vulkanOver the course of the last decade, Rust has emerged as a new programming language for writing safe low-level code. I've been contemplating the idea of using it in Mesa for a few years now. Specifically, I'd like to know if it's practical to write a Vulkan driver mostly in Rust and if doing so would bring enough benefit to be worth the effort. This blog post is intended to be the first in a series exploring the area of using Rust to write Mesa Vulkan drivers.
Games and Graphics
Games built with Rust and other graphics related work. Posts 
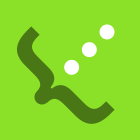
I don’t recall the first time I found out about Halite. It was probably years ago. I do remember thinking a lot about the idea of writing your own bot that plays the game and hopefully wins. I thought about doing it in Python for a week or so, but then I gave up and forgot about it.
Fast forward a few years, and here I am rediscovering Halite again. This time it was much more entertaining to play with. I combined it with my enthusiasm for Rust and spent a couple of days writing what I like to call a BDK, bot development kit.
amethystCourtesy of @jazarro we proudly announce our 5th showcase game, Dwarf Seeks Fortune! It's a 2D puzzle platformer, and an homage to the 1988 classic King's Valley II.
Recently I embarked on a fun little side project. There have been just too many rampant fireworks going on outside and I was feeling a little left out, so I decided to make some of my own.
Given I have no experience with anything like OpenGL at all, and was feeling like learning termion since I consistently use tui with a termion backend and only sort of understand how it works, I decided terminal fireworks would be the way to go.
data-vizIn this section, we're going to use 120 years of Olympic history to create two visualisations. Let's set our sights on something that illustrates the age and height in athletes grouped by the different Olympic games.
Rust is an increasingly popular programming language, and one of the things that people really like to program is games. As such, there are many available libraries for implementing game functionality. The APIs come in many flavors. I have tried many over the last couple years, but Piston, which was one of the first I came across, remains my favorite.
The content covered here builds on the code explored in Creating a Simple Spritesheet Animation with Amethyst. This part will be extending the previous implementation for an “idle” sprite animation by adding a “running” animation in response to user input. In the previous post, we implemented an AnimationSystem responsible for telling the game what sprite image to draw to the screen during each iteration of the game loop.
Now we will be introducing another system called MovePlayerSystem, which will implement the logic for moving our sprite image on the screen based on user input.
Today, we are happy to announce the release of 0.6 versions across gfx/wgpu projects!
A few days ago I just posted this link internally. I, like everyone else, needed a few days to properly review Bevy and get a better feel for what it brings to the table.
To put it simply, Bevy is essentially a Amethyst Engine 2.0. That can sound intimidating since it’s a separate project under different leadership, but I’m convinced this will turn out to be a Good Thing for the entire Rust gamedev community, including the Amethyst project.
Bevy has enjoyed some pretty wild success in the week since its launch. Here are my thoughts on how we can run the Bevy project at its newfound scale.
Here here! Announcing a fresh release for you! We are happy to announce 0.15.1 which will be our last engine version using specs! All aboard the Amethyst 0.16 Legion hype train!
Ever since finding OpenCV Rust bindings I’ve been looking for a good project to try it out. Than a friend sparked my curiosity when talking about gesture recognition project he was implementing.
This inspired me to create a tool which can read frames from the camera, track eye movements and translate them to mouse cursor movements. I reckoned Rust is a good fit for writing performant, numerical code. First step would be to implement an algorithm responsible for tracking the location of eye center in a frame (the pupil), and this blog post is dedicated to describing one possible approach.
I started researching the subject and quickly found that the current state-of-the art is described in Timm and Barth, 2011 - I highly recommend reading the paper. A bit more searching revealed that trishume did all the hard work of implementing the algorithm in C++ using OpenCV. Armed with this reference implementation and the original paper I could easily port it to Rust.
After months of work, I am ecstatic to finally announce Bevy Engine!
Bevy is a refreshingly simple data-driven game engine and app framework built in Rust. It is free and open-source forever!
It has the following design goals:
* Capable: Offer a complete 2D and 3D feature set
* Simple: Easy for newbies to pick up, but infinitely flexible for power users
* Data Focused: Data-oriented architecture using the Entity Component System paradigm
* Modular: Use only what you need. Replace what you don't like
* Fast: App logic should run quickly, and when possible, in parallel
* Productive: Changes should compile quickly ... waiting isn't funBevy has a number of features that I think set it apart from other engines:
* Bevy ECS: A custom Entity Component System with unrivaled usability and blisteringly-fast performance
* Render Graphs: Easily build your own multi-threaded render pipelines using Render Graph nodes
* Bevy UI: A custom ECS-driven UI framework built specifically for Bevy
* Productive Compile Times: Expect changes to compile in ~0.8-3.0 seconds with the "fast compiles" config
NES was one of the most popular gaming platforms through the 80ies and the 90ies. The platform and the emergent ecosystem was and still is a huge cultural phenomenon. The device itself had relatively simple hardware (judging from the modern days), and it's incredible how much was made out of it.
This series is about creating an emulator capable of running and playing first-gen NES games.
We would go with incremental updates, with potentially enjoyable milestones, gradually building a fully capable platform. One of the problems in writing an emulator is that you can't get any feedback until the end. Until the whole thing is done, and that's no fun. I've tried to break the entire exercise into small pieces with visible and playable goals. After all, it's all about having a good time.
A really powerful tool in Computer Aided Design (CAD) is the ability to apply “constraints” to your drawing. Constraints are a really powerful tool, allowing the drafter to declare how parts of their drawing are related, then letting the CAD program figure out how parameters can be manipulated in such a way that you can think of a constraint as some sort of mathematical relationship between two or more parameters.
Welcome to the twelfth issue of the Rust GameDev Workgroup’s monthly newsletter.
iosHere's a guide to setting up an iOS project using Rust and SDL2.
I must admit Rust has provided a pleasant journey so far. I remember one occasion where the borrow checker actually saved me from another session of empty-screen-debugging.
Hi. In the previous part, we harnessed randomness to generate boids and added a rule for obstacle avoidance. We'll be completing our experiment in this part by covering the following:
1. Adding rules for flock behaviour
2. Using parallelism to boost performance
3. Bench-marking performance
Welcome to another pointless tangent into the exciting world of line joints in embedded-graphics! embedded-graphics is an integer only, iterator based no-std graphics library for environments with low resource availability. This time, we’ll be looking at some not-so-great optimisations made to a point sorting function.
Hello again. In part 1, we began our ambitious experiment of simulating a school of fish. We set up a scene and added some basic animation for a moving cone. Those were baby steps. You can download the project at this stage from this tagged version.
debuggingwgpu is a native WebGPU implementation in Rust, developed by gfx-rs community with help of Mozilla. It’s still an emerging technology, and it has many users:
* Gecko and Servo - for implementing WebGPU in the browsers
* wgpu-rs - for using idiomatically from Rust
* wgpu-native - for using from C language and others binding to C (Scopes, Python, even Julia), striving for C header compatibility with Dawn via the shared webgpu-headers.Given the diversity of platforms and configurations it runs, and the variety of users, the questions of reproducing issues, debugging, and testing the implementation were critical to resolve.
Ludum Dare, the world's premiere 48 hour solo gamejam, occurred back in April.
I've entered the Compo a few times using Unity, but this time around I wanted to write a game purely with the relatively new programming language Rust.
This post started out focused on the experience of using Rust, but turned into a general overview of the technical and design process for the game.
Rust Sokoban is an extended tutorial on making a Sokoban copy in Rust. We'll use an existing 2D game engine, pre-made assets and by the end we'll have a fully working game.
In this three part blog series, we will simulate a group of virtual agents (boids) that will swim around an enclosed space behaving like a school of fish. This is a beautiful application of procedural graphics generation, where simple rules create complex patterns. It is almost entirely inspired by Sebastian Lague's Coding Adventure with boids.
This Month in #RustLang #GameDev #11 - June 2020! Includes updates about:
• Legion jam
• Veloren
• CrateAttack
• Way of Rhea
• A/B Street
• Garden
• Mun Lang
• rust-psp
• NodeFX
• macroquad
• Göld
• Tetra
I recently wrote my first 4K intro in Rust and released it at the Nova 2020 where it took first place in the new school intro competition. Writing a 4K intro is quite involved and requires you to master many different areas at the same time. Here I will focus on what I learned about making Rust code as small as possible.
This guide is for people who are interested using Rust with the Godot game engine, but found the API docs to be too abstract to follow along, and the existing tutorials too shallow to accomplish much.
Efficient rendering is complicated. In order to start learning the technology in a hands on way, and guarantee certain functionality, my strategy is going to be to start working from functional code. In this post I’ll break down my process of understanding some existing tutorial code, packaging up its elements in a way I can build on, and extending some of its static aspects to meet the needs of the first Rust render of the static scene from the post Fast Spaceship Physics. I’ll be using the basics of Rust, such as can be gleaned from the first few chapters of the exceptional Rust book, with ad hoc elements courtesy of Google.
wasmPont is an online implementation of Qwirkle, a board game by Mindware Games. It was written for my parents, so they could play with friends and family during the COVID-19 stay-at-home era.
Play is split into rooms, which are identified by a three-word code (size moody shape in the image above). Within each room, the game distributes pieces, enforces the game rules, and provides a local chat window.
Unusually, it's a web-based multiplayer game without any Javascript: both the client and server are written in Rust, which is compiled into WebAssembly to run on the browser. (There's a Javascript shim to load the WebAssembly module, but I didn't have to write it myself)
Starting from zero poses a unique challenge. We can strike out in any direction, and try any technique or technology. This can be dangerous. Unguided by experience, we are free to invest in techniques or technologies that will not ultimately prove helpful. Conveniently, in these enlightened days, experience is easily consulted. By identifying some of the biggest choices to be made in starting a game project and seeking out guidance, we can research a reasonable path to start out on. This post will talk about why I’ve chosen Rust, how the development environment and rendering technology were chosen, and walk through the initial installation of that environment.
lispGameLisp is a scripting language for Rust game development. It was created while working on The Castle on Fire.
Includes updates about:
* Veloren
* Sailing Simulator Prototype
* Orb Farm
* Crate Before Attack
* Garden
* Mun
* rg3d
* NodeFX
* Oxygengine
* TetraAnd lot's of other news!
This post is an update to 2D Graphics on Modern GPU, just over a year ago. How time flies!
I am currently learning Vulkan using gfx-hal. It's quite complex, but fun actually!!!
Recently I built a very minimal full fledged working example using gfx-hal and got to learn many things. So thought to share my learnings with everybody out there.
I mashed together my interests in procedural graphics generation and Rust programming for this experiment. I implemented the boids flocking algorithm to create swirling patterns. The implementation is heavily inspired by Sebastian Lague's coding adventure with boids video.
This post is about a Rust program…
$ cargo install conway-nes
…that prints out a NES binary…
$ conway-nes > life.nes
…that runs Conway’s Game of Life!
fontsWhile developing Robo Instructus I spent an inordinate amount of time messing about with text rendering. After releasing the game last year, I’d thought my time diving into the glyph filled rabbit hole of text would be over… This was not to be, my text-retirement came to an end with a thought: How hard can it be to get otf fonts working?
As you may know, and if you're reading this chances are you do, there's this post that's been roaming around for 7 years and periodically resurfaces. It refers to a code golfing challenge dating back in 1984, a few years before I was born. Its rules asked to build a raytracer in C with certain features and functions and shading models. Among those, Andrew Kensler's version, checking in at 1337 bytes, stood out and became world-wide renowned (for good reasons, if you ask me).
wgpuWebGPU is a new graphics and compute API designed on the grounds of W3C organization (mostly) by the browser vendors. It’s designed for the Web, used by JavaScript and WASM applications, and driven by the shared principles of Web APIs. It doesn’t have to be only for the Web though. In this post, I want to share the vision of why WebGPU on native platforms is important to me. This is highly subjective and doesn’t represent any organization I’m in.
Efficiently synchronizing a client application with the server can be challenging. Today, I write about my recent experience on this topic and the benefits I found when using Rust for both the server and the client endpoints.
Everything in this post is based on my long-term hobby project called Paddlers, an online multiplayer game playable in the browser.
waylandWhen the Rust programming language was bright and shiny and new, one of the more interesting/popular projects for it was Way Cooler, a Wayland compositor in Rust. Wayland is Linux’s next-gen API to replace X11 for graphics and user interaction, so this was pretty cool, two next-gen technologies playing nice together. So it was a little bit of an “oof” moment when Way Cooler announced it was giving up on Rust to rewrite in C instead.
It left me with some questions though, mainly, what IS it like to write a Wayland compositor in Rust? How safe can you make it, or not make it? So now, a couple years later, I’ve taken a bit of time and translated the wlroots example code from C to Rust. Here are my findings.
Welcome to the ninth issue of the Rust GameDev Workgroup’s monthly newsletter.
wgpugfx-rs is a Rust project aiming to make low-level GPU programming portable with low overhead. It’s a single Vulkan-like Rust API with multiple backends that implement it: Direct3D 12/11, Metal, Vulkan, and even OpenGL.
wgpu-rs is a Rust project on top of gfx-rs that provides safety, accessibility, and even stronger portability.
This is Part 3 of a series of tutorials on low-level graphics programming in Rust using gfx-hal.
In Part 2 of the series, we learned how to use push constants to change the position, color, and size of the triangles we were drawing.
Push constants open up a lot of possibilities - but at the end of the day, just drawing individual triangles can only be so much fun. That’s why in this part, we’re going to draw an actual 3D model. Which 3D model? Why, the Utah teapot of course!
We’re going to update our shaders to use vertex attributes, create a vertex buffer full of teapot data, and finally update our pipeline to connect them together.
This Month in #RustLang #GameDev #8 - March 2020!
This is Part 2 of a series of tutorials on graphics programming in Rust using gfx-hal. In Part 1 of the series, we embarked on a grand expedition to draw a single triangle. It was a tremendously exciting example - but not the most flexible one.
That’s why in this part, we want to be able to draw more interesting triangles. To do that, we’ll have to supply some inputs to the shaders - specifically things like color, position, and scale. This means we’ll need a way to send some data from our CPU-side app over to the GPU.
This is Part 1 of a series of tutorials on graphics programming in Rust, using gfx-hal.
If you’re reading this, I assume you want to learn gfx-hal. I will also callously assume you know a little OpenGL, or something similar, and have at least a rough idea of how shaders work. At least, this was where I was before I wrote these tutorials - so hopefully if you fit that category, you’ll find them useful.
The very simplest thing I can think to do with a graphics API is to draw a single triangle. You might expect that to be quick and easy. Not so! Like Vulkan (the API it is based on), gfx requires a lot of set up to render anything at all. I’ve tried to make the code concise, but the fact remains that Part 1 of this series will likely be the longest and most complex entry.
I would encourage you to stick with it though! Once you have this foundation to build on, everything after will come more easily.
ecsFull disclosure, I worked on specs. I’m biased. Specs has evolved quite a bit since I last worked on it years ago, there are some very talented developers who were willing to put in the hard work to improve it. Today it is a better library than it was when I stopped working on it. The developers have done a wonderful job of improving it.
Still, ideas evolve, and there are always different approaches that perform better or worse. Have different ergonomic etc. Legion is one of the ECS that has come onto my attention as it is the planned replacement for specs in the the Amethyst project. It has been a huge project to try and port the code-base over to it, and it is still not complete.
Announcing Amethyst 0.15.0. Better panic messages, configurable log levels, and more!
Welcome to the seventh issue of the Rust GameDev Workgroup’s monthly newsletter.
Nannou is an open source, creative coding framework for Rust. Today marks one of the biggest milestones for the project since its launch - the release of version 0.13.
This version is particularly special for our community as it opens the floodgates for a lot of pending graphics work and paves the road ahead for running nannou on the web!
Running Retro Games with Rust is not that hard on PineTime Smart Watch... Here's how I ported the libchip8 CHIP8 Game Emulator to PineTime.
In Part 1 we looked at quickly doing operations on all items in a vector, using many CPU cores.
In this part we're looking at making it possible to update data in some other vector while traversing the first vector.
This is harder than it looks, since we can't just allow the parallel threads to update the auxiliary vectors. If we do, there will be races, unless we somehow know that different threads won't ever update the same item.
I started making The Recall Singularity in November 2018, remaining part-time through today. It took a shove out the door by my BFF to believe in myself and the concept right at the start. As TRS has matured so have my skills. At the beginning I held many doubts around my own ability to deliver. As the months have passed this concern has faded away, leaving me with the confidence that the future is in my hands.
godotIntro This is a short guide to get going with Rust and Godot 3.2. This is written with Linux in mind but most of the knowledge should be transferable to MacOS and Windows.
This blog post and git describes my search for a way to parallelize simulation of cars, intersections and roads in a computer game project. I won't be talking anything about the domain, but the problem is something like this:
There are three types of objects: Intersections, Roads, Cars.
Intersections have red lights, and alternately admit cars from different roads into the intersection. When a car arrives at its destination, the car object is updated changing its state from 'on road' to 'idle'.
The gist of it is that calculations are done for each intersection, and for each intersection the calculation may yield an update to a road or a car. There are hundreds of thousands of intersections, about the same number of roads, and a million cars. Calculations need to be quick, so we should run as parallel as possible.
Welcome to the sixth issue of the Rust GameDev Workgroup’s monthly newsletter.
Each evening after the kids are asleep, I load up some music and decide what I want to work on next. If I’m feeling visual, I load Blender or Substance Painter. If I’m in a “quick progress” or prototyping mood I jump into Godot and if I want to deepen my gameplay it’s time to load up and edit my simulation in Rust using CLion.
In August last year, we conducted a survey for the Rust gamedev ecosystem. After an unfortunate delay, we can finally present the results. We received a whopping 403 responses! This trove of valuable feedback will inform the WG's roadmap for 2020.
I’ve been trying to learn Rust lately, the hot new systems programming language. One of the projects I wanted to tackle with the speed of Rust was generating 3D polyhedron shapes. Specifically, I wanted to implement something like the Three.js IcosahedronGeometry in Rust. If you try to generate icosahedrons in Three.js over any detail level over 5 the whole browser will slow to a crawl. I think we can do better in Rust!
wasmIn this course, we will build a Snake Game with Rust, JavaScript, and WebAssembly. We will learn how to export API implemented with Rust to JavaScript app. We will get to know canvas rendering, applications of vectors, and basics of game development.
In the previous chapter, we created a window and gave it a background color. Next, let's draw some paddles and make them move!
ecsI’ve heard from a few people who are just getting started with entity component systems (ECS) that implementing logic for a turn-based game seems more complicated than it should be. I thought that seemed odd, but I just recently ran into this problem myself. While certainly not insurmountable, implementing turn-based logic in an ECS just doesn’t feel great. I think the reason is that no one likes to implement a loop via distributed state machines.
Some weeks ago I started looking at using Rust for making a 64K intro. I started out by making a minimal window app that takes up only 3.5Kbytes without any compression. Some of the feedback I got encouraged me to have a look at Crinkler for compressing the executable. Given that crinkler is really targeted at 4K intros I decided to try creating a minimal modern OpenGL app that can be squeezed into 4K or less.
Welcome to the fifth issue of the Rust GameDev Workgroup’s monthly newsletter.
In this tutorial, we'll build a simple Pong clone using Tetra.
This guide is targeted at people who have a basic understanding of Rust, but have not used it for game development before. If you have read The Rust Programming Language, you should be able to follow along without getting lost - if you can't, please file an issue so I can fix that!
MuOxi is a modern library for creating online multiplayer text games (MU* family) using the powerful features offered by Rust; backed by Tokio and MongoDB,. It allows developers and coders to design and flesh out their worlds in a fast, safe, and reliable language.
Current Status: The codebase is currently in alpha stage . Majority of development is done on the master branch. There is a working TCP server that allows for multiple connections and handles them accordingly. Effort is focused at the moment in designing the backend database structure using a combination of MongoDB and json files.
c2rustThe Rust-loving team at Immunant has been hard at work on C2Rust, a migration framework that takes the drudgery out of migrating to Rust. Our goal is to make safety improvements to the translated Rust automatically where we can, and help the programmer do the same where we cannot. First, however, we have to build a rock-solid translator that gets people up and running in Rust. Testing on small CLI programs gets old eventually, so we decided to try translating Quake 3 into Rust. After a couple of days, we were likely the first people to ever play Quake3 in Rust!
wasmI've been studying WebAssembly recently, which has included porting some of my m4vga graphics demos. I started with the Rust and WebAssembly Tutorial, which has you use fancy tools like wasm-pack, wasm-bindgen, webpack, and npm to produce a Rust-powered webpage.
And that's great! But I want to know how things actually work, and those tools put a lot of code between me and the machine.
In this post, I'll show how to create a simple web graphics demo using none of those tools — just hand-written Rust, JavaScript, and HTML. There will be no libraries between our code and the platform. It's the web equivalent of bare metal programming!
The resulting WebAssembly module will be less than 300 bytes. That's about the same size as the previous paragraph.
ggezFirst off, I’d like to thank everyone for what can only be described (for maximum awkwardness) as an “outpouring” of support in the wake of last year’s unemployment and general grumpiness. It didn’t exactly go viral, but half a dozen people inviting me to apply for jobs at various places and a noticeable uptick in Patreon donations does wonders for one’s self-esteem. Thank you all.
Since I don’t have much new to report on ggez itself, let’s talk about random things that have happened in the greater vaguely-Rust-gamedev ecosystem!
vulkanThis walkdown (somewhere between a walkthrough and a rundown) is about how I handled loading textures to the GPU using Vulkan in my Rust game engine from scratch!
physicsSalva is a 2 and 3-dimensional particle-based fluid simulation engine for games and animations. It uses nalgebra for vector/matrix math and can optionally interface with nphysics for two-way coupling with rigid bodies, multibodies, and deformable bodies. 2D and 3D implementations both share (mostly) the same code!
I'm Ryan, maintainer of Quicksilver 67 and a relatively new maintainer of Winit, a pure-Rust library for creating and managing windows. We're used by a wide range of desktop and gaming software, from Alacritty and Servo to ggez and Amethest. With an alpha release of Winit's next version (0.20.0-alpha4), we've brought Winit to a new platform: the web!
graphics-2dAbout a year ago, in the lyon in 2018 post on this blog, I mentioned that I was working on a complete rewrite of lyon's central piece, the fill tessellator. I have been working on this for quite a bit. The work-in-progress pull request was created in February 2018. Almost two years in the making, this work made it in version 0.15, the project's biggest release ever.
It was a lot of work. Too much work. Fortunately, I am pretty happy about the result.
This crate is my attempt to be the easiest way to create a hardware-accelerated pixel frame buffer. The stated goals and comparison with similar crates can be found in the readme. Below is a discussion of use cases where I imagine pixels will fill an existing need.
TL;DR: It puts pixels on your screen.
Valora is a brush for generative fine art. With valora you can write visual compositions that: are repeatable with rng seeds managed for you, can be reproduced at arbitrary resolutions without changing the scale of the composition, have strict type-safe color semantics that won't surprise you in print (thanks to palette!), leverage all of your hardware (You can shade each path with a different fragment shader!), you will rarely have to debug, thanks to Rust!.
This guide covers valora in depth, but to start let's see some pixels!
As WebGPU specification is approaching a usable shape, we are working on prototype implementations in both Gecko and Servo. If you ever wondered about what it’s like to implement a complex Web API in Gecko, this article is for you. It’s focused on the plumbing: what the parts of implementation are, and how they fit together. We are going to start with the user-facing API (JS on the Web) and go down the shaft till we reach the GPU.
gtkgled is an application for creating animations and effects on light installations. Featuring: All animations are based on the beat of the music, All lamps are controlled using Art-Net udp protocol, Lamps are placed at the correct location in a SVG file, Leds/Lamps can be grouped in "render groups" which enable very flashy effects, gled is a single binary, very small and uses little system ressources while maintaining 60 frames per second with thousands of lamps.
Rendology is a 3D rendering pipeline based on Glium and written in Rust. It features basic implementations of shadow mapping, deferred shading, a glow effect, FXAA and instanced rendering. In this blog post, I’ll outline some of the concepts of Rendology and describe how they came to be this way.
Time ago browsing reddit i found the following meme, and i thought, this seems like a good excersice to practice some rust code. Although im going to do it only with gifs.
> When IOS keeps you from screen recording Netflix so you screenshot every frame and stitch it together in PowerPoint
Yep, thats right, im going to write a rust program that takes a gif and tries to map every frame to a different slide in a powerpoint file and have it animate. Why? Because why not?
In this post, I will start to talk about the exciting technical stuff around Paddlers. If you haven’t read my other posts (#0, #1, #2), Paddlers is a game in which your goal is to make ducks happy. As of recently, I also have an online live-demo where you can look around and see the current progress. (You can log in using a test user: Username: Tester, password: 1) Just don’t expect too much, it is not a playable game, yet.
Paddland, the world in which the game plays, is a colorful place to relax and have fun. And today, I show you how building this is also fun, using Rust, WebAssembly, and some good old creative freedom to glue everything together.
Welcome to the fourth issue of the Rust GameDev Workgroup’s monthly newsletter.
Rust is a systems language pursuing the trifecta: safety, concurrency, and speed. These goals are well-aligned with game development.
We hope to build an inviting ecosystem for anyone wishing to use Rust in their development process!
A peek inside the day-to-day work with Rust and open source game development at Embark Studios.
luminance is an effort to make graphics rendering simple and elegant. It was originally imagined, designed and implemented by @phaazon in Haskell and eventually ported to Rust in 2016. The core concepts remained the same and the crate has been slowly evolving ever since. At first used only by @phaazon for his Rust demoscene productions and a bunch of curious peeps, it has more visibility among the graphics ecosystem of Rust.
So you wanted to use the very best engine, while also using the very best and most performant language?
Well, I have the guide for you — but this adventure is not for the faint of heart. You’re going to run into a lot of issues with the integration, with Godot and also challenges with Rust.
Very slowly over a period of around a year, I wrote a NES emulator. It was the first emulator I’ve written, and it was the first non-trivial project for which I used the Rust programming language. It’s been a fun experience to travel back in time and appreciate the history and architecture of old systems, so I wanted to highlight some of my favorite parts of emulating the NES.
“The Ten Top” is the working title of a game that I’m writing in Rust using the Amethyst game development framework. My goals with the project are to make a fun casual simulation game in the vein of Game Dev Story and Overcooked.
WASM has been making a lot of progress recently so I was looking for a small project to run through and tetris with rust came to mind. Its small enough to understand easily and complicated enough that it is not quite a ‘hello world’ program. We are mostly going to be looking at the interaction between WASM and the browser, there is interesting stuff happening on top of WASM like WASI. The code for tetris is not relevant to the main goal (maybe a later article).
We're going to build a small demo with the nannou creative coding framework for Rust. This example itself is very simple, but specifically over-engineered to prepare to scale even further for your own project.
I’ve been making a game engine in my spare time for a game called The Clockwork. I’ve been loving it, but recently have decided that I need to rework the build process. Let me walk you through that here, show you ways to hook into Cargo, Rust’s build system, and the pros and cons of my approach.
Sulis, a turn based tactical RPG written in Rust. Sulis is open source with turn based, tactical combat, deep character customization and an engaging storyline. The game has been built from the ground up with modding and custom content in mind. Currently supported on Windows and Linux platforms.
This tutorial is primarily about learning to make roguelikes (and by extension other games), but it should also help you get used to Rust and RLTK - The Roguelike Tool Kit we'll be using to provide input/output. Even if you don't want to use Rust, my hope is that you can benefit from the structure, ideas and general game development advice.
VeoLuz is an exploration-focused playground and an artistic tool. It attempts to mimic the behavior of photons as they interact with barriers of various kinds − those that absorb light, those that reflect light, and those that refract light.
Huge round-up of updates to Rust games, libraries, and tools.
My project for the last few months has been creating a 3D rendering engine in Rust, which turned out to be much harder than expected. The original intent was to make a game-engine style thing with built-in support for object loading, cameras, scene graphs, and all that good stuff. What I've ended up with is three separate crates: a small helper for creating command buffers and managing windows, a Vulkan abstraction, and a set of demos showing off what the Vulkan abstraction can do (not much, right now). Pretty.rs in the test-render-engine repository is what's shown in the video, the other demos are less interesting.
Release Notes for v0.7.2
Ferris Fencing is a live tournament in which player-programmed bots combat each other on a RISC-V virtual machine. It is a showcase of CKB-VM, a simple implementation of the RISC-V instruction set, written in the Rust programming language.
Want to build a desktop app? You probably want to use Electron or Qt or GTK or a native toolkit. Want to build a thing that you could (maybe) someday use to build a native desktop app? Contribute to Druid! Druid is a work-in-progress, open source "data-oriented Rust UI design toolkit." You can use it right now to build very feature-incomplete desktop applications for macOS, Windows, and Linux.
Emulators are cool! They help preserve games, improve games, and help make games more accessible. On top of that, making an emulator is a cathartic and satisfying technical challenge!
So, I took this challenge myself and came out the other end with a pretty limited NES emulator, which I call Lochnes. It’s not very good at actually emulating most games, but I’m pretty happy with the guts of the thing and I learned a lot a long the way. I figured it might be worthwhile to share my approach, which might help or inspire others on their own emulation venture!
It's been about 51 days since I first announced Raygon, a WIP high-performance CPU path tracer written in the Rust programming language, which will feature state of the art light transport integrators, including path tracing, bidirectional path tracing and VCM.
In this post I'll go over some of the features implemented or improved, and show some recent renders, then talk about the future of Raygon and how you can help.
Ruffle is an Adobe Flash Player emulator written in the Rust programming language. Ruffle targets both the desktop and the web using WebAssembly.
OxidizeBot as an open source Twitch Bot empowering you to focus on what's important. It allows for a richer interaction between you and your chat. From a song request system, to groundbreaking game modes where your viewers can interact directly with you and your game.
A library for reading and writing LDraw model files and rendering the model using OpenGL for both native and web!
This tutorial will show you how to write a roguelike in the Rust programming language and the libtcod library. In this update the Asciidoctor documents were changed to allow generating the final source files at the end of each chapter directly from the tutorial text.
gfx-rs is a Rust project aiming to make low-level GPU programming portable with low overhead. It’s a single Vulkan-like Rust API with multiple backends that implement it: Direct3D 12/11, Metal, Vulkan, and even OpenGL. wgpu-rs is a Rust project on top of gfx-rs that provides safety, accessibility, and even stronger portability. This is an update that is not aligned to any dates or releases. We just want to share about some of the exciting work that landed recently, which will make it to the next release cycle.
The games I play come with simple ballistic physics for projectile-based weapons: the projectiles are fired with an initial speed and are only affected by gravity. The resulting trajectory is a ballistic trajectory.
Because gravity is a harsh mistress literally every game ‘cheats’ this and fakes a much lower gravity constant for projectiles than is used for players. Without this hack projectiles are either pathethic or need a very high velocity to compensate.
In this article I present a method of calculating where to aim your weapon which fires a projectile under simple gravity to hit an arbitrary moving target in 3D space.
pixel-artSelective Anti-Aliasing is a common technique in pixel art. The idea is to color some parts of the outline to a different color so it blends better with the background color. I wrote this simple algorithm: Given a pixel perfect line, Calculate the length and direction of each 1-px-wide chunk, and For each chunk, color some fraction of the length to some other color.
pixel-artrx is an extensible, modern and minimalist pixel editor implemented in Rust. It's designed to have as little UI as possible, and instead takes inspiration from vi's modal nature and command mode. Compared to other pixel editors, rx aims to be smaller, yet more configurable and extendable. `rx` takes a different approach when it comes to animation as well, which is done with *strips*.
If you made it here you might have already read my previous post 24 hours of game development in Rust, but if you haven't, it was basically a short summary of how I started learning rust and decided to make a game with it. I started with a classical OOP/trait architecture that was taking me nowhere really fast, so I switched to ECS and got a minimal prototype working. Fast forward 6 months, I wanted to catch you folks up on where I am and what I've learnt so far.
Rust’s combination of low-level control, excellent performance and modern build tools makes it an exciting choice for game developers. The idea of a working group to support this burgeoning community has been proposed many times over the years, and we’re excited to announce that a group has finally been formed!
If you’re new to Amethyst, now is a great time to get better acquainted with our project, developed by a global collective numbering in the double digits. The engine is settling into more frequent releases, making it easier for gamedevs to keep up with our incremental improvements. We’ve added a second showcase game to teach by example whilst demonstrating what Amethyst is currently capable of. And best of all for the newcomers, we’ve introduced the “2D quickstarter”, a simple game template that gets you up and running with a working Amethyst game as quickly as possible.
As I've been learning the Rust programming language lately I thought creating a photo-mosaic generator could be a fun project. I knew a generator like this would require a large set of tile images to sample from. The original plan was for it to only create mosaics from emoji which is how it got it's name emosaic (emoji + mosaic = emosaic) but as I progressed it made more sense to keep in generic and let the user provide their own pool of tile images.
Lately, I’ve been wanting to re-write demoscene-like applications. Not in the same mood and way as I usually did, though. Instead, I want to build small things for people to play with. A bit like small and easy to use audiovisual experiences (it can be seen as small video games for instance, but focused on the artistic expression as some games do).
The thing is, the kind of program we want generates its own inputs based on, mostly, the speed at which the hardware it’s running on is able to render a complete frame. The faster the more accurate we sample from that continuous function. That is actually quite logical: more FPS means, literally, more images to sample. The difference between two images will get less and less noticeable as the number of FPS rises. That gives you smooth images.
The “challenge” here is to write code to schedule those images. Instead of taking a parameter like the time on the command-line and rendering the corresponding image, we will generate a stream of images and will do different things at different times. Especially in demoscene productions, we want to synchronize what’s on the screen with what’s playing on the audio device.
Are you looking to build 2D games with Amethyst? Well, we've got some great news for you! Getting started just became a whole lot easier. There's been 2 recent project releases that we want to tell you more about; encourage you to use them, give us feedback and maybe even contribute!
Role-playing and adventure games often require a considerably high number of names to describe characters, locations, items, events, abilities, etc. Humans are very awful generators of randomness, especially upon request. Writers and designers can come up with a handful of well devised names for important identifiers, but having a human generate 100 character names will likely result in many duplicates or boring derivatives. There are a few approaches to this problem: Pattern substitution of a data set with explicit rule-based probability. Using Markov chains to synthesize new results from a data set. Training a neural network to synthesize new results from a data set.
We are having fun moving the gfx-rs project forward thanks to the power provided by the Rust language. We are building rich abstractions which expand beyond the gfx-hal API itself and into the internal layers of the backends, structured to be modular and maintainable. We are building high-performance graphics and compute infrastructure that deeply interacts with OS and drivers, thanks to the Rust’s FFI capabilities and the lack of runtime. We do all of this while requiring only a portion of our developers’ time and attention, who work on gfx-rs mostly as a side project. Rust allows us to move forward confidently and quickly, experiment with features as well as land production-quality code.
DMG-01 is a guide for how to emulate a Game Boy. Together, we'll explore the insides of one of the world's most beloved computers.
Today is an exciting point in the evolution of native GUI in Rust. There is much exploration, and a number of promising projects, but I also think we don’t yet know the recipe to make GUI truly great. As I develop my own vision in this space, druid, I hope more that the efforts will learn from each other and that an excellent synthesis will emerge, more so than simply hoping that druid will win.
In my work, I have come across a problem that is as seemingly simple, yet as difficult to get right, as making decent tea: handling smooth window resizing. Very few GUI toolkits get it perfect, with some failing spectacularly. This is true across platforms, though Windows poses special challenges. It’s also pretty easy to test (as opposed to requiring sophisticated latency measurements, which I also plan to develop). I suggest it become one of the basic tests to evaluate a GUI toolkit.
Amethyst 0.11.0 comes packed with the Rendy integration & many small additions throughout the engine.
gfx-rs project started with a simple idea: separate the API-specific logic of interaction with the graphic driver from a Rust application. That idea was brewing in the heads of @kvark and @bjz precisely 5 years ago, when they realized the common goal and kicked off the project. The Rust game dev community at the time consisted of a few prominent projects (like kiss3d, claymore, and q3) driven by individuals. They used gl-rs for rendering with no strong separation between higher levels, built as mostly monolithic systems. Because GL was known to keep the CPU occupied on the owning thread, we wanted to provide a separate thread dedicated to talking to the GPU. We had a lot to learn, some great contributors to meet, and hoped to eventually make Rust ecosystem a better place.
Nannou is an open source, creative coding framework for Rust. Today marks one of the biggest milestones for the project since its launch - the release of version 0.9. This version is particularly special for our community as it lands the last eight months of progress into master and onto crates.io. While some of us have already been using the work-in-progress 0.9 branch in our personal and commercial work over the past few months, it is a relief to finally be able to land and share the progress with the wider world!
The biggest change for this release is that I started writing a parser for binary Blender files. There is a blog post, where I talk a bit about that, how to explore binary Blender files, and basically how to reconstruct useful information from their DNA.
Entity systems won by a long shot, so that’s what I’m going to be writing about today. In particular, I’m going to outline the process that lead me to Way of Rhea’s current entity system. Way of Rhea is being built in a custom engine and scripting language written in Rust, but the ideas described should still be applicable elsewhere. Hopefully this writeup will be found helpful, or at least interesting. :)
Today marks the first stable release of Evoli, an evolution-inspired simulation game made in Amethyst. We’ve successfully completed the MVP spec (and then some) as it was laid out back in February.
At long last we get to the point of tracing rays - not entirely as we want.
The Piston core is a set of libraries that defines a core abstraction for user inputs, window and event loop. If you take a look at the dependency graph in the README, it might look a bit scary: In this post I will go through each library, explain what it does and describe the status of stability.
To explore a bit the Blender binary file format and provide tools to read and use them I started a new repository on Codeberg. Finally I want to read Blender files directly, and render them with my own renderer. But on my way to develop such a thing, there are many other possibilities, e.g. one could convert Blender files to a new file format, which does not only work for Blender and one single renderer, but would allow any Digital Content Creation (DCC) tool to save to, and any renderer to read from. Anyway, that's a complicated topic and let's start far simpler, by exploring Blender's file format. You get the source code of Blender for reverse engineering (create a debug version and single step through file related code with a debugger) and some Rust code (provided by me) to see what I have figured out so far...
This post is about pathfinder, a GPU vector graphics renderer written in Rust by Patrick Walton as part of his work in the emerging technologies team at Mozilla. Pathfinder can be used to render glyph atlases and larger scenes such as SVG paths. The two use cases are handled a bit differently and in this post I will be focusing on the latter.
After I posted the previous post, one of my friends remarked, "i like the way there is no raytracing in the first post its just faffing to get a window". This is an entirely accurate diagnosis. But, right now, have a representation of a frame buffer as a container (probably a vector) of pixels, which we can pass to the window to render a frame. Now we need to figure out what we’re drawing to it!
There are a couple of tweaks I need to make to this - a representation of the framebuffer in linear float space, for example. But let’s come back to that. I want to trace rays.
I just spent the past two weeks building a GUI Rust app to help you generate a 3-d model of terrain from around the world, that can then be used for 3-d printing, rendering, or whatever you like.
Zemeroth is a turn-based hexagonal tactical game written in Rust. You can download precompiled v0.5 binaries for Windows, Linux, and macOS. Also, now you can play an online version (read more about it in the "WebAssembly version" section).
Is the traditional 2D imaging model nearing the end of its usefulness, or does it have a shiny future in the “modern graphics” world? I spent a week on a research retreat in a cottage in the woods to answer this question, as it shapes the future of UI toolkits. Performant UI must use GPU effectively, and it’s increasingly common to write UI directly in terms of GPU rendering, without a 2D graphics API as in the intermediate layer. Is that the future, or perhaps a mistake?
Vulkan is a C API so we’ll need some kind of bindings to be able to use it in Rust. The API is defined in a XML file distributed by Khronos. This file describes the structs, enums, constants, and functions for each version of the API, and all published extensions. The functions can then be loaded from the Vulkan dynamic library and other functions from the API.
However using a raw C API isn’t easy in Rust because it requires using a lot of unsafe code. This is why we’ll also generate builders for all structs so we can for instance fill in pointer/size pairs using slices, but we’ll also generate methods that return Rust’s Results and take in Rust-friendly types like references instead of raw C types. Finally we’ll also generate loaders so we don’t have to manually load the function we need.
People on the gamedev channel of the Unofficial Rust Discord were talking about graphics API’s and what goes where and what does what, people were contradicting and correcting each other, the rain of acronyms was falling hard and fast, and it was all getting a bit muddled. So I’m here to attempt to set the record straight. This is intended to provide context for people who want to get into writing graphics stuff (video games, animations, cool visualizations, etc) in Rust and don’t know where to start.
Sandspiel is a falling sand game I built in late 2018. I really enjoyed writing this game, and wanted to put into writing some of my goals, design decisions, and learnings from that process.
This is a toy program to render Iterated Function System fractals with Rust and OpenGL. Glium is used for OpenGL and the GUI is provided by imgui-rs.
I realised early on that the heart of the game (engine) was going to be the ECS, and while there are many good Rust written crates for an ECS implementation available, I wanted to write my own as an exercise. And so getting it right (for some definition of right) early on would save me a lot of headaches later on down the track. In this post I'll explain the what/where/how/why and some of the intricacies of using Rust for it.
gfx-rs is a Rust project aiming to make graphics programming more accessible and portable, focusing on exposing a universal Vulkan-like API. It’s a single Rust API with multiple backends that implement it: Direct3D 12/11, Metal, Vulkan, and even OpenGL. We are also building a Vulkan Portability implementation based on it, which allows non-Rust applications using Vulkan to run everywhere. This post is focused on the Metal backend only.
Previously, we benchmarked Dota2 and were able to run many other applications and engines successfully, including Dolphin Emulator. For Dolphin, we previously focused on visual correctness. After games appeared to render correctly, we shifted our focus to performance to ensure they also render quickly.
Recently, I made an emulator for the Nintendo Entertainment Console(NES) - a game console first released in 1983. In this article, I’ll talk about how I used Rust to develop the emulator. I’ll cover questions like: What features does the emulator support? What games can it play? How did I approach the problem of emulating the NES? Did Rust’s type system or borrow checker interfere? Were there performance issues?
In this post I'll walk you through how you can create game user interface with imgui and ggez. I spent a lot of time trying to make this work for my game (which you can read more about here) and suffered greatly because of the lack of documentation, so I'm writing this so you don't have to!
WebGPU is a new graphics/compute API developed by the browser vendors (and Intel) within W3C: The goal is to design a new Web API that exposes these modern technologies in a performant, powerful and safe manner.
Don’t be confused by the “Web” part here - both us (gfx-rs team) and Google are trying to make it feasible to run on native platforms as well. Typically, the Web as a platform has different priorities from native: a lot of focus is placed on the security and portability (in a wider and stronger sense). Coincidentally, these are qualities we are currently missing in the gfx-rs ecosystem: security means safety (in the Rust sense), and portability means that people can use it and run everywhere, without worrying about thousands of potential configurations at run-time, or diverging behavior between platforms due to timing differences or loosely defined behavior.
With these goals in mind, we’d like to announce our new project: wgpu-rs.
In the last post I talked about a small game I built in Rust and roughly how far I got in 24 hours. One of the biggest challenges I had was…
In this post I'll talk about a small game I've been developing in about 24 hours in total (mostly in a few hour blocks during evenings or weekends). The game is far from finished, but I thought I'd write up about my experience so far, what I've learnt and some interesting observations about building a game from scratch-ish and doing it in Rust.
Wave Function Collapse is a procedural generation algorithm which produces images by arranging a collection of tiles according to rules about which tiles may be adjacent to each other tile, and relatively how frequently each tile should appear. The algorithm maintains, for each pixel of the output image, a probability distribution of the tiles which may be placed there. It repeatedly chooses a pixel to “collapse” - choosing a tile to use for that pixel based on its distribution. WFC gets its name from quantum physics. The goal of this post is to build an intuition for how and why the WFC algorithm works.
This post is actually a collection of updates about pretty big things, but the writeup is small. Several will be expanded into larger blog posts - if there are any that you are especially eager to see, please tweet at me and I’ll give the topic priority.
Learning Rust through the porting of the code from Peter Shirley's Ray Tracing in a Weekend. Focused on Polymorphism, Ownership and Traits in Rust.
I want to participate in this year's 7 Day Roguelike Challenge. If you've looked around this blog, you know the language is going to be Rust. But for the 7DRL, I'd really love if people could play it in the browser. What follows is a little guide to get you to a small playable proof of concept that can build native Windows, macOS and Linux executables but also runs in the browser via WebAssembly.
This blog post is a brief summary of what happened the past 8 months in the Piston project.
Building a cross platform game for desktop operating systems in Rust is fairly doable without needing much platform specific code. Glutin is a Rust alternative to SDL for handling window creation & input. GFX handles most of the graphics API abstraction for you. You still write the shaders, but I was able to just use OpenGL and get it working on Windows 10, MacOS, and Ubuntu.
StarCraft: Brood War. This game means so much to me! And to many of you, I guess. So much, that I wonder if I should even give a link to its page on Wikipedia or not.
Once Halt sent me PM and offered to learn Rust. Like any ordinary people, we decided to start with hello world writing a dynamic library for Windows that could be loaded into StarCraft's address space and manage units.
OpenMoonstone is a open source reimplementation of Moonstone: A Hard Day's Knight following along the lines of projects like OpenTTD and OpenXcom. You can try it out https://github.com/joetsoi/OpenMoonstone
In my spare time I am working on a dwarf colony management game that’s written in rust. I started this project about one year ago and since it has reached this milestone and I didn’t abandon it I think it’s a good time to look at the curent status.
Welcome to the inaugural article of the metaview project. metaview is an effort to create a universal platform for VR/AR applications similarly to how the web browser is a platform for web applications. For more information about metaview and its goals, see About. This article covers ammolite, a work-in-progress rendering engine with focus on VR/AR.
ggez is a lightweight portable game framework in Rust, inspired by LÖVE. I do most of the actual maintenance and planning behind it, and I’ve been sort of in and out of contact this year, so I thought I’d write up a little thing about the present and future of the project. I remembered that at the beginning of last year I wrote GgezOnWasm, but only remembered a little of what went into it, so I went back and read it. And then got hives from the stress of all the promises I’ve made. So, I feel justified in being a bit slow with working on ggez lately.
wlroots is a hip new Wayland compositor framework that Way Cooler has been using for about a year now. I’m going to go ahead and declare 2019 the year of wlroots. Most of the work was done in 2018, but this year is when major compositors will begin to use it. Currently the only usable Wayland compositor that uses wlroots is sway, which is fast approaching a stable 1.0. However there is a long list of startup compositors (including Way Cooler) that are using wlroots. At least some of them are expected to come into their own as alternatives to traditional X11 based systems this year, and it’s all thanks to wlroots.
In this tutorial we’ll discuss the ideas and concepts behind rendering water and then talk through some demo code.
Here are some followups on previous blog postings. Including, Arclength, and 2D graphics.
I’ve recently created a new website and finished an online game named Adventures of Pascal Penguin. One of the most unique things about this game is that it was written in Rust and runs in web browsers thanks to WebAssembly. WebAssembly has only been mainstream for about a year or so, so not many games have been created like this. I want to share a little about my journey with Rust: how I started with it and how I got to this point.
Russian AI Cup — annual IT-oriented competition initiative, organized by Mail.Ru Group and Codeforces. Last year we started using Rust compiled to WebAssembly to show the games on web. This time, we went even further, and the game itself is now written in Rust. So, now web player, local game runner and testing suite is actually same application with same source code. This means that you can, among other things, play the game in browser, which was not possible before.
In 2018 the project left the nursery and entered the adolescence period...
This past weekend I made a game for Ludum Dare 43. Tools used: Aseprite, quicksilver. Inspired by Zachtronics. It is written in Rust and compiled to WebAssembly.
In my post on GPGPU in Rust, I declared that I intended to work on improving the state of CUDA support in Rust. Since then, I’ve been mostly radio-silent. I’m pleased to announce that I have actually been working on something, and I’ve finally published that something.
RustaCUDA RustaCUDA is a new wrapper crate for the CUDA driver API. It allows the programmer to allocate and free GPU memory, copy data to and from the GPU, load CUDA modules and launch kernels, all with a mostly-safe, programmer-friendly, Rust-y interface.
The Amethyst team has some special announcements. A non-profit foundation has been formed and a new community forums site has been created.
I wrote a nightly Rust library called immense for synthesizing 3D structures with simple composable rules, inspired by Structure Synth. In the docs I cover the basics, and in this article I’ll go over making a mesh from start to finish.
Rust is excellent for performance crucial applications that run on multi-processor architectures and these two aspects are also critical for game development. Rust has already seen a bunch of interest from games developers like Chucklefish, Embark Studios, Ready at Dawn, etc. - but in order to really excel I’d love to organize some structured efforts to improve the ecosystem and I think it would be great if the 2019 roadmap will include game development.
In contrast to many other ECS, iteration in Pyro is fully linear. Different combinations of components always live in the same storage. The advantage is that iteration is always fully linear and no cache is wasted. The storage behind the scene is a SoA storage.
In this post I'll write about an piece of the low level details of an hypothetical rust 2d graphics crate built on top of gfx-hal. Gfx provides a vulkan-like interface implemented on top of vulkan, d3d12, metal or flavors of OpenGL. just like the previous post this is in the context of recent discussions about a 2d graphics crate in rust.
glsl-quasiquote-0.2 was released early this morning. This new version provides a more stable public API. Two major changes: The glsl_str! proc-macro would have only survived the 0.1 version. It’s now deprecated and will be removed soon. The glsl! proc-macro now supports GLSL pragmas (both #version and #extension).
When triangle meshes are rendered by a GPU, there are pipeline stages that need to load and process vertex and index data. The efficiency of this process will depend on the layout of the data, and how the GPU is designed. There is an excellent library from Arseny Kapoulkine called meshoptimizer, which provides a variety of algorithms for optimizing geometry for the GPU.
We want to share some of the progress being made on Amethyst! As we've had a lot of expansion, we've also revamped the way we manage the project and we have some very interesting new features being worked on.
Ralph Levien recently published A crate I want: 2d graphics on his blog, which started some interesting discussions on reddit. At the same time there is a nascent discussion on the draw2d repository (which doesn't have any code at this point) about a potential 2d graphics crate.
The Rust ecosystem has lot of excellent crates, and many more new ones being published. I believe one is missing, though, and I’d really like to see it happen: a cross-platform abstraction for 2D graphics. In this post I will set out what I want.
With this article, I want to introduce you to game development. I want to give you a little tour of where to start, how to explore possibilities and revive my journey a little bit at the same time. I will try to construct different games from the ground up while writing this article, so you can experience game-dev live.
For the last two parts of this tutorial, all we’ve had to look at on-screen is a single blueish triangle. In this part, we want to display a more complex shape, with more variation in color. To do this, we’ll have to stop hard-coding our triangle mesh in the vertex shader. (And start hard-coding it in the source code!)
glsl-quasiquote-0.1 was released today! The crate provides you with two macros: glsl! and glsl_str!. Both are procedural macros that requires a nightly compiler and the proc_macro_non_items feature. They will both output a TranslationUnit, that represents a whole shader AST.
In this second blog post I’m showing how I implemented objects - things the player can interact with - for a text adventure written in Rust. As usual, the full code is available on GitHub.
A bunch of stuff has happened since I published my post on The State of GPGPU in Rust. Most importantly, Denys Zariaiev (@denzp) released his work on a custom linker for Rust CUDA kernels, and a build.rs helper crate to make it easier to use.
These two crates eliminate many of the problems I referred to in my previous post. The linker solves most of the “invalid PTX file” problems, while the ptx-builder crate does all of the magic that Accel was doing behind the scenes.
We’re going to walk through a Rust application that I’ve built, which is essentially a basic Roguelike in most regards.
I’m attempting to write a (very simple, at least initally) text adventure in Rust, so I’m going to share progress on my blog starting today. This first post is about the sections, that to day is the rooms of a house for example.
It’s been a while since my last post! Lately I’ve been quite demotivated to actually produce something worth while for doing a writeup about. Instead I offer you some thoughts on my recent findings using rust and some crates I found particularly useful for computer graphics.
I said in my talk that I would post a potentially more interesting long-form version of the talk that includes things that I couldn’t fit into the 30-ish minute time slot. What I’ve included below is my original long-form version of this talk, but my original intention was not to post this as-is. Originally I wanted to clean this up a bit more and make this into something that wasn’t so much a giant wall of text, but after I started doing this I realized that I was just rewriting it entirely, and at that rate I would never get around to releasing it, which I promised I would do in a timely manner.
After improving functionality and performance of gfx-portability’s Metal backend through benchmarking Dota2, and verifying certain functionality through the Vulkan Conformance Test Suite (CTS), we decided to expand our testing to other projects. We quickly found two projects which matched our criteria: RPCS3 and Dolphin.
I have made four rust programs for programmatically generating artwork in different styles. Here is a sample in each style, along with an explanation of the algorithms.
This tutorial builds on the code we wrote in the previous part. You can find the new code here with comments explaining everything that’s changed, and run it to see what the end result will look like. Last time we got a single triangle rendering on screen. This time we’re going to look at what we need to do to allow window resizing to work properly.
I realized after my first post of this series that it’s not just a journey into rust but also OpenGL. I’ve used other Graphics API’s before but never actually got my hands dirty into OpenGL. Someone on the rust user forums (they are awesome, go check it out!) suggested using compute shaders instead. At the time I had never used compute shaders for a project so I decided to take some time to refactor the program to use a compute shader. This post is a follow up on that remark and will explore the possibilities of using a rust together with OpenGL to run compute shaders.
I enjoy using OpenGL a lot, but when I switched from C++ to Rust I found it to be less fun to use. This is because the OpenGL (from gl-rs) code never really fit alongside the rest of my Rust code, due to unsafe blocks, PascalCase function names, hacky conversions to c_void pointers etc. So, to make my life easier in the future when using OpenGL in Rust, I made this crate.
Eight part series on using gfx-hal for low level graphics programming.
UniverCity is a university management game being programmed in the Rust programming language. As stated above, the game is in early access and is not complete. There will be changes and additions down the line and finishing it may take some time. You may wish to wait until its further along before buying it.
For this years GSoC I (@fkaa) worked on implementing the DirectX 11 backend for gfx, a graphics API which translates to Vulkan, DirectX 12 and Metal.
One of my favorite game jams is Ludum Dare: It’s just you, a theme, and 48 hours to make a game. Pure and challenging, and I’ve participated in several of them from time to time with a variety of tools. Usually my go-to is Unity3D, though arguably my best game was in Python+Pygame. This time though, for Ludum Dare 42 on August 2018, I finally had both the energy and ability to write my game in Rust, using ggez. So I decided to write about it!
For the past few months I've been toying about with WebAssembly. The examples I've built using WebAssembly were very simple and could easily have been written in JavaScript with perfectly adequate performance. This got me thinking it's about time I make something to really shows where WebAssembly shines. This led me down the path of thinking about very compute-demanding applications. An obvious example is 3d graphics rendering. Even a small scene like the ones I've been creating involve computing millions of vector dot product calculations per second. This kind of CPU-intensive application seemed right up the street of WebAssembly.
gfx-rs is a Rust project aiming to make graphics programming more accessible and portable, focusing on exposing a universal Vulkan-like API. It’s a single Rust API with multiple backends that implement it: Direct3D 12/11, Metal, Vulkan, and even OpenGL. We are also building a Vulkan Portability implementation based on it, which allows non-Rust applications using Vulkan to run everywhere. This post is focused on the Metal backend only.
At work a few months ago, we started experimenting with GPU-acceleration. My boss asked if I was interested. I didn’t know anything about programming GPUs, so of course I said “Heck yes, I’m interested!“. I needed to learn about GPUs in a hurry, and that led to my GPU Path Tracer series. That was a lot of fun, but it showed me that CUDA support in Rust is pretty poor.
The language rust has been popping up on my twitter feed and my personal life more and more. It’s been promoted and presented as the ultra safe language, so naturally I decided to check it out. The upcoming series of posts “Journey into rust” will describe and document my experiences using rust, hopefully explaining certain concepts that rust does differently. This will all be written from a C++ programmers standpoint that was thought writing Object Oriented code. I encourage you the reader to think critically and correct where necessary.
On to the actual first post! After reading “the Rust Programming Language” I wanted to get my hands dirty and actually write some code. I like graphical applications and using low level graphics API’s so I decided to implement a cellular automation in rust. But just implementing cellular automation isn’t very exciting, is it? What if we could do this on the GPU…And off I went on my journey to create Conway’s game of life in rust.
With a brand new tutorial and a ton of new features, including prefabs, controller support, MP3 audio, localisation and an even better ergonomics!
A Vulkan game from scratch in nine days. Using minimal libraries and pure Rust.
I’m excited to announce the brand new website/user-guide for the nphysics2d and nphysics3d crates: pure-rust 2D and 3D real-time physics engines with rigid bodies and joints! Online wasm-based demos are also provided (see for example the Multibody joints 34 demo).
I titled this post “from scratch”, because I am going to assume little knowledge of Rust and basic knowledge of 3D graphics and OpenGL.
Therefore, this tutorial may teach you basic Rust and how to get Rust working with OpenGL, however for in-depth OpenGL learning you will need another tutorial or book.
“From Scratch” also means that we will try to build abstractions ourselves, so that we get better knowledge of Rust. In addition to that, we will able to follow existing OpenGL tutorials, because we will know exactly what OpenGL functions we are calling.
Hello! Welcome to my third and final post on my GPU-accelerated Path Tracer in Rust. In the last post, we implemented all of the logic necessary to build a true path tracer. Problem is, even on the GPU it’s terrifically slow. This post is (mostly) about fixing that.
But first, we need to fix a bug or two, because I goofed. *sad trombone*
Step -1: Fixing Bugs /u/anderslanglands on Reddit pointed out that, since I’m using Cosine-weighted Importance Sampling, I need to do some extra math to avoid biasing the results.
A modern z-machine for the classics. Encrusted is an interpreter for Infocom-era interactive fiction games like Zork. Run the web interface here ↑, or get it for your terminal.
Up to now, all the rendering code has been in the game loop in the main function. The rendering code is simple and straightforward, so, while it should have been extracted into its own function, there wasn't a pressing need to do so. Now that I'm going to enhance the UI, the rendering needs to be extracted.
I now have a map, and a bunch of monsters on the map, able to attack and be attacked by the player. To provide for different kinds of monsters, I needed to add stats like combat strength and HP.
Wikipedia states that flocking is a behavior exhibited when a group of birds, called a flock, are foraging or in flight. Flocking simulation are basically softwares which simulate this flocking behavior of birds. The original algorithm is developed by Craig Reynolds in 1986.
Hello, and welcome to part two of my series on writing a GPU-accelerated path tracer in Rust. I’d meant to have this post up sooner, but nothing ruins my productivity quite like Games Done Quick. I’m back now, though, so it’s time to turn the GPU ray-tracer from the last post into a real path tracer.
Tracing Paths As mentioned last time, Path Tracing is an extension to Ray Tracing which attempts to simulate global illumination.
In the current implementation, the player can see the entire layout of the dungeon from the beginning. The next step is to change the game to start off with the dungeon hidden, so the player will actually have some rooms to explore.
I now have the game up to the point where the player has a random dungeon to explore. It's time to make it more interesting by adding some threats - namely, some monsters.
Cheddar is a GLSL superset language. What it means is that most of the GLSL constructs and syntax you’re used to is valid in Cheddar – not all of it; most of it. Cheddar adds a set of features that I think are lacking to GLSL. Among them: Some non-valid GLSL constructions made valid in Cheddar to ease the writing of certain shader stages; A more functional approach to programming shaders on the GPU; Structures, types and GLSL-specific constructs sharing; Imports and modules with live reloading and transitive dependencies.
In the past few years, I've heard a lot about Rust. As someone that hacks on computer graphics and low-level infrastructure libraries, it seems relevant to my interests. I decided to make a small demo – of a procedural planet generator – and see how it went.
It's time to start making some rooms and connecting them together. These will require making modifications to the map, so I guess it's time to finally refactor the map code into its own legitimate class.
As I mentioned in the last post, until I got a better handle on the off screen console code, I temporarily made all the Rust bindings call out to the default root console. Now that there's a bit of discussion on how the off screen consoles work, it's time to refactor the code to enable their use.
Procedural generation is a technique which allows content to be created programmatically, rather than everything in a game being specifically placed by a designer. Procedural generation doesn't mean completely randomised, rather randomised elements are used as long as they make sense.
This tutorial will show how to create a tilemap-based level with rooms connected by straight corridors, using Rust. We'll also cover how to use seeds to reproduce specific layouts and serialise the output into JSON. The rooms will be placed at random within the level, and corridors are drawn horizontally and vertically to connect the centres of the rooms.
When we built the original Monsters and Sprites demo, we only had 9 days to get it working before the Playcrafting expo we had signed up for, so we had to cut a lot of corners. Since then I’ve been doing bug fixes and working on a lot of miscellaneous engine/language features that I either couldn’t get done in time for the demo, or didn’t realize were important until I started building it. We’ve made a few game updates since then (we now have sound!), but this post is specifically going to explore some language updates I’ve made.
In my first post about my journey to the center of the NES, I was at the point where I was still working on the CPU; implementing new addressing modes and instructions as I made my way through the nestest ROM. Well, I finally finished the CPU, including a handful of the illegal opcodes. The last of the illegal opcodes just need some placeholders, because, as I understand it, very few games use them.
This game was coded in Rust and is playable in web browsers by means of WebAssembly, WebGL and Howler.js. The software I developed is partly open source in the form of Gate, which is the Rust library that powers this game and can power other similar games. Special thanks to the tools I used to create assets: Gimp, FL Studio and BFXR.
In my last post on optimising my Rust path tracer with SIMD I had got withing 10% of my performance target, that is Aras’s C++ SSE4.1 path tracer. From profiling I had determined that the main differences were MSVC using SSE versions of sinf and cosf and differences between Rayon and enkiTS thread pools. The first thing I tried was implement an SSE2 version of sin_cos based off of Julien Pommier’s code that I found via a bit of googling. This was enough to get my SSE4.1 implementation to match the performance of Aras’s SSE4.1 code. I had a slight advantage in that I just call sin_cos as a single function versus separate sin and cos functions, but meh, I’m calling my performance target reached.
The other part of this post is about Rust’s runtime and compile time CPU feature detection and some wrong turns I took along the way.
I gave a talk about lyon at RustFest Paris. This post is the introduction of the talk, wherein I introduce vector graphics and try to get the audience somewhat excited about it. Things will get technical in the follow-up posts.
For the past month or so, I’ve been working on a follow-up to my series on Writing a Raytracer in Rust. This time around, I’ll be talking about writing a GPU-accelerated Path Tracer. As always, I’m writing it in Rust - including the GPU kernel code. Compiling Rust for GPUs at this point is difficult and error-prone, so I thought it would be good to start with some documentation on that aspect of the problem before diving into path tracing.
These early posts will mostly be me trying to work out how to use gfx-rs. I was previously using glium which is fantastic, but is sadly no longer being developed. So my choices are: Learn Vulkan, Use raw OpenGL bindings, Use gfx.
I opted to use the current released version (v0.17.1) but it’s currently undergoing a significant rearchitecture so I may move to that whenever it releases.
I was inspired to work through Peter Shirley’s Ray Tracing in a Weekend mini book (for brevity RTIAW) but I wanted to write it in Rust instead of the C++ that’s used in the book. I found out about the book via @aras_p’s blog series about a toy path tracer he’s been building.
Chucklefish, an independent game studio based in London, publishes hit video games like Stardew Valley and Starbound. Now, the company is developing its next game, code-named Witchbrook, using the Rust programming language instead of C++. Why the switch? Two main reasons: to get better performance on multiprocessor hardware and to have fewer crashes during game play.
This is part 2 of a story on taking the long road towards Rust bindings to Faiss. You may wish to read part 1 first for a motivation section and a deeper understanding of how I built a plain C API on top of the C++ library.
I wrote the NES emulator with Rust and WebAssembly to learn Rust. It’s not perfect and have some audio bugs, but it’s good enough to play Super Mario bros.
Perhaps you have once wondered how search engines such as Google and TinEye enable their users to search for images which are similar to one that you provide, or how they can identify a building from nothing but a picture. Content-based image retrieval (CBIR) is the backbone concept, and provides exciting new ways to search for useful information. While the concept is no longer novel, the requirements imposed on systems for CBIR are ever increasing due to the increasingly larger amounts of data and demand for higher quality of retrieval.
The short version is: I have some shapes. I want to find their intersection.
Really, I want more than that: I want to drop them all on a canvas, intersect everything with everything, and pluck out all the resulting polygons. The input is a set of cookie cutters, and I want to press them all down on the same sheet of dough and figure out what all the resulting contiguous pieces are. And I want to know which cookie cutter(s) each piece came from.
But intersection is a good start.
Hello! This tutorial will show you how to write a roguelike in the Rust programming language and the libtcod library.
UniverCity is a university management game being programmed in the Rust programming language. This month was spent mostly on the business side of things, including going through the steam partner process.
Game Development is one of the fields in which Rust can gain a lot of traction. Let’s look at the current ecosystem and let’s see what the community has to offer.
My nice brother Johannes Ridderstedt sent me some old files a few weeks ago (in late 2017), stuff that he had preserved from an age-old computer of ours. One of these was the file named gameland.zip (not published yet, but I might put it up here some day.) I managed to get this running, and liked what I saw (you'll find the YouTube link to it further down on this page.) Around this time I was reading a bit about WebAssembly which I think will redefine and help reshape the web as we see it today. I was also looking at the Hello, Rust web page, and the "FizzleFade effect using a Feistel network" page in particular.
This is a follow up post. RLSL is a Rust to SPIR-V compiler. SPIR-V is the shading language for Vulkan, similar to other shading languages like GLSL, HLSL but more low level. OpenGL, DX9/11/12, Vulkan, Metal are all graphic APIs that are able to use the GPU to draw pixels on the screen. Those APIs have certain stages that can be controlled by the developer by using the correct shading language.
In an effort to do more fun side projects, I’ve been learning Rust, a wonderful systems programming language developed by the Mozilla Foundation. It’s been a while since I’ve touched a compiled language as my day-to-day often deals with Python and Javascript variants. I was inspired after seeing a lot of interesting articles about Rust usage and decided to dive into learning Rust by creating a very basic 2D game, inspired by the classic Defender arcade game.
Last month I was working on a lot of new test scenes for my Rust implementation of the PBRT renderer. But a big chunk of my time went into implementing the curve shape needed for the geometry of hair, and a material, which implements a hair scattering model.
Lyon is a side-project that I have been working on for quite a while. The goal is to play with rendering 2D vector graphics on the GPU, and it's been a lot of fun so far. I haven't talked a lot about it online (except for a couple of reddit threads a year or two ago) so I figured it would be a good topic to get this blog started.