asyncAsync/await syntax in Rust was initially released to much fanfare and excitement. Recently, the reception has been a bit more mixed. To some extent this is just the natural progression of the hype cycle, but I also think as we have become more distant from the original design process, some of the context has been lost.
Tag: async
Posts 
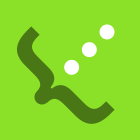
asyncThe Async Foundations Working Group believes Rust can become one of the most popular choices for building distributed systems, ranging from embedded devices to foundational cloud services. Whatever they're using it for, we want all developers to love using Async Rust. For that to happen, we need to move Async Rust beyond the "MVP" state it's in today and make it accessible to everyone.
We are launching a collaborative effort to build a shared vision document for Async Rust. Our goal is to engage the entire community in a collective act of the imagination: how can we make the end-to-end experience of using Async I/O not only a pragmatic choice, but a joyful one?
asyncIn a recent lang team meeting we discussed the upcoming Stream RFC. We covered both the steps required to land it, and the steps we'd want to take after that. One of the things we'd want eventually for streams is: "async iteration syntax". Just like for x in y works for Iterator, we'd want something similar to work for Stream.
mqtt asyncWhen I began this project, many months ago, there were no good resources on Tokio. I procrastinated on writing this blog series, but my intention was for it to be a guide on using Tokio and implementing a real project. A reference of sorts. When I began populating this blog in March, there still weren’t any resources. That is no longer the case. I recently discovered that Tokio added a much better tutorial in June.
This project/tutorial series was my way of learning Rust and Tokio, and I’ve gained a lot out of it. However, the mini-redis tutorial that I linked above seems to cover everything that I would. By the end of this section, we’ll have a client-server architecture that is easily extensible to be a compliant MQTT broker, but I won’t continue the tutorial to create a full-blown MQTT server. If people are interested, I can continue the series, but I don’t see the need for it anymore. So let’s continue from where we left off.
asyncFirst there was cooperative multiprocessing. Then there were processes. An operating system could run multiple processes, each performing a series of sequential, blocking actions. Then came threads. A single processes could spawn off multiple threads, each performing its own series of sequential, blocking actions. (And really, the story starts earlier, with hardware interrupts and the like, but hopefully you'll forgive a little simplification.)
Sitting around and waiting for stuff? Ain't nobody got time for that. Spawning threads at the operating system level? That's too costly for a lot of what we do these days.
Perhaps the first foray into asynchronous programming that really hit the mainstream was the Nginx web server, which boasted a huge increase in throughput by natively using asynchronous I/O system calls. Some programming languages, like Go, Erlang, and Haskell, built runtimes systems that support spawning cheap green threads, and handle the muck of asynchronous system calls for you under the surface. Other languages, such as Javascript and more recently Rust, provide explicit asynchronous support in the language.
Given how everyone seems to be bending over backwards to make it easy for you to make your code async-friendly, it would be fair to assume that all code at all times should be async. And it would also be fair to guess that, if you stick the word async on a function, it's completely asynchronous. Unfortunately, neither of these assumptions are true. This post is intended to dive into this topic, in Rust, using a simple bit of code for motivation.
asyncRust's approach to bringing async code into the language is novel, in that instead of packaging the async system with the language, such as Golang's approach of providing in-built goroutines, it presents an interface to be used by independent crate developers to implement the async runtime for a given process. It also separates the job of the executor or runtime (which polls the futures) and the reactor (which tells the executor when the futures should be polled). Out of this interface, a number of projects have emerged.
async tokioI am pleased to announce the release of async-raft v0.5.0. This release has been a great deal of effort, lots of early mornings and late nights. I am VERY pleased with the quality of the code and how things have come together. The Raft API couldn't be more simple, async/await has absolutely simplified and clarified so many of the interfaces throughout this project, and the many excellent tools available through Tokio have greatly helped with code quality overall.
asyncBefore going on to write a backend for our weather station we first need to familiarize ourselves with a few concepts from the Rust world. If you are unfamiliar with the language take a few minutes to read through Learn Rust in Y minutes to get used to the syntax. When we will write a Telegram bot with Rust, we will use a technique called asynchronous programming. Let’s tackle what that means.
asyncI used to be afraid of async Rust. It's easy to get into trouble!
But thanks to the work done by the whole community, async Rust is getting easier to use every week. One project I think is doing particularly great work in this area is async-std.
async testingOne reason to like the Rust ecosystem is testing. No test runners need to be installed, no reading up on 10 variations of unit testing frameworks, no compatibiltity issues…
… or are there? Rust has recently started supporting full async/await abilities after years of development and it looks like there is a missing piece there: tests. How so?
asyncAsynchronous I/O in Rust is still very much in its infancy. Async/await, Rust’s solution to the problem, was recently stabilized and so when came the time to implement some peer-to-peer networking code, I reached for the shiny new feature. To my dismay, it created more problems than it solved. Indeed, I quickly regretted going down that path and searched for alternatives. All I was looking for was an easy way to handle between fifty and a hundred TCP connections (net::TcpStream) efficiently to implement the reactor for nakamoto, a Bitcoin client I’ve been working on.
asyncThe async/await keywords in modern Rust make building high-throughput daemons pretty straightforward, but as I learned that doesn’t necessarily mean “easy.” Last month on the Scribd tech blog wrote about a daemon named hotdog which we deployed into production: Ingesting production logs with Rust. In this post, I would like to write about some of the technical challenges I encountered getting the performance tuned for this async-std based Rust application.
async redoxThis week has initially been mostly minor bug fixes for the redox_syscall and kernel parts. I began the week by trying to get pcid to properly do all of its scheme logic, which it hasn’t previously done (its IPC is currently, only based on passing command line arguments, or pipes). This meant that the kernel could no longer simply process the syscalls immediately (which I managed to do with non-blocking syscalls such as SYS_OPEN and SYS_CLOSE) by invoking the scheme functions directly from the kernel. So for the FilesUpdate opcode, I then tinkered a bit with the built-in event queues in the kernel, by adding a method to register interest of a context that will block on the event, and by allowing non-blocking polls of the event queues.
asyncSeveral months ago, on May 1st, I spoke to Stjepan Glavina about his (at the time) new crate, smol. Stjepan is, or ought to be, a pretty well-known figure in the Rust universe. He is one of the primary authors of the various crossbeam crates, which provide core parallel building blocks that are both efficient and very ergonomic to use. He was one of the initial designers for the async-std runtime. And so when I read stjepang’s blog post describing a new async runtime smol that he was toying with, I knew I wanted to learn more about it. After all, what could make stjepang say:
> It feels like this is finally it - it’s the big leap I was longing for the whole time! As a writer of async runtimes, I’m excited because this runtime may finally make my job obsolete and allow me to move onto whatever comes next.
If you’d like to find out, then read on!
asyncIn this post we will take a look at how to integrate a Rust web application using warp with RabbitMQ. For this purpose, we will use the lapin library together with deadpool for pooling connections.
The example we will build is pretty simple. There is an endpoint where you can send messages which are then sent to a RabbitMQ instance. At the same time, the service listens to new events coming in, logging them as they are taken from the queue.
async httpI started experimenting with asynchronous Rust code back when futures 0.1 was all we had - before async/await. I was a Rust baby then (I'm at least a toddler now), so I quickly drowned in a sea of .and_then, .map_err and Either<A, B>.
But that's all in the past! I guess!
Now everything is fine, and things go smoothly. For the most part. But even with async/await, there are still some cases where the compiler diagnostics are, just, so much.
There's been serious improvemenst already in terms of diagnostics - the errors aren't as rough as they used to be, but there's still ways to go. Despite that, it's not impossible to go around them and achieve the result you need.
So let's try to do some HTTP requests, get ourselves in trouble, and instead of just "seeing if a different crate would work", get to the bottom of it, and come out the other side slightly more knowledgeable.
linux asyncLast time I wrote about ringbahn, a safe API for using io-uring from Rust. I wrote that I would soon write a series of posts about the mechanism that makes ringbahn work. In the first post in that series, I want to look at the core state machine of ringbahn which makes it memory safe. The key types involved are the Ring and Completion types.
warp tide async httpSince I write a lot of articles about Rust, I tend to get a lot of questions about specific crates: "Amos, what do you think of oauth2-simd? Is it better than openid-sse4? I think the latter has a lot of boilerplate."
And most of the time, I'm not sure what to responds. There's a lot of crates out there. I could probably review one crate a day until I retire!
Well, I recently relaunched my website as a completely custom-made web server on top of tide. And a week later, mostly out of curiosity (but not exclusively), I ported it over to warp.
So these I can review. And let's do so now.
asyncHave you ever looked up into the stars and wondered, “How the is that feature implemented”? In this series, I’ll (hopefully) dive into the implementation of coroutines for several (compiled) programming languages.
A short disclaimer: I’m not too sharp on the details of some (or actually any) of these implementations. Most of this will just be me rambling and looking at compiler source code/the output of the Godbolt Compiler Explorer. I’ll try to validate every claim I’ll post here, but some mistakes are sure to sneak their way into one of these. Feel free to point them up and I’ll fix them as soon as I can.
asyncProgramming languages have different methods of representing asynchronous operations. The way Rust handles concurrency should be familiar if you’ve ever used async/await in JavaScript. The keywords are the same and the fundamental model is similar, except that in Rust, deferred computations are called futures instead of promises. What is not so familiar is that you need to pick a runtime to actually run your asynchronous code.
Rust targets everything from bare-metal, embedded devices to programs running on advanced operating systems and, like C++, focuses on zero-cost abstractions. This impacts what is and isn’t included in the standard library.
Once you have the know-how, you’ll find it’s really not difficult to get started with async in Rust. If you’re writing an asynchronous program in Rust for the first time or just need to use an asynchronous library and don’t know where to start, this guide is for you. I’ll try to get you going as quickly as possible while introducing you to all the essentials you should know.
asyncOneSignal has been using Rust extensively in production since 2016, and a lot has changed in the last four years – both in the wider Rust ecosystem and at OneSignal.
At OneSignal, we use Rust to write several business-critical applications. Our main delivery pipeline is a Rust application called OnePush. We also have several Rust-based Kafka consumers that are used for asynchronous processing of analytics data from our on-device/in-browser SDKs.
Since the last blog post about OnePush, the volume of notifications delivered has increased dramatically. When we published that blog post back in 2016, we were delivering 2 billion notifications per week, and hit a record of 125,000 deliveries per second.
Just this month, we crossed the threshold of sending 7 Billion notifications per day, and hit a record of 1.75 million deliveries per second.
async bookThere is more to a programming language than the language itself: tooling is a key element of the experience of using the language.
The same applies to many other technologies (e.g. RPC frameworks like gRPC or Apache Avro) and it often has a disproportionate impact on the uptake (or the demise) of the technology itself.Tooling should therefore be treated as a first-class concern both when designing and teaching the language itself.
The Rust community has put tooling at the forefront since its early days: it shows.
We are now going to take a brief tour of a set of tools and utilities that are going to be useful in our journey. Some of them are officially supported by the Rust organisation, others are built and maintained by the community.
asyncThis is just a note on getting the best performance out of an async program.
The point of using async IO over blocking IO is that it gives the user program more control over
handling IO, on the premise that the user program can use resources more effectively than the kernel
can. In part, this is because of the inherent cost of context switching between the userspace and
the kernel, but in part it is also because the user program can be written with more specific
understanding of its exact requirements.
There are two main axis on which async IO can gain performance over threads with blocking IO:
* Scheduling time overhead: scheduling tasks in userspace can be substantially faster than
scheduling threads in kernel space when implemented well.
* Stack memory overhead: userspace tasks can use far less memory per task than an OS thread uses
per thread.
async bookZero To Production is a book that I will be writing in the open, publishing one chapter at a time on this blog.
The Rust ecosystem has had a remarkable focus on smashing adoption barriers with amazing material geared towards beginners and newcomers, a relentless effort that goes from documentation to the continuous polishing of the compiler diagnostics. There is value in serving the largest possible audience. At the same time, trying to always speak to everybody can have harmful side-effects: material that would be relevant to intermediate and advanced users but definitely too much too soon for beginners ends up being neglected.
I struggled with it first-hand when I started to play around with async/await. There was a significant gap between the knowledge I needed to be productive and the knowledge I had built reading The Rust Book or working in the Rust numerical ecosystem.
I wanted to get an answer to a straight-forward question: Can Rust be a productive language for API development? Yes. But it can take some time to figure out how. That’s why I am writing this book.
linux asyncIn my previous post, I discussed the new io-uring interface for Linux, and how to create a safe API for using io-uring from Rust. In the time since that post, I have implemented a prototype of such an API. The crate is called ringbahn, and it is intended to enable users to perform IO on io-uring without any risk of memory unsafety.
react asyncThis article covers building a chat app in Rust using asynchronous code.
async tokioIn the last blog of this series, I implemented job queue with tmq. I noted back then that tmq is great if you need to interact with other languages, but may be a little overkill if you are just using rust. I wondered what it'd take to build the job queue with a smaller library footprint, using something like tokio-serde instead of tmq. It was successful, and this blog will step through some of the changes needed.
async tokio async-stdIn this post we will explore a brief example of asynchronous programming in Rust with the Tokio runtime, demonstrating different execution scenarios. This post is aimed at beginners to asynchronous programming.
The source code for this example is available on Github. A branch using the async-std runtime is also available (contributed by @BartMassey).
linux asyncLast fall I was working on a library to make a safe API for driving futures on top of an an io-uring instance. Though I released bindings to liburing called iou, the futures integration, called ostkreuz, was never released. I don’t know if I will pick this work up again in the future but several different people have started writing other libraries with similar goals, so I wanted to write up some notes on what I learned working with io-uring and Rust’s futures model.
async tokioWhat we'll be making: We'll be listening to a port. This port is streaming out some XML events. Only caveat is is that it is first padded (sometimes) with a 32bit number to tell you how much bytes are on its way.
Now, we'll be parsing this stream of events and make it fit into our model. After converting these events into JSON we'll push it onto an Apache Kafka stream. As an added bonus these Kafka messages will be mimicking the messages generated by Spring Cloud Stream.
We'll be using futures and async code for most of the time.
asyncIn about 4 weeks time from the publish date of this blog post, you'll be able to use the async/await feature in no_std code on stable! In this blog post we go over the work we did to make it happen and what we learned from building a proof of concept executor for the ARM Cortex-M architecture.
asyncThe point of the async interview series, in the end, was to help figure out what we should be doing next when it comes to Async I/O. I thought it would be good then to step back and, rather than interviewing someone else, give my opinion on some of the immediate next steps, and a bit about the medium to longer term. I’m also going to talk a bit about what I see as some of the practical challenges.
asyncI have just spent some time doing an initial async version of lorikeet now that the async/await syntax is stable and the ecosystem has caught up. The major blocker was reqwest, as this is used extensively in the http test. This async version is available now as version 0.11.0. You can also install the cli by running cargo install lorikeet.
asyncI’m now building the third async runtime and publishing it very soon. While async-std and tokio are conceptually very similar, the new runtime is outside their boxes and approaches problems from a completely new angle.
But don’t worry, this runtime is not intended to fragment the library ecosystem further - what’s interesting about it is that it doesn’t really need an ecosystem! I’m hoping it will have the opposite effect and bring libraries closely together rather than apart.
asyncTokio is a runtime for asynchronous Rust applications. It allows writing code using async & await syntax. The Rust compiler transforms this code into a state machine. The Tokio runtime executes these state machines, multiplexing many tasks on a handful of threads. Tokio’s scheduler requires that the generated task’s state machine yields control back to the scheduler in order to multiplex tasks. Each .await call is an opportunity to yield back to the scheduler. In the above example, listener.accept().await will return a socket if one is pending. If there are no pending sockets, control is yielded back to the scheduler.
This system works well in most cases. However, when a system comes under load, it is possible for an asynchronous resource to always be ready.
asyncIn this post we explore cooperative multitasking and the async/await feature of Rust. We take a detailed look how async/await works in Rust, including the design of the Future trait, the state machine transformation, and pinning. We then add basic support for async/await to our kernel by creating an asynchronous keyboard task and a basic executor.
asyncMy (mis)adventures with async, tokio, and async-std - wherein I fail with purpose, learning a ton in the process.
asyncLast weekend I released parallel-stream, a data parallelism library for async std. It's to streams, the way rayon is to iterators. This is an implementation of a design I wrote about earlier.
The way parallel-stream works is that instead of calling into_stream to create a sequential stream you can call into_par_stream to create a "parallel" stream instead. This means that each item in the stream will be operated on in a new task, which enables multi-core processing of items backed by a thread pool.
asyncI’ve just released a new crate called waitmap. This is a concurrent hash map (built on top of dashmap) intended for use as a concurrency primitive with async/await. It extends the API of dashmap by having an additional wait method.
asyncHello everyone! I’m happy to be posting a transcript of my async interview with withoutboats. This particularly interview took place way back on January 14th, but the intervening months have been a bit crazy and I didn’t get around to writing it up till now.
async httpI have heard many good things about Rust for several years now. A couple of months ago, I finally decided to start learning Rust. I skimmed through the Book and did the exercises from rustlings. While they helped me get started, I learn best by doing some projects. So I decided to replace the crawler that I used for my Ghost blog, which had been written in bash with wget, with something written in Rust.
And I was pleasantly surprised. I am by no means very knowledgeable in Rust, I still have to look up most of the operations on the Option and Result types, I have to DuckDuckGo how to make HTTP requests, read and write files and so on, but I was still able to write a minimal crawler in about 2-3 hours and then in about 10 hours of total work I had something that was both faster and had fewer bugs than the wget script.
So let's start writing a simple crawler that downloads all the HTML pages from a blog.
async httpToday Friedel Ziegelmayer (Protocol Labs), Ryan Levick (Microsoft), and myself would like to introduce a new set of HTTP libraries to make writing encrypted, async http/1.1 servers and clients easy and quick:
* async-h1 – A streaming HTTP/1.1 client and server protocol implementation.
* http-types – Reusable http types extracted from the HTTP server and client frameworks: Tide and Surf.
* async-native-tls – A streaming TLS client and server implementation.With these libraries writing a streaming, encrypted HTTP client takes about 15 lines of code.
asyncA while ago I realized that I was a visual learner which can be frustrating at times since some concepts might take me a bit longer to fully understand until I create the proper mental image(s) for it (or somebody else does it for me).
When I started wrapping my head around Async programming in Rust I felt like I was missing some of those images. What follows is my attempt visualize the concepts around async programming.
async"Audit" is probably a strong word. Also, take this with a grain of salt. I am by no means an expert with task scheduling. I am, however, interested in using an async RwLock in a production environment.
What I was really interested in is answering the question: If I have a ton of readers acquiring and releasing the lock at all times, do the writers get a chance to acquire the lock, too?
asyncHello! For the latest async interview, I spoke with Eliza Weisman (hawkw, mycoliza on twitter). Eliza first came to my attention as the author of the tracing crate, which is a nifty crate for doing application level tracing. However, she is also a core maintainer of tokio, and she works at Buoyant on the linkerd system. linkerd is one of a small set of large applications that were build using 0.1 futures – i.e., before async-await. This range of experience gives Eliza an interesting “overview” perspective on async-await and Rust more generally.
asyncThis article is not comprehensive on the Rust Async topic but could be an easy overview if you have no idea about Async Programming in Rust or in general. If you are wondering about the new async/await keywords, Futures, and intrigued what Tokio is useful for, then you should feel less clueless by the end.
Rust Async is the new hot thing in Rust’s land. It has been hailed as a big milestone for Rust; especially for people developing highly performant networking applications. The long time for development, the different incompatible versions, and the various libraries; however, might made it not very straightforward to grasp. There is a lot going and it’s not obvious from where to start.
Let’s start from the beginning.
aws asyncRusoto, an AWS SDK for Rust, is now compatible with std::future::Future in a beta release, v0.43.0-beta.1.
This comes shortly after merging Rusoto’s async/.await pull request, which is likely the biggest change to Rusoto since shattering the mega-crate.
If you are using Rusoto today, please help us test this release, file issues if it doesn’t work, and tell us in Discord or email me if it does.
Three separate contributors brought Rusoto forward to modern Rust, and several others made additional contributions to improve the ergonomics of using the SDK. Thanks so much to everybody working on Rusoto.
asyncThere’s been a lot of excitement in the Rust community about the new async and await keywords that dropped on the stable channel last year, but until recently there wasn’t much in the way of documentation or library support. The futures and tokio developers have been working like mad to migrate their own crates over, finally pulling off their own releases in November of 2019. Many library crates using futures have followed suit, and the ecosystem is finally starting to settle into the new way of doing things. This really is a completely different way to express asynchronous code, which means in many cases code must be rewritten or tossed out. So there’s an obvious question for developers: is migrating all your existing code worth the trouble?
The answer is a resounding yes.
asyncFirstly, congratulation on Rust lang achieving stable async/await syntax! As of the release, async/await is becoming the preferred way to do asynchronous programming instead of using Futures in Rust lang.
In Obsidian Web Framework, we do the same move just like other libraries which enabling async/await syntax in order to provide a better development experience.
asyncNow that we’ve built the block_on() function, it’s time to take one step further and turn it into a real executor. We want our executor to run not just one future at a time but many futures concurrently!
This blog post is inspired by juliex, a minimal executor and one of the first that pioneered async/await support in Rust. Today we’re writing a more modern and cleaner version of juliex from scratch.
The goal for our executor is to have only simple and completely safe code while delivering performance that rivals existing best-in-class executors.
asyncWith the new std::future way of doing things and tokio slowly reaching maturation, it's time to look at updating the libraries out there that are using the old ways. For one of my libraries, tmq, a Tokio ZeroMQ library, there is some awesome work already done to get this updated.
But, I thought it pertinent to at least get my feet in the water to see how hard it would be, from a library maintainer perspective, to update to std::future. For this effort, I chose my small library: mpart-async. You can see the changes I have made by comparing the versions here. This blog is a small collection of notes & gotches I found when porting code across.
asyncIf you’ve ever wondered how block_on from the futures crate works, today we are going to write our own version of the function.
Inspiration for this blog post comes from two crates, wakeful and extreme. wakeful has devised a simple way to create a Waker from a function, while extreme is an extremely terse implementation of block_on().
Our implementation will have slightly different goals from extreme. Rather than going for zero dependencies and minimal number of lines of code, we’ll go for a safe and efficient but still pretty simple implementation.
asyncHello! For the latest async interview, I spoke with Steven Fackler (sfackler). sfackler has been involved in Rust for a long time and is a member of the Rust libs team. He is also the author of a lot of crates, most notably tokio-postgres.
asyncNo one ever gets in trouble for posting micro benchmarks and making broad assumptions about the cause of observed results! This post will focus on a couple of such benchmarks pertaining to blocking operations on otherwise asynchronous runtimes. Along the way I’ll give only sparse background on these projects I’ve been working on, but plenty of links if you are interested in reading further. This blog post is sort of a followup to an URLO post: Futures 0.3, async♯await experience snapshot, and I’ll cross-post this one to URLO as well.
asyncHello! For the latest async interview, I spoke with Florian Gilcher (skade). Florian is involved in the async-std project, but he’s also one of the founders of Ferrous Systems, a Rust consulting firm that also does a lot of trainings. In that capacity, he’s been teaching people to use async Rust now since Rust’s 1.0 release.
heroku tokio asyncIn an effort to understand the new Rust async/await syntax, I made a super-simple app that simply responds to all HTTP requests with Hello! and deployed on heroku.
If you want to skip right to the punchline, the source code and README instructions can be found on https://github.com/ultrasaurus/hello-heroku-rust
async tokioI would like to understand how Tokio works. My interests run to the real-time and concurrent side of things but I don't know much about Tokio itself. Before the introduction of async and stable futures I more or less intentionally avoided learning it, not out of any sense that Tokio was wrong but there's only a finite amount of time to learn stuff and it's a rough business to learn a thing that is going to go out of date soonish.
Anyhow. These are my notes for learning Tokio. I don't have a plan of how to learn it's internals, but, generally, I learn best when I have some kind of project to frame my reading around. Context really helps. I don't have a sense of what I want to build long-term, but an HTTP load generator that can scale itself to find the maximum requests per second a server can handle while still satisfying some latency constraint would be pretty neat. This does mean I need to combine my learning with another library – hyper -- but I've used it before and think I can get away with leaving it as a black-box.
async httpreqwest is a higher-level HTTP client for Rust. Let me introduce you the v0.10 release that adds async/await support!
async haskellGHC Haskell supports a feature called asynchronous (or async) exceptions. Normal, synchronous exceptions are generated by the currently running code from doing something like trying to read a file that doesn't exist. Asynchronous exceptions are generated from a different thread of execution, either another Haskell green thread, or the runtime system itself.
Rust does not have exceptions at all, much less async exceptions. (Yes, panics behave fairly similarly to synchronous exceptions, but we'll ignore those in this context. They aren't relevant.) Rust also doesn't have a green thread-based runtime like Haskell does. There's basically no direct way to compare this async exception concept from Haskell into Rust.
Or, at least, there wasn't. With Tokio, async/.await, executor, tasks, and futures, the story is quite different. A Haskell green thread looks quite a bit like a Rust task. Suddenly there's a timeout function in Tokio. This post is going to compare the Haskell async exception mechanism to whatever powers Tokio's timeout. It's going to look at various trade-offs of the two different approaches. And I'll end with my own personal analysis.
asyncThe Rust async story is extremely good and many people (like me) have already converted their networked applications to async. In this post, I document some async/await pitfalls and show you how to avoid them. Finally, how to debug a async program.
asyncHello! For the latest async interview, I spoke with Carl Lerche (carllerche). Among many other crates1, Carl is perhaps best known as one of the key authors behind tokio and mio. These two crates are quite widely used through the async ecosystem. Carl and I spoke on December 3rd.
asyncIn our last post about Async Rust we looked at Futures concurrency, and before that we looked at Rust streams. In this post we bring the two together, and will take a closer look at concurrency with Rust streams.
async tokioThe release of Tokio 0.2 was the culmination of a great deal of hard work from numerous contributors, and has brought several significant improvements to Tokio. Using std::future and async/await makes writing async code using Tokio much more ergonomic, and a new scheduler implementation makes Tokio 0.2’s thread pool as much as 10x faster. However, updating existing Tokio 0.1 projects to use 0.2 and std::future poses some new challenges. Therefore, we’re very excited to announce the release of the tokio-compat crate to help ease this transition, by providing a runtime compatible with both Tokio 0.1 and Tokio 0.2 futures.
asyncMio 0.7 is the work of various contributors over the course of roughly half a year. Compared to Mio version 0.6, version 0.7 reduces the size of the provided API in an attempt to simplify the implementation and usage. The API version 0.7 will be close to the proposed API for a future version 1.0. The scope of the crate was reduced to providing a cross-platform event notification mechanism and commonly used types such as cross-thread poll waking and non-blocking networking I/O primitives.
asyncThis blog post is continuing my conversation with cramertj. This will be the last post.
In the first post, I covered what we said about Fuchsia, interoperability, and the organization of the futures crate.
In the second post, I covered cramertj’s take on the Stream, AsyncRead, and AsyncWrite traits. We also discused the idea of attached streams and the imporance of GATs for modeling those.
In this post, we’ll talk about async closures
asyncThis blog post is continuing my conversation with cramertj.
In the first post, I covered what we said about Fuchsia, interoperability, and the organization of the futures crate. This post covers cramertj’s take on the Stream trait as well as the AsyncRead and AsyncWrite traits.
asyncFor the second async interview, I spoke with Taylor Cramer – or cramertj, as I’ll refer to him. cramertj is a member of the compiler and lang teams and was – until recently – working on Fuchsia at Google. They’ve been a key player in Rust’s Async I/O design and in the discussions around it. They were also responsible for a lot of the implementation work to make async fn a reality.
asyncHi everyone, I haven’t blogged in a while so it feels good to be back. First things first — here’s some quick news. After two years of work on Crossbeam, in 2019 I’ve shifted my main focus onto asynchronous programming to research the craft of building runtimes (think of async-std and tokio). In particular, I want to make async runtimes more efficient and robust, while at the same time also simpler.
In this blog post, I’d like to talk a bit about an interesting problem all runtimes are facing: calling blocking functions from async code.
async tokio tutorialIn the previous lesson in the crash course, we covered the new async/.await syntax stabilized in Rust 1.39, and the Future trait which lives underneath it. This information greatly supercedes the now-defunct lesson 7 from last year, which covered the older Future approach.
Now it’s time to update the second half of lesson 7, and teach the hot-off-the-presses Tokio 0.2 release.
asyncIt’s about a year since I wrote the last installment in the Rust Crash Course series. That last post was a doozy, diving into async, futures, and tokio. All in one post. That was a bit sadistic, and I’m a bit proud of myself on that front.
Much has happened since then, however. Importantly: the Future trait has moved into the standard library itself and absorbed a few modifications. And then to tie that up in a nicer bow, there’s a new async/.await syntax. It’s hard for me to overstate just how big a quality of life difference this is when writing asynchronous code in Rust.
asyncA few years back, I wrote up a detailed blog post on Docker's process 1, orphans, zombies, and signal handling. The solution from three years ago was a Haskell executable providing this functionality and a Docker image based on Ubuntu.
A few of the Haskellers on the FP Complete team have batted around the idea of rewriting pid1 in Rust as an educational exercise, and to have a nice comparison with Haskell. No one got around to it. However, when Rust 1.39 came out with async/await support, I was looking for a good use case to demonstrate, and decided I'd do this with pid1.
asyncHello from Iceland! (I’m on vacation.) I’ve just uploaded the first
of the Async Interviews to YouTube. It is a conversation with Alex
Crichton (alexcrichton) and Nick Fitzgerald (fitzgen) about how
WebAssembly and Rust’s Async I/O system interact.
asyncLately, I’ve been seeing some common misconceptions about how Rust’s futures and async/await work (“blockers”, haha). There’s an influx of new users excited for the major improvements that async/await brings, but stymied by basic questions. Concurrency is hard, even with async/await. Documentation is still being fleshed out, and the interaction between blocking/non-blocking can be tricky. Hopefully this article will help.
asyncHello all! I’m going to be trying something new, which I call the “Async Interviews”. These interviews are going to be a series of recorded video calls with various “luminaries” from Rust’s Async I/O effort. In each one, I’m going to be asking roughly the same question: Now that the async-await MVP is stable, what should we be doing next? After each call, I’ll post the recording from the interview, along with a blog post that leaves a brief summary.
async tutorialIn which we explore Rust's newly stabilized async/.await language feature by creating a simple, asynchronous application. We look at what you need to do asynchronous programming in Rust and how it differs from other languages. And we talk a little bit about Pokémon!
asyncOne of the big sources of difficulty on the async ecosystem is spawning tasks. Because there is no API in std for spawning tasks, library authors who want their library to spawn tasks have to depend on one of the multiple executors in the ecosystem to spawn a task, coupling the library to that executor in undesirable ways.
Ideally, many of these library authors would not need to spawn tasks at all.
async tutorial bookThis book is targeted towards experienced programmers that already feel somewhat comfortable with vanilla Rust (you definitely do not need to be an "expert" though, I certainly am not) and would like to dip their toes into its async ecosystem.
As the title indicates, this is not so much a book about how to use async Rust as much as it is about trying to build a solid understanding of how it all works under the hood. From there, efficient usage should come naturally.
asyncasync-std is a port of Rust’s standard library to the async world. It comes with a fast runtime and is a pleasure to use. We’re happy to finally announce async-std 1.0. As promised in our first announcement blog post, the stable release coincides with the release of Rust 1.39, the release adding async/.await. We would like to thank the active community around async-std for helping get the release through the door.
asyncOn Thursday, November 7, async-await syntax hit stable Rust, as part of the 1.39.0 release. This work has been a long time in development -- the key ideas for zero-cost futures, for example, were first proposed by Aaron Turon and Alex Crichton in 2016! -- and we are very proud of the end result. We believe that Async I/O is going to be an increasingly important part of Rust's story.
asyncAfter reading boat’s excellent post on asynchronous destructors, I thought it might be a good idea to write some about async fn in traits. Support for async fn in traits is probably the single most common feature request that I hear about. It’s also one of the more complex topics. So I thought it’d be nice to do a blog post kind of giving the “lay of the land” on that feature – what makes it complicated? What questions remain open?
async tokioWe’ve been hard at work on the next major revision of Tokio, Rust’s asynchronous runtime. Today, a complete rewrite of the scheduler has been submitted as a pull request. The result is huge performance and latency improvements. Some benchmarks saw a 10x speed up! It is always unclear how much these kinds of improvements impact “full stack” use cases, so we’ve also tested how these scheduler improvements impacted use cases like Hyper and Tonic (spoiler: it’s really good).
asyncAfter originally researching the history and discussions about Rusts Async story, I realized I needed a better understanding of async basics and the result is this book. It's published it as a gitbook to make this journey easier for the next person (hopefully).
asyncAs you've perhaps heard, recently the async-await feature landed on the Rust beta branch. This marks a big turning point in the usability story for Async Rust. But there's still a lot of work to do. As we mentioned in the main post, the focus for the Async Foundations WG in the immediate term is going to be polish, polish and (ahem) more polish.
In particular, we want to take aim at a backlog of strange diagnostics, suboptimal performance, and the occasional inexplicable type-check failure. This is a shift: whereas before, we could have laser focus on things that truly blocked stabilization, we've now got a large set of bugs, often without a clear prioritization between them. This requires us to mix up how the Async Foundations WG is operating.
asyncBig news! As of this writing, syntactic support for async-await is available in the Rust beta channel! It will be available in the 1.39 release, which is expected to be released on November 7th, 2019. Once async-await hits stable, that will mark the culmination of a multi-year effort to enable efficient and ergonomic asynchronous I/O in Rust. It will not, however, mark the end of the road: there is still more work to do, both in terms of polish (some of the error messages we get today are, um, not great) and in terms of feature set (async fn in traits, anyone?).
asyncSometimes, I get this nudging feeling that something is not exactly right and that I have to go out and save the world and fix it (even though it’s usually something minor or doesn’t need fixing at all). I guess everyone has days like these. It’s part what drives me to invest my free time to writing software.
This is about some dead ends when trying to fix the problem of Rust’s async networking fragmentation. I haven’t been successful, but I can at least share what I tried and discovered, maybe someone else is having the same bugging feeling so they don’t have to repeat them. Or just maybe some of the approaches would work for some other problems. And because we have a bunch of success stories out there, having some failure stories to balance it doesn’t hurt.
asyncLast month we introduced Surf, an async cross-platform streaming HTTP client for Rust. It was met with a great reception, and people generally seem to be really enjoying it. A common piece of feedback we've gotten is how much people enjoy the interface, in particular how little code it requires to create HTTP requests. In this post we'll cover a pattern at the heart of Surf's ergonomics stjepang came up with: the "async finalizer".
asyncIn a previous post we've looked at Rust streams. In this post we're going to discuss another problem in the async space: futures concurrency combinators. We're going to cover the different forms of concurrency that can be expressed with Futures, and cover both fallible and infallible variants.
asyncIn Part 1, we covered how async fns in Rust are compiled to state machines. We saw that the internal compiler implementation uses generators and the yield statement to facilitate this transformation. In this post, we'll go over some subtleties that the compiler implementation must consider when optimizing generators. We'll look at two different kinds of analysis, liveness analysis and storage conflict detection.
asyncOne neat result of Rust’s futures and async/await design is that all of the async callers are on the stack below the async callees. In most other languages, only the youngest async callee is on the stack, and none of the async callers. Because the youngest frame is most often not where a bug’s root cause lies, this extra context makes debugging async code easier in Rust.
asyncWe are excited to announce a beta release of async-std with the intent to publish version 1.0 by September 26th, 2019. async-std is a library that looks and feels like the Rust standard library, except everything in it is made to work with async/await exactly as you would expect it to. The library comes with a book and polished API documentation, and will soon provide a stable interface to base your async libraries and applications on. While we don't promise API stability before our 1.0 release, we also don't expect to make any breaking changes.
async httpToday we're happy to announce Surf, an asynchronous cross-platform streaming HTTP client for Rust. This project was a collaboration between Kat Marchán (Entropic / Microsoft), Stjepan Glavina (Ferrous Systems), and myself (Yoshua Wuyts). Surf is a friendly HTTP client built for casual Rustaceans and veterans alike.
async tokioWe’re pleased to announce the release of the first Tokio alpha with async & await support. This includes updating all of the Tokio crates to use std::future instead of futures 0.1. It also includes adding async fn versions of the APIs.
asyncIf you are familiar with Python ecosystem, probably you had heard about psutil package — a cross-platform library for retrieving information about system processes and system utilization (CPU, memory, disks, network and so on). It is very popular and actively used package, which has analogs in other languages: gopsutil for Golang, oshi for Java, you name it. Rust, of course, is not an exception here: we do have psutil, sysinfo, sys-info and systemstat crates.
Now, despite the tremendous work that had been done already by the authors of these crates, I’m excited to announce what I’ve been working on for the past three months: “heim” project — library for system information fetching.
asyncI recently migrated a small/medium-sized crate from Futures 0.1 to 0.3. It was fairly easy, but there were some tricky bits and some things that were not well documented, so I think it is worth me writing up my experience.
async tokioIt took a bit longer than I had initially hoped (as it always does), but a new Tokio version has been released. This release includes, among other features, a new set of APIs that allow performing filesystem operations from an asynchronous context.
ownership-and-borrowing asyncThe networking working group is pushing hard on async/await notation for Rust, and @withoutboats in particular wrote a fantastic blog series working through the design space. I wanted to talk a little bit about some of the implications of async/await, which may not have been entirely clear. In particular, async/await is not just about avoiding combinators; it completely changes the game for borrowing.
async tokioTo close out a great week, there is a new release of Tokio. This release includes a brand new timer implementation.
async tokioI’m happy to announce a new release of Tokio. This release includes the first iteration of the Tokio Runtime.
asyncOn behalf of the futures-rs team, I’m very happy to announce that the master branch is now at 0.2: we have a release candidate! Barring any surprises, we expect to publish to crates.io in the next week or two.
You can peruse the 0.2 API via the hosted crate docs, or dive right in to the master branch. Note that Tokio is not currently compatible with Futures 0.2; see below for more detail.
async tokioI'm happy to announce that today, the changes proposed in the reform RFC have been released to crates.io as tokio 0.1. The primary changes are: Add a default global event loop, eliminating the need for setting up and managing your own event loop in the vast majority of cases, and decouple all task execution functionality from Tokio.