talk, wherein I introduce vector graphics and try to get the audience somewhat excited about it. Things will get technical in the follow-up posts.
Tarpaulin (or cargo-tarpaulin) is a code coverage tool for Rust. Last year was pretty busy with the launch of the project and the rush of issues as people started to use it so this is just a chance to look at what’s new with version 0.6.0 and what’s planned for the rest of this year.
In this blog post, I would like to present a research project I have been working on: Trying to use QML from Rust, and in general, using a C++ library from Rust. The project is a Rust crate which allows to create QMetaObject at compile time from pure Rust code. It is available here: https://github.com/woboq/qmetaobject-rs
Crates.rs, an alternative opinionated front-end to crates.io:
It’s fast.
All readmes are displayed whenever possible, and if there’s no or poor readme, doc comments are shown too.
It combines information from multiple sources, e.g. byline is a compact amalgamation of Cargo.toml, crates.io, and GitHub contributors, so you get an idea who wrote the code even if authors forgot to keep Cargo.toml up to date.
Crate popularity is displayed as top-N position in its most relevant category, e.g. “#5 in Cryptography”, which is more meaningful than absolute download numbers.
Optional dependencies display which feature or platform they’re for.
Version history is summarized to help see at a glance whether a crate gets regular updates and how often it has breaking changes.
Recognizes sys crates even if they’re not called -sys and shows when build.rs is used.
Category pages fit more crates on screen despite looking less cluttered and having easier to read descriptions.
All categories and their representative crates are right there on the homepage.
Syntax highlighting everywhere, including code blocks.
Everything works without JS and gets indexed by search engines, so I’m hoping the site will help find crates.
While the http crate generally has a great API I have been unsatisfied how it handles URLs. To create a HTTP request a full URL is needed with a scheme (http/https), authority (example.org) and a path (/search?q=rust) but http does enforce this and allows you to only state the path. This means both clients and servers are either unable to determine protocol and and authority information or have to do this manually.
Today sees the release of hyper v0.12.0, a fast and correct HTTP library for the Rust language.
This release adds support for several new features, while taking the opportunity to fix some annoyances, and improve the extreme speeds!
parsersIt took nearly 6 months of development and the library went through nearly 5 entire rewrites. Compare that to previous major releases, which took a month at most to do. But it was worth it! This new release cleans up a lot of old bugs and unintuitive behaviours, simplifies some common patterns, is faster, uses less memory, gives better errors, but the way parsers are written stay the same. It’s like an entirely new engine under the same body work!
async tokioIt took a bit longer than I had initially hoped (as it always does), but a new Tokio version has been released. This release includes, among other features, a new set of APIs that allow performing filesystem operations from an asynchronous context.
gtk testingCurrently, testing UIs is difficult, but with gtk-test you can test basically everything UI-related way more simply.
As happy Rust users ourselves, it makes us even happier to be able to say that we now have a Sentry Rust SDK. This means you and your Fungiculture can now report panics, failures, and other types of incidents to Sentry.
Not only is the SDK new and fancy, it is also one of the first ones that follows our new API guidelines for Sentry SDKs, which makes it even newer and fancier than you might normally expect.
More than a year ago a friend of mine wanted to learn a bit more about Rust by trying out a project. He had a nice project in mind which suits Rust quite well I think. For fun I joined his effort and created an implementation at the same time as he did, discussing and comparing along the way. In this post I’ll tell you about the project specifics, but the point of the post is more an encouragement. If you’ve read about Rust before but haven’t tried it yet, find a small project like the one below, and learn Rust in a fun and hands-on way yourself. It’s a great programming language, I highly recommend it.
gnome gtkLast week, I was working on improving the integration of Rust with GNOME libraries at the third Hackfest, which happened this time in Madrid.
This library implements several of the more commonly useful immutable data structures for Rust. They rely on structural sharing to keep most operations fast without needing to mutate the underlying data store, leading to more predictable code without necessarily sacrificing performance.
tlsMesaLink is a memory-safe and OpenSSL-compatible TLS library. Since 2014, the industry has seen a huge loss due to memory vulnerabilities in TLS stacks, such as the infamous "Heartbleed" bug. MesaLink is created with the goal of eliminating memory vulnerabilities in TLS stacks. MesaLink is written in Rust, a programming language that guarantees memory safety. This significantly reduces the attack surfaces, which facilitates auditing and restricting the remaining attack surfaces. MesaLink is cross-platform and provides OpenSSL-compatible APIs. It works seamlessly in desktop, mobile, and IoT devices. With the growth of the ecosystem, MesaLink would also be adopted in the server environment in the future.
hyperThe newest release of hyper includes some lower-level connection APIs for both the server and client. Notably, this allows using hyper send and receive HTTP upgrade requests. The most popular of these is Websockets.
I am learning Clojure for the past one year and I thought making open source contributions is a great way to interact with the community. I made a post previously on using Clojars metadata to analyse JDK 9 and Clojure 1.9 issues that helped me file issues to ensure compatibility. I used the same method here to find the modules that were broken on a nightly version of a rustc due to a recent stabilisation.
async tokioTo close out a great week, there is a new release of Tokio. This release includes a brand new timer implementation.
I wrote this bit to give a some background about why Rand looks the way it does, and why it is time to make some changes. The new release, Rand 0.5, is getting almost ready fro release. Also I hope it is a bit entertaining to read about the history in combination with early Rust.
async tokioI’m happy to announce a new release of Tokio. This release includes the first iteration of the Tokio Runtime.
asyncOn behalf of the futures-rs team, I’m very happy to announce that the master branch is now at 0.2: we have a release candidate! Barring any surprises, we expect to publish to crates.io in the next week or two.
You can peruse the 0.2 API via the hosted crate docs, or dive right in to the master branch. Note that Tokio is not currently compatible with Futures 0.2; see below for more detail.
error-handlingI’m planning to release a 1.0.0 version of failure on March 15. Once this happens, I don’t plan to release any further breaking changes to the failure crate (though maybe someday in the distant future).
Breaking changes in 1.0 failure is in a somewhat unique position as being a significant part of the public API of other libraries that depend on it. Whether they use the Error struct or derive Fail for a custom error type, this becomes a part of the API they expose to other users.
iui, the Improved User Interface crate, has just gotten its 0.2 release. Improved User Interface is a set of safe, idiomatic Rust bindings to platform native GUI libraries (Win32API, Cocoa, and GTK+) via libui and ui-sys. Highlights of this release include: Correct, semantic use of mutability, GTK+ theme application per window, and menu bars and file open/create modal support.
serialisationBincode is a serializer implementation for Serde. If you stick a #[derive(Deserialize, Serialize)] on your struct, Bincode can efficiently serialize and deserialize those structs to and from bytes. Bincode is unique in that it’s a format that was built specifically for the Rust serialization ecosystem. Tight coupling with Serde allows Bincode to be very fast and serialize to very small payloads.
testingIt’s been a while since I last suggested Mutation Testing in Rust, almost two years ago. Since then I got sidetracked a lot, and later lost interest. Just one more cool project I couldn’t afford to take on. But as things go, my interest in mutation testing was rekindled, and I decided to give it a shot and do more than blogging about it.
For some time now I develop a Rust library for asynchronous programming with coroutines, called Corona (note there’s a version 0.4.0-pre.1, but Crates prefer the „stable“ 0.3.1). I believe it is starting to be useful, so I wrote this description to show what it is good for and how it fits into the big picture of Rust. There’ll be some more changes, though, at least because Tokio just released a new version (and Futures plan to do so soon), so Corona will have to adapt.
sqlAfter a couple of weeks of work, I'm now happy to release the first version of tql, the easy-to-use ORM for Rust. While the focus was to make tql work on the stable version of the compiler, I also added some new features. The most notable new feature is the support for SQLite: now tql supports SQLite as well as PostgreSQL. The support for SQLite is almost as complete as the one for PostgreSQL: the only missing function is not implemented because the backend (SQLite) does not support it.
parsingCombine is a parser combinator library for the Rust programming language. I first announced version 3 of Combine back in August and back then I definitely expected to have a stable version by now. However other projects (cough gluon cough) got in the way and Combine fell to the wayside. It didn’t help that I didn’t have a killer feature for 3.0 either, user-defined error types make it possible to define parsers usable in #[no_std] crates which is great when you need it but it is still a fairly niche use-case.
async tokioI'm happy to announce that today, the changes proposed in the reform RFC have been released to crates.io as tokio 0.1. The primary changes are: Add a default global event loop, eliminating the need for setting up and managing your own event loop in the vast majority of cases, and decouple all task execution functionality from Tokio.
benchmarkingCriterion.rs is a statistics-driven benchmarking library for Rust. It provides precise measurements of changes in the performance of benchmarked code, and gives strong statistical confidence that apparent performance changes are real and not simply noise. Clear output, a simple API and reasonable defaults make it easy to use even for developers without a background in statistics. Unlike the benchmarking harness provided by Rust, Criterion.rs can be used with stable versions of the compiler.
faster began as a yak shave, created to aid base💯 in its quest to become the fastest meme on Github. Writing an explicit AVX2-accelerated version of base💯's encoder and decoder, then realizing I'd have to do the same thing again to see the speedups on my Ivy Bridge desktop, pushed me to make this library. Months later, it has blossomed into its own project, and has eclipsed base💯 in both popularity and promise.
cryptographyA suite of cryptographic libraries and protocol implementations, written in the systems programming language Rust, for creating blazingly-fast, production-quality cryptographic applications.