testingNextest is faster than cargo test for most Rust projects, and provides a number of extra features, such as test retries, reusing builds, and partitioning (sharding) test runs. To power these features, nextest uses Tokio, the leading Rust async runtime for writing network applications.
Tag: testing
Posts 
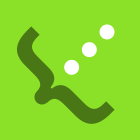
testingHTTP mocking libraries allow you to simulate HTTP responses so you can easier test code that depends on third-party APIs. This article shows how you can use httpmock to do this in Rust.
testingMaxime Chevalier-Boisvert requested resources for learning about fuzzing programming language implementations on Twitter:
> I’d like to learn about fuzzing, specifically fuzzing programming language
> implementations. Do you have reading materials you would recommend, blog
> posts, papers, books or even recorded talks?
Maxime received many replies linking to informative papers, blog posts, and lectures. John Regehr suggested writing a simple generative fuzzer for the programming language.
testing property-testingSoftware testing is an industry-standard practice, but testing methodologies and techniques vary dramatically in their practicality and effectiveness.
Today you’ll learn about property-based testing (PBT), including how it works, when it makes sense, and how to do it in Rust.
async testingOne reason to like the Rust ecosystem is testing. No test runners need to be installed, no reading up on 10 variations of unit testing frameworks, no compatibiltity issues…
… or are there? Rust has recently started supporting full async/await abilities after years of development and it looks like there is a missing piece there: tests. How so?
testingIn this post, we will discuss an interesting technique for testing test coverage, and the associated Rust crate — cov-mark. The two goals of the post are:
1. Share the knowledge about a specific testing approach.
2. Show a couple of Rust tricks for writing libraries.This post is an independent sequel to A Trick for Test Maintenance one.
testingWhenever you write any kind of code, it’s critical to put it to the test. In this guide, we’ll walk you through how to test Rust code.
But before we get to that, I want to explain why it’s so important to test. To put it plainly, code has bugs. This unfortunate truth was uncovered by the earliest programmers and it continues to vex programmers to this day. Our only hope is to find and fix the bugs before we bring our code to production.
Testing is a cheap and easy way to find bugs. The great thing about unit tests is that they are inexpensive to set up and can be rerun at a modest cost.
Think of testing as a game of hide-and-seek on a playground after dark. You could bring a flashlight, which is highly portable and durable but only illuminates a small area at any given time. You could even combine it with a motor to rotate the light to reveal more, random spots. Or, you could bring a large industrial lamp, which would be heavy to lug around, difficult to set up, and more temporary, but it would light up half the playground on its own. Even so, there would still be some dark corners.
testingKaos is a chaotic testing harness to test your services against random failures. It allows you to add points to your code to crash sporadically and harness asserts availability and fault tolerance of your services by seeking minimum time between failures, fail points, and randomized runs.
Kaos is equivalent of Chaos Monkey for the Rust ecosystem. But it is more smart to find the closest MTBF based on previous runs. This is dependable system practice. For more information please visit Chaos engineering.
testingfaux is a traitless Rust mocking framework for creating mock objects out of user-defined structs. faux uses attributes to transform your structs into mockable versions of themselves at compile time.
testingOften when writing automated tests for parts of distributed systems such as a microservice, one runs into the problem of the service under test calling external web services.
In this post, we’ll look at the mockito library, which provides a way for mocking such requests in tests, making writing those tests a lot more convenient.
The library is being actively developed. Currently, it’s possible to match requests on method, path, query, headers and the body, with the possibility to combine these matchers as well.
testingLuckily getting starting with testing Rust code is reasonably straightforward and no external libraries are needed. cargo test will run all test code in a Rust project. Test code is identified with the code attributes #[cfg(test)] and #[test].
testingMock objects are test versions of objects that contain fake implementations of their behavior. For example, if your code accesses your file system, makes network requests, or performs other expensive actions, it is often useful to mock that behavior. Mocking can help make your tests into true unit tests that run quickly and produce the same result every time without relying on external dependencies. For a deeper dive into mocks, read this post.
testingWe’re proud to announce mockiato! For the last few months, we tackled the issue of creating a usable mocking library. Our primary goals were: Ease of use: The mocks are written in idiomatic Rust and don’t rely on custom macro syntax. Maintainability: The entire code base strives to follow the rules of Clean Code and Clean Architecture as specified by Robert C. Martin. Strict expectation enforcement: Mockiato catches unexpected behavior as soon as it happens instead of returning default values.
gtk testingCurrently, testing UIs is difficult, but with gtk-test you can test basically everything UI-related way more simply.
testingIt’s been a while since I last suggested Mutation Testing in Rust, almost two years ago. Since then I got sidetracked a lot, and later lost interest. Just one more cool project I couldn’t afford to take on. But as things go, my interest in mutation testing was rekindled, and I decided to give it a shot and do more than blogging about it.