There are many guides and introductions to Rust.
This one is something different: it is intended for the experienced programmer who already knows many other programming languages.
I try to be comprehensive enough to be a starting point for any area of Rust, but to avoid going into too much detail except where things are not as you might expect.
Getting Started
Introductory posts, guides and tutorials for getting started with Rust. Posts 
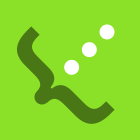
I'm excited to launch Low-Level Academy, an explorable systems programming course that uses Rust and web assembly for interactive playgrounds and visualisation. It starts with network programming, but it will be expanded to more topics!
goRecently I've been starting to use Rust more and more for larger and larger projects. As things have come up, I realized that I am missing a good reference for common things in Rust as compared to Go. This post contains a quick high-level overview of patterns in Rust and how they compare to patterns in Go. This will focus on code samples. This is no replacement for the Rust book, but should help you get spun up on the various patterns used in Rust code.
Rust is a new language that already has good textbooks. But sometimes its textbooks are difficult because they are for native English speakers. Many companies and people now learn Rust, and they could learn faster with a book that has easy English. This textbook is for these companies and people to learn Rust with simple English.
Rust is a language that is quite new, but already very popular. It's popular because it gives you the speed and control of C or C++ but also the memory safety of other newer languages like Python. It does this with some new ideas that are sometimes different from other languages. That means that there are some new things to learn, and you can't just "figure it out as you go along". Rust is a language that you have to think about for a while to understand. But it still looks pretty familiar if you know another language and it is made to help you write good code.
As with any programming language one of the main building blocks of Rust is variable usage. Since I’m just learning Rust I wanted to really dive into the specifics around how it works.
javascriptLearning a new programming language is a great opportunity to learn new universal concepts and patterns that apply to all languages, not just the one you're learning. However, before you can get a handle on all the new stuff a language provides, first you have to figure out how to write the new language like you would write whatever old language(s) you know.
For the JavaScript developer, Rust offers a plethora of new and sometimes brain-bending concepts that exist in Rust but not in JavaScript. But in order to appreciate those concepts, first you have to get a handle on the basic syntax of the language. To speed up that process, you can use the JavaScript you already know to draw parallels to the Rust equivalents.
This cheatsheet provides some basic comparisons between JavaScript syntax and their parallels in Rust. It purposefully sticks to the basics that have decent parallels, to get you comfortable writing some simple programs in Rust.
I'm about two weeks into Rust now, so this feels like a good time to write a critique, before I get Stockholm Syndrome'd.
My main motivation in learning Rust is that I have to maintain some of Dark's Rust code. There was a recent outage related to that code, and I had to learn on the fly, so better to actually know what I'm looking at.
I've also been dreaming of rewriting Dark in Rust for quite some time, largely due to frustrations with OCaml as well as some excellent marketing by the Rust community. I'm trying to evaluate whether this is a good idea, and if so, trying to figure out how to do it in a way that makes sense (which is to say, gradually).
Rust gets a lot of first impression posts. For people in the community, reading those posts can often be frustrating, with factual errors leaving people concerned that others will get the wrong impression. For people outside the community, they may be unsure of how much said is true, or how to read these posts effectively. In this post, I outline how I think about first impression posts, and why I recommend reading them the same way.
Ran into a funny variable shadowing situation, and ended up writing about the details of variables, and shadowing, in Rust.
With RustConf 2020 coming tomorrow, I want to tell folks about how I have been “re-reading” The Rust Programming Language. Affectionately known as “The Book”, this resource is the ultimate guide on how to get started with the Rust programming language. You can buy it at almost any storefront, or view it online for free.
Why should you care about how I read a book?! Psssssh. “I kNoW hOw tO rEaD A BoOk”.
Yeah… I get it… PLEASE STAY, DON’T LEAVE.
The Rust programming language doesn't just aim to be practical, it also aims to be useful for the people working with it. Not only does this improve productivity, it also helps learning the language! One of the features I want to pick out: the borrow checker of the Rust compiler. This feature helps to avoid a great number of memory related bugs by enforcing correct memory allocation and use of pointers. At the same time, it also seems to be the source for a lot of frustration. This is reflected in in a phrase we often see: "fighting the compiler".
lifetimesAs I've said before, I'm working on a book about lifetimes. Or maybe it's just a long series - I haven't decided the specifics yet. Like every one of my series/book things, it's long, and it starts you off way in the periphery of the subject, and takes a lot of detours to get there.
In other words - it's great if you want an adventure (which truly understanding Rust definitely is), but it's not the best if you are currently on the puzzled end of a conversation with your neighborhood lifetime enforcer, the Rust compiler.
So, let's try to tackle that the crux of the issue another way - hopefully a more direct one.
wasmI initially picked up Rust because of the fantastic work the team has done to support and push WebAssembly. The official documentation is a great resource for building an example project.
This guide will serve as an introduction to WebAssembly and a tutorial on how to set up and work in a Rust Wasm environment.
If you feel confused about all the intricacies of Cargo.toml [features] section you are not alone.
First of all, I assume that you have already read the docs at https://doc.rust-lang.org/cargo/reference/features.html to get some foundation of what "features" are before going through these examples. I did read the docs first too, but they left me confused until days later when I had to go much deeper into the topic to make some crates work together. What I think was missing from the docs for me was more examples with explanations how they work. This post was written to fill that gap.
It is the second part of a series about Rust for Python users.
In this article, we will build a foundation for a Rust-powered Python library - a crate that implements CSS inlining. It is a process of moving CSS rules from style tags to the corresponding spots in the HTML body. This approach to including styles is crucial for sending HTML emails or embedding HTML pages into 3rd party resources.
I’ve been learning Rust for the past twenty days or so, working through the Blandy & Orendorff book, coding up things as I go. Once I got into playing with Rust traits and closures and associated types, the similarities to programming in Haskell with typeclasses, data structures, closure passing and associated types was pretty obvious.
As a warm up I thought I’d try porting the stream fusion core from Haskell to Rust. This was code I was working on more than a decade ago. How much of it would work or even make sense in today’s Rust?
Rust is getting more popular among software developers, and the Python community is no exception. I started learning Rust a few years ago, but after some point, I began to lose motivation because most of my exercises were toy examples and far away from the real applications. So I questioned myself:
⏵ Can I use Rust in my day-to-day job as a Python developer?
⏵ Can I build something that will benefit the projects I am working with?Probably, many of us, people coming from the Python background, had similar thoughts. In a 3 chapter series, I will share my experience with embedding Rust into Python projects and try to give you some options that may answer such questions.
ownership-and-borrowingYou’ve heard a lot about it, you’ve bought into the hype, and the day has finally come. It’s time for you to start writing Rust!
So you sit down, hands on the keyboard, heart giddy with anticipation, and write a few lines of code. You run the cargo run command, excited to see whether the program works as expected. You’ve heard that Rust is one of those languages that simply works once it’s compiled. The compiler starts up, you follow the output, then, suddenly:
error[E0382]: borrow of moved value
Uh-oh. Looks like you’ve run into the dreaded borrow checker! Dun, dun, DUUUUUUN!
error-handlingError handling in Rust is very different if you’re coming from other languages. In languages like Java, JS, Python etc, you usually throw exceptions and return successful values. In Rust, you return something called a Result.
The Result<T, E> type is an enum that has two variants - Ok(T) for successful value or Err(E) for error value:
enum Result<T, E> {
Ok(T),
Err(E),
}Returning errors instead of throwing them is a paradigm shift in error handling. If you’re new to Rust, there will be some friction initially as it requires you to reason about errors in a different way.
In this post, I’ll go through some common error handling patterns so you gradually become familiar with how things are done in Rust.
We often hear that Rust is a language that is too complex and too difficult to learn. While, yes, the language is complex, we have identified some existing mindsets and expectations that serve as obstacles to the learning process. Let's discuss these obstacles and how we can alleviate them in our first post on learning Rust.
The countdown problem was presented in a paper by Graham Hutton with a simple and elegant solution in Haskell. See the paper here. In this post I'll implement the same solution in Rust and see how it looks compared to the original Haskell solution.
Rust has two major mechanisms for delegating logic: enums and trait objects, and it may be unclear when to use one or the other. In this post, I will walk through how each works, what the tradeoffs are, and how to choose the right option for your code.
This next algorithm was really fun and a bit more challenging. The Algorithms book I’m going through went through several more examples of breaking a problem into a smaller, simpler problem and letting recursion CPU its way to a solution, like the merge sort we just looked at. Then I moved on to chapter 2 about “backtracking” into the n Queens Problem!
New chapter in "Rust for JavaScript Developers": Pattern Matching, Destructuring, Enum, Option.
Rust, a systems-programming language, which prides itself on being Stack Overflow’s “most loved language for five years in row” and GitHub’s second fastest growing (235% 2018-2019) has gained popularity both internally here at Facebook and externally within the programming community.
As a Developer Advocate on the Facebook Open Source Team, I have been focused on developing my own understanding of the space. I work within the DevTools pillar and focus primarily on programming languages. My main priority for H1 of 2020 has been Rust. The goal of this article is: to paint a high-level overview of the ecosystem.
The article covers various aspects of the ecosystem including community, companies using the language, selling points, etc.
A blog post titled, "Diving into Go by Building a CLI Application" has been making it's rounds of the internet. It uses a small XKCD downloader as the subject. I thought was small and self contained enough, that it'd be interesting to see the same example in Rust!
Both contain utf-8 encoded text, but:
* String owns the data stored within it. When the String is deallocated the text data it stores is deallocated as well.
* &str borrows data that is stored elsewhere (usually in a String), it doesn't store any text data of its own. When a &str is deallocated the data it points to is left untouched.Handily, a &str can be shorter than the String it references. This helps make string handling fast and memory efficient as we can simply break up a pre-existing String into chunks that all just reference the original String, no heap allocations or data copying needed.
error-handlingRust’s error handling is precise and curious - and in this article, we are going to take a look at why that is the case. I’ll introduce you to the basics of errors in Rust and then explain some more advanced concepts of dealing with errors.
async bookThere is more to a programming language than the language itself: tooling is a key element of the experience of using the language.
The same applies to many other technologies (e.g. RPC frameworks like gRPC or Apache Avro) and it often has a disproportionate impact on the uptake (or the demise) of the technology itself.Tooling should therefore be treated as a first-class concern both when designing and teaching the language itself.
The Rust community has put tooling at the forefront since its early days: it shows.
We are now going to take a brief tour of a set of tools and utilities that are going to be useful in our journey. Some of them are officially supported by the Rust organisation, others are built and maintained by the community.
javaIt's been a long time since I properly learned a new language—computer or human. Maybe 25 years. That language was Java, and although I've had to write little bits of C (very, very little) and JavaScript in the meantime, the only two languages I've written much actual code in have been Perl and Java.
I'm a co-founder of a project called Enarx, which is written almost entirely in Rust. These days I call myself an "architect," and it's been quite a long time since I wrote any production code. In the lead-up to Christmas 2019, I completed the first significant project I've written in quite a few years: an implementation of a set of algorithms around a patent application in Java. It was a good opportunity to get my head back into code, and I was quite pleased with it.
Here are some of my thoughts on Rust, from the point of view of a Java developer with a strong object-oriented background.
macrosThis is the first post on my new series "practical rust bites" that shows very tiny pieces of rust, taken out of practical real projects. So this article will be super short, easy to follow and hopefully helpful to find your way into the rust eco system.
When I started learning Rust I made the mistake of following the advice to read The Book first. While it's a great resource, it's pretty overwhelming for a beginner to get told "If you'd like to learn this programming language the best way to start is to read this 20 chapter book!" Most people give up before they even get started when they get advice like this. Nobody ever told someone to read a 20 chapter book just to get started with Javascript or Python. Rust's learning curve is no joke but you gotta give the people what they want, and they want to program, not read about programming. Programming is fun and reading about programming is not as fun.
I try to contribute to projects I use in my daily routine to feel the impact of my contributions and be aware about the use cases and the project itself. I use Rust analyzer in VsCode everyday when I write Rust code and I had an issue with it. The issue was a kind of false positive given by rust analyzer.
I decided it could be an opportunity to contribute to the project and learn a lot of new things. At the same time I also made other pull-requests to improve auto completions and assistances.
ownership-and-borrowingI want to start the series with multiple mutable references. We’ve just learned about ownership, and it doesn’t look that hard. We follow some simple rules, we even managed to write some code, see some ownership errors, and fix them.
How to set up Sublime Text 4 for Rust development.
Welcome to the next part of our Rust programming mini-series. With this part we skim through basic Rust concepts like traits, and generics. These topics are complex enough to require some shift in thinking paradigms from programmer accustomed to OOP like C++/Java in order to be used with ease.
Welcome to the Tour of Rust. This is meant to be a step by step guide through the features of the Rust programming language. Rust is often considered a language with a steep learning curve, I hope I can convince you there's a lot to explore before we even get to complex parts.
Hello and welcome! This might be the first time we meet so I thought I’d start this post off with a short introduction.
I’m Joe and previously I worked with JavaScript building web apps and mobile apps. Now, I’m learning Rust both for personal reasons and work-related reasons. I work as a Developer Advocate in the DevTools/Languages space for the open source team. One of my primary focuses for this half of the year is Rust!
As a new Rustacean, I knew it would be best to look for something beginner-friendly such as finding a small bug to fix or contributing to documentation. I am happy to say I found something within my abilities and achieved my goal!
While staring at the keywords documentation in the standard library, it occurred to me that an interactive playground could be a really effective way of explaining/teaching Rust's syntax.
explaine.rs is an interactive playground to explore the syntax of the Rust Programming Language.
You can write any Rust code in the editor and the analyzer will allow you to click/tap on different keywords, punctuation elements and other bits of code and help you understand what they mean.
A test-driven workshop to learn Rust by building your own JIRA clone!
If you’ve read our article on Rust’s Ownership or if you’ve written your first few programs and wondered what’s the difference between String and &str, you’re most likely aware that there’s the notion of references in Rust. References enable us to give things like functions an data structures access to values, without transferring ownership. Or, in other words, without moving them. In this article we’re going to explore references a bit further and take a closer look at some interesting characteristics.
Most likely, soon after you’ve started your Rust journey, you ran into this scenario where you tried to work with string types (or should I say, you thought you were?), and the compiler refused to compile your code because of something that looks like a string, actually isn’t a string.
In part one we started an initial exploration of the Rust programming language, using a small programming problem as a starting point. The problem was:
Given a string which contains comma-separated values, transform it into a list of Globally Unique Identifiers (GUIDs) if and only if every value is a valid GUID. Ignore whitespace and newlines.
The following Rust function was given as a candidate solution, which proved to capably solve the problem while retaining excellent runtime performance:fn extract_ids(input: &str) -> Option<Vec<&str>> {
input
.split(',')
.map(|s| s.trim())
.map(|s| Uuid::parse_str(s).ok().map(|_| s))
.collect()
}This is not necessarily the most optimal way to solve this problem, but it is succinct and correct and good enough for our initial purposes.
Is it obvious, though, how it works? Let’s unpack it.
Rust is an exciting new programming language that makes it easy to make understandable and reliable software. It is made by Mozilla and is used by Amazon, Google, Microsoft and many other large companies.
Rust has a reputation of being difficult because it makes no effort to hide what is going on. I’d like to show you how I start with Rust projects. Let’s make a small HTTP service using Rocket.
- Setting up your environment
- A new project
- Testing
- Adding functionality
- OpenAPI specifications
- Error responses
- Shipping it in a docker image
You can probably tell by the title of this article that I’m working on learning Rust. Prior to the past week, I had no experience at all in the language, so it’s very fresh, and it’s been quite an adventure so far. My purpose in learning Rust was originally out of individual interest, but I quickly became interested in its potential for becoming a key portion of Apollo’s CLI toolchain. This article is just a brief overview of my experience learning Rust so far, and where I see myself going with it in the future!
csharpIt’s a dark, gloomy February night and I can hear the rain pelting against the windows of my office, behind me. It’s late, but I’ve lost track of time. I am puzzling over some code, kind of hacking away at it, trying to gain clarity. On my second monitor there is a Discord channel running, which I’m trying to follow while experimenting on my own with the code. The book on my desk is open to Chapter 13 and I occasionally refer to it. Something about the problem I’m working on is not quite clicking, but I can feel it…I’m getting close to understanding.
The book is The Rust Programming Language and the Discord window is showing the #beginners channel on The Rust Programming Language Discord Server.
javaI’d like to take a change for this blog and leave the more bleeding edge topics to focus on perhaps one of the most important things one can do in the Rust community: teaching new Rust developers. I’ve been thinking about how best to approach teaching Rust to those used to working with Java, in order to bring a group of developers up to speed with the language for a new project.
Java was the language I learned & abused in university, so my experience with it is somewhat anachronistic and I haven’t made any real attempt to keep up with the language. When I last wrote Java, if you wanted to pass a function as an argument, you had to declare a new interface or wrap a function in Callable<T>. Java has come along way since then. It’s added features that have a clear influence from functional programming and the ML lineage of langs. I’m talking about lambda’s, Optional types, etc. This article isn’t going to tell you to write everything in Rust, or that you need to throw out all your Java code. Java is a great language with valid use cases. I want to explore some comparisons between Java and Rust for the budding Rust programmer.
Before we begin, let me point out that the aim of the series is to give a gentle introduction to Rust programming language supported by some practical examples. Please note that this is not by any means a systematic introduction to Rust, but rather a coffee break story for those who heard a little bit about Rust and want to get better understanding of the features of the language.
For actual learners struggling hard with Rust these articles can be also of interest, mainly because of a possibly different perspective on selected topics or bringing up some details that may have escaped your attention while reading more comprehensive sources.
In this part we will discuss enums, pattern matching and, what is very characteristic of Rust, Options.
asyncA while ago I realized that I was a visual learner which can be frustrating at times since some concepts might take me a bit longer to fully understand until I create the proper mental image(s) for it (or somebody else does it for me).
When I started wrapping my head around Async programming in Rust I felt like I was missing some of those images. What follows is my attempt visualize the concepts around async programming.
I’ve been trying to learn Rust lately, the hot new systems programming language. One of the projects I wanted to tackle with the speed of Rust was generating 3D polyhedron shapes. Specifically, I wanted to implement something like the Three.js IcosahedronGeometry in Rust. If you try to generate icosahedrons in Three.js over any detail level over 5 the whole browser will slow to a crawl. I think we can do better in Rust!
ownership-and-borrowingThis beginner Rust tutorial, unlike most others, features ownership front-and-center. Short examples highlight the practical consequences of ownership. I'm no Rust expert. However, I have tried to choose examples that speak for themselves, and descriptions that jibe with reputable sources when possible.
This is the first part of a mini-series of articles addressed to all who want to get familiar with Rust programming language. No previous background in Rust is expected, but understanding of basic concepts of programming languages may be of help. The articles try to skip trivia not to bore the reader, but have to bring them up occasionally for clarification purposes.
In order to increase fluency in a programming language, one has to read a lot of it. But how can you read a lot of it if you don't know what it means?
In this article, instead of focusing on one or two concepts, I'll try to go through as many Rust snippets as I can, and explain what the keywords and symbols they contain mean.
Ready? Go!
asyncWith the new std::future way of doing things and tokio slowly reaching maturation, it's time to look at updating the libraries out there that are using the old ways. For one of my libraries, tmq, a Tokio ZeroMQ library, there is some awesome work already done to get this updated.
But, I thought it pertinent to at least get my feet in the water to see how hard it would be, from a library maintainer perspective, to update to std::future. For this effort, I chose my small library: mpart-async. You can see the changes I have made by comparing the versions here. This blog is a small collection of notes & gotches I found when porting code across.
javascriptIn this article we will build a simple command line program that returns the word count of a file. This will essentially be a simpler version of the Unix utility wc, written in Rust. The goal of this article is to give an introduction to some core Rust concepts for readers who might be more familiar with web-focused languages such as JavaScript and Typescript. Therefore, the Rust code examples will be compared to similar code and concepts in JavaScript or TypeScript. This guide also assumes no prior knowledge of Rust or related tools, but it does assume you have node installed on your machine already.
The aim of the exercise is simple. Start with an empty vector of unsigned integers - as it does not implement the Copy Trait - and create different types of functions and closures to push a new number to the original vector. Now that the goal is set, let's create and instantiate an empty vector.
The book is written so that we newbie Rustaceans can easily dive in the language and get productive as fast as possible. This book is not an attempt to teach you Rust, it simply provides question for practise and it is not a tutorial.
wasmRust slowly but steadily progressing into the world of web development.
Rust is easy to get started (if you wrap your head around the ownership model) and backed by an awesome community that is ready to help.
Rust provides the first class support for the WebAssembly. Rust and WebAssembly toolchain makes it easier and faster to get started with WebAssembly.
JavaScript is easy. They enabled millions (even billions) of developers to start writing applications. The fast feedback loop while development and simple API. JavaScript provides a good performance. When optimised correctly, JavaScript may yield a better performance.
The performance that JavaScript provides is not reliable and consistent. Any optimisations to increase performance is not consistent across various JavaScript engines. This makes it difficult for developers to give a better optimised and consistent performance with JavaScript.
The Educational Products platform provides support for a vast variety of modern programming languages, one of which is Rust, a modern multi-paradigm system programming language combining memory safety and high performance. It is best known for its reliability, efficiency, and an environment focused on your productivity. There are a lot of systems written in Rust, from low-level embedded ones to large scale web-servers.
Rust has one of the most supportive and engaged communities and, as a result, a very friendly onboarding process supported via “The Rust Programming Language” book and Rustlings.
We are excited to introduce the Educational Products adaptation of the Rustlings course – now available via the EduTools plugin!
I am Benoit Chassignol, technical consultant at LinkValue, currently working at M6 Web as FrontEnd developer on embedded solutions. I graduated in Multimedia Communication, and I have been working as a graphical designer 3 years. I started my journey as a developer by learning frontend integration by myself, after which I learned more about Javascript with ReactJS, Typescript, and now Node.
With that in mind, let's talk about my personal experience as I am about to reach a new turning point in my professional life.
Together we're going to build a very basic calculator command line tool in this article. It'll be far from complete but it'll teach you a lot about Rust. I want to build a program that will take input on a command line through stdin and on pressing enter will perform the calculation and spit out the result. I'll go through a few iterations to get to where I want in order to help introduce as much as I can along the way. I'll make mistakes on purpose (honest) and show you what to do to correct them.
Of late, my main side project has been rug, a stripped-down Git implementation which I’m building in Rust. I’m following along James Coglan’s excellent book Building Git where he lays out how he went about building the same project, in Ruby. In essence, my project boils down to porting James’s code1 into Rust. But why bother with this at all?
My focus for this post (and other posts in this potential series) is to focus on other language features and idioms that may be unfamiliar to managed-language developers.
In my first post in this series, I talked about the fact that Rust does not have the concept of null. Rust does not have the concept of exceptions or the associated concept of try-catch blocks. Instead, in Rust we use an enum type called std::result::Result<T, E> . The T in the generic signature is the return result. The E represents the type of the Error should one occur.
So I started learning Rust a while ago and since my post about what I thought of Go was popular, I decided to write about what my first impressions of Rust were as well.
Hello folks, in this blog, we will be learning how to create our own Blockchain, which is a technology behind Bitcoin. Blockchain technology has been called the
I’ve been learning Rust lately. I have also got back to playing futsal with some friends. Weirdly enough, these two worlds intersected a few weeks ago. This is a summary of my experience learning Rust. Starting with reading about it here and there, eventually getting the book, doing a Hackathon project at Onfido using it and then spinning up my own personal project as a way to further experiment with the language.
Zines (pronounced “zeens") are small publications that come in the form of mini pamphlets or magazines!
Seeing Rust as a potential successor to TypeScript, we go through through the Rust Book with TypeScript in mind.
I recently finished my first rust project - a command line utility called “what” that displays network utilization information. As a newcomer to rust, this project offered quite some challenges for me. This post is a write up of one of them, going into detail on the parts that I personally found most difficult to understand.
In this first post, I’d like to talk about implementing a job queue to resolve IPs into their hostnames by querying a remote DNS server.
ownership-and-borrowingIn this article we'll take a closer look at Rust's Ownership model and how it manages memory.
My name is Jesse, and this is an introductory Rust tutorial for developers who like learning by doing. The purpose of this tutorial is to develop intuition about toolbuilding in Rust–specifically, to learn how to think and build in Rust. Our goal is to produce a very basic command line compiler that will turn a basic Markdown document containing headings and paragraphs into an html file. To do this, we will start from scratch by building a simple “Hello, World!” executable. Then, over the course of six chapters, iterate and expand until finally we can compile a very simple Markdown file into valid HTML.
People seem to like Rust a lot! But if you're coming from JavaScript, not everything may make a lot of sense at first. But no problem; this guide is for you! Because I think Rust and JavaScript are really similar in many ways; to the point that if you know JS it's mostly a matter of getting the hang of some of the nuances before you can more or less get the hang of Rust.
Over the course of my internship at the Microsoft Security Response Center (MSRC), I worked on the safe systems programming languages (SSPL) team to promote safer languages for systems programming where runtime overhead is important, as outlined in this blog. My job was to port a security critical network processing agent into Rust to eliminate … An intern’s experience with Rust Read More »
This year’s #hacktoberfest became a great opportunity for me to start learning Rust. In this article, I will share a couple of tricks about how to start mastering this relatively new technology.
ownership-and-borrowingThis blog post will take a deep dive into the Rust world of mutability. By deep dive, it means the blog post is considerably long. So it will take time to go through the different examples. The topic we will dive through is specific but we will have to go through various Rust concepts: Ownership/Borrowing, Lifetimes, Unsafe, Sync, Closures, Macros and more. This might be intimidating and I think this is where many developers are put off.
This is a introduction to Rust, intended for developers that already know another language. In the examples, Rust is compared with TypeScript, JavaScript or Java, sometimes with C++ or Kotlin.
This tutorial will show you how to write a roguelike in the Rust programming language and the libtcod library. In this update the Asciidoctor documents were changed to allow generating the final source files at the end of each chapter directly from the tutorial text.
Traits are the abstract mechanism for adding functionality to Types or it tells Rust compiler about functionality a type must provide. In a nutshell, Traits are Interfaces of other languages. In this article, I’ll talk about some deep concepts of Traits in Rust Programming.
memory-managementIt feels like an eternity since I’ve started using Rust, and yet I remember vividly what it felt like to bang my head against the borrow checker for the first few times.
I’m definitely not alone in that, and there’s been quite a few articles on the subject! But I want to take some time to present the borrow checker from the perspective of its benefits, rather than as an opponent to fend with.
This is a quick & dirty guide to building a web app using an all-rust stack: Diesel as an ORM, Rocket as a web framework, and Seed for the [WebAssembly] frontend. Read this book to learn how to quickly throw together a prototype application, all with your favorite programming language.
I really like learning new programming languages, especially the ones that make you reconsider some fundamental assumptions you make when writing code or designing a piece of software. I've been playing with Rust for some time now and I can definitely say that it's one of these languages.
Rusty Weather: My first Rust App I have wanted to learn a new language and framework, as well as I, know C# and .NET for many years. I have dabbled in several different ones, but the only one that I seemed to come back to continually was Python. After trying all kinds of languages over the last couple years, I decided I would give Rust a try. I have only been a consumer of garbage collected runtimes, so I was a bit hesitant to give it a try.
I like having a difficult task to solve. One of those is my endeavor to truly learn a low-level programming language. My goal is to write really fast and safe APIs to supply data for visualizations on dashboards and in reports. I want to gain a fundamental understanding of how the language works, of the concepts and philosophy applied instead of trial-and-error with lots of googling. Sometimes it just makes sense to take your time.
I used to live in the single-thread JavaScript happy-land where the closest thing to working with threads I ever did was communicating between a website and a Chrome extension. So when people talked about the difficulties of parallelism and concurrency, I never truly got what the fuss was about.
As you may have read before, I started learning Rust a few weeks ago, re-writing a text-based game I previously made with Vue. It's a survival game in which you must gather and craft items to eat and drink. It has no winning condition other than trying to survive as many days as possible. I managed to get most of the game features working, but there was an annoying bug: if the user left the game idle for hours, it didn't check for the stats until the user interacted again. You could live for hundreds of days without doing nothing!
I knew this could be solved with threads, so I finally gathered the courage and read the chapter Fearless Concurrency of The Rust Programming Language.
Build a basic chat app with the Rust Programming Language.
From<Elixir>, Into<Rust>. I loved learning the Elixir language and how its pragmatic supervision trees and process model taught me the value fault tolerance as a quality of code than of infrastructure. Having safety and failure recovery as an idiomatic culture and mindset of the language made me a better thinker and developer. As a personal preference then in selecting new languages to learn, I look for potentially new perspectives and insights that it ascribes to its pilgrims. In general, a good learning curve is a good indicator since it has much to teach.
ownership-and-borrowing guiImplications of Rust's borrow checking and memory ownership on GUI development (simple case)
Well I am a professional programmer for over a decade now, so my brain is trained to remember algorithmic thinking. But it's not trained to retain music theory. Which leads me to my beginning life-hack of music theory .... write a music theory shell application in rust to help me understanding the underlying mathematical structures by implementing them. I will try to make this an ongoing regular exercise to combine of my two currently favorite things: Rust and Guitar playing / Music.
In Part 1, we introduced Rust and built a little program that allowed us to get all the GameDay links for a particular day. Today, we’ll dive right into code and begin the process of building out a complete game from the xml files. As much as possible, I’ll try to explain important concepts along the way as we build out the application. My goal is to deliver a crash course into baseball-centric programming in Rust, complete with code, theory and practical use, without getting too much into the weeds.
From time to time, major league teams will post job offers on FanGraphs. Most of these postings, if not all of them, ask for a level of proficiency in Python or R. While these languages have built up tremendous ecosystems, especially for data science, they are limited in the amount of data they can handle.
This is not a flaw in either language, rather a design choice. Without getting into the weeds too much about language theory, each language plants itself somewhere on the performance/ease-of-use spectrum. Nothing in today’s piece should be construed as a critique of Python or R. Quite the contrary. Python and R are the bedrock languages of the data science worlds.
Today, I would like to introduce you to Rust, a modern systems programming language that aims to be, in their words, “A language empowering everyone to build reliable and efficient software.” I can personally attest to this being the case.
Learn WebAssembly while building a Wasm-based QR decoder for the browser.
ownership-and-borrowingOne of the biggest Rust’s pros is unique ownership system. Unfortunately, it is also one of the hardest thing to learn. In this article I will try to explain it the same way I had learnt it and how I introduce people to one.
Information overload and I’m still trying to find a bigger project I can work on that interests me. I have the Interpreter to work on still, and that will take some serious work! But I’m also thinking of going back to small systems of my programming past and playing with writing them in Rust. In the meantime, I thought I’d sneak around the Rust #beginners channel on Discord and give everyone a peak at some questions (and hopefully some answers) that beginners are asking about their early Rust code.
I thought it would be a nice start to just take existing code and try to see how well it would translate from C++. I simply took my previous CHIP-8 emu and started to chip at it with the help of the docs. If you want to dive right in, here’s the code on GitHub. Anyway, it was my first go at Rust so go easy on me! =)
We will continue the previous post with two complementary examples. Both will demonstrate the capability of enums to push various language constructs and states into the type system. As an example for it, imagine we could create a type which represents an if statement, and then hand over instances of it around our program. Enums tends to work better than using the underlying concepts for various reason, starting from the complexity of the borrow checkers and lifetimes, through the powerful type system in Rust. And not less important, allowing you to code common patterns as functions, in a way otherwise wouldn’t be available to you. We will see all of this today. Later in the series, we will revisit those examples, and we will demonstrate how well they can compose with other code we can write.
This article demonstrates how to perform basic file and file I/O operations in Rust, and also introduces Rust's ownership concept and the Cargo tool. If you are seeing Rust code for the first time, this article should provide a pretty good idea of how Rust deals with files and file I/O, and if you've used Rust before, you still will appreciate the code examples in this article.
We continue our series “Rust for OOP” with Enums & Pattern Matching, one of my preferred features of Rust. I didn’t hear about it before getting into the language, yet immediately fell in love with it. Enums are simple, expressive, reducing code bloat, enable encapsulation, easy to understand, and reason with. It also enables many useful design pattern.
Rust has a “community of developers empowered by their tools and each other” (via Katharina Fey in “An async story“). The Rust community helps each other through effective narrative documentation and attention to error messages, and the robust tooling around Rust drives momentum, overcoming some of the natural hurdles when diving into a new language.
Here’s my list of essential rust tools (so far).
Rust 1.32.0 introduced a macro dbg! for quick and dirty debugging with which you can inspect the value of a given expression. Rust 1.35.0 announced an improvement in this macro to make it more usable for Rustaceans. Now you can trace any fine and line number using this macro without passing any parameter.
Environment variables plays a vital role in setting up a large scale project in real time environment. This blog explains env crate and env! macro in Rust.
I left a comment on HN about how I teach new users not to clone, and someone asked me if I’d written more, so here we go!
When new users are writing Rust code, it’s reasonably common to “fight with the borrow checker”. I personally find this to be quite a misnomer, as it is not a fight so much as a misunderstanding of the nature of Rust’s semantics. In an attempt to make the errors go away, users will understandably take the path of least resistance, according to the docs they’ve got. They dutifully read up, and eventually land on the clone method. This makes the errors go away! Great! Except if you were my newbie, in the code review I’d tell you clone is banned unless you can tell me why you need it.
Rust is one of the major programming languages that’s been getting popular in recent years. It has many advanced high level language features like Scala. This made me interested to learn Rust. So in this next series of blogs I will share my experience with Rust from a Scala developer point of view. I would like to explore how these two language approach things. I would like to explore the similarities and their differences.
This is fifth post in the series. In this post, I will be talking about domain models.
The basics of Rust project layout are simple, and common to many other languages. You have the artifacts of your project. The basics artifacts are executables(binaries) and libraries. You use binaries whenever you want to produce a runnable application. For reusable code, use libraries. Nothing remarkable in Rust. In my projects, I prefer to write almost everything inside libraries, as one can never know when he will reuse a piece of code. Usually, I want my executable to be a thin wrapper around my libraries. Rust has a uniform name for a single library or binary: crate. Meaning crate is either an executable or a library. Creating either a library or a binary crate is straightforward.
I implemented an image processing experiment in Rust and was positively surprised.
While working on my simple chat program, I’ve identified some aspects of Rust, which mastering them will probably turn my code to be more idiomatic. These aspects will turn into a blog series. I’m far from mastering those aspects of Rust, but I’ll share what I’ve learned so far. And how I’ve used it in my chat project.
A beginners guide to using StructOpt for parsing command line arguments.
I wanted to learn Rust for some time, but wasn't motivated enough. Finally, there was an opportunity: Every year in December, there is a coding challenge called Advent of Code. So I decided I will use edition 2018 as a motivator to learn new programming language.
Not that long ago I decided to start learning Rust. While it has a lot of useful resources online and a very friendly community there's still things that are weird to me. That's because Rust is a very different mental model than the frontend mental model I'm used to.
That means that things that are obvious to a lot of people are not obvious to me. So I'll try to document some of these things from the perspective of a frontend developer. This time on using modules.
Modules are one of the better ways to organize your code and reuse it wherever it is necessary. Rust module system is completely different from what I've used in Languages like JavaScript, GoLang and Python. When I started to learn this pattern, I didn't really like it but, after using them for sometime, now I really like the way it works.
Although I’m a beginner in Rust, I would like to share the process behind a toy application that I developed recently. It’s a very simple command line tool that prints an image file using ASCII symbols directly to your terminal.
This blog is for new Rustacean, who's just started exploring Rust. Demonstrating a scenario that is possible because of the Non-lexical lifetimes feature. This feature was introduced in Rust 2018 edition. Earlier than, in Rust 2015, it was not possible.
This tutorial was part of a whorkshop which took place at the Rust Community Stuttgart on April, 17th 2019. We will develop a Hangman text based console game within this tutorial.
ownership-and-borrowing pythonFollowing the previous post, here I am going to introduce the key concepts of Rust — Ownership and Borrowing.
This article will outline an overview of the why and how async exists in Rust.
Pretty-print debug, unimplemented!, ”..” struct literal operator, Pattern match guards, and Padding format operator.
The intended audience is newly started Rust users, especially if you have been using mostly garbage collection to manage memory recently. The explanation is very machine-oriented, but I promise this way is faster than fighting the answers out of the compiler case-by-case. (Elaboration on this and other choices is at the end.)
Rust’s memory management is slick enough to barely notice you’re doing any. It can make explaining compiler errors very opaque. If you are already familiar with or willing to learn bare-minimum microarchitecture, just the basics of function execution and memory usage, this article is an efficient crash course for finding the low resistance paths to writing natural Rust.
In my journey for better understanding of anything I tend to always return to the basics.
To often we base our assumptions on blind 'guess-timations' where we don't understand why something is actually happening but we observed that certain patterns ...
We will go through on how we can build command line tools with Rust, and maybe have fun along the way.
ownership-and-borrowingRust has a perception of being a very difficult language to learn. I had a similar experience, but just as I was told, there is a point where things start to get a lot easier. This post aims to describe the hard parts that I had to get through in order to start being productive with Rust in the belief that this may help others get over the hill to that sweet spot of infinite bliss and productivity. In this post, I’m going to cover references and borrowing.
Rust is different. You can pick up Python or Ruby over the weekend, create a first CRUD application and be happy with the results. With Rust… with Rust you will struggle to pass a String to a different method, change and return it. You then will order the Rust book, see its size, *sigh* and get started. After a few weeks fighting through the book after work, you give up and wait until someone else creates an easy-to-follow tutorial. Here is your “easy” tutorial.
Python developers encompass a huge variety of developers. I am going to target use cases for a particular set of python developers: Machine Learning developers. But for others too, it might prove to be useful.
Do you want your fields to be private but got stuck in accessing them from other module. Then this blog let you know the ways to access the private fields as well as which one is safer way.
Command Line Arguments are used to control program from outside instead of hard-coding those values inside the code and supplied to program when it is invoked. In this blog, I’ll demystify you how to use Command Line Arguments in the Rust Programming Language.
I started learning Rust in 2018. I completed my work in an open source project and was thinking about learning a new programming language. My motive was to learn a language that allows you to control the lower level of a high-level programming language. I considered learning Golang, but, in most of the online articles I learned that Rust (being a system programming language) gives you more control than Go, however, the learning curve is far steeper than Go. I had no worries about deadlines or time, therefore I chose Rust.
If you are coming from Java background, where you have used Exception Handling extensively and you have started working on new language like Rust, which doesn’t support Exception Handling then you will be forced to think that does such kind of world really exists?
I heard of Rust back in 2015 at a meetup. I have since spent time with Rust on and off. In 2018, I had more time to explore Rust. Thinking of using blogging as a learning tool while going further down the rabbit hole of Rust, I setup this blog. In this post, I would like to briefly walk through some important features of the language.
Structuring of data in the correct way is very important. In java and C++, we use Class & Object. In C, we use structures, unions, and enums. We know that Rust adopts some features of functional programming and OOPs. Class and object is the main weapon of OOPs but how does Rust provide that functionality?
An introduction to the Rust programming language for Node developers.
I spent the month of January playing with Rust in my free time. In this article I'm going to share some quick notes on some of the mistakes I made while learning, the resources I used to get unblocked, and what I took away from the experience. My hope is that I can save other Rust newcomers a bit of time by documenting some of the things that I banged my head into. Now, it's worth noting that I'm still a Rust beginner, so if I got something wrong please let me know so that I can update it.
To learn Rust, I implemented an audio game. There were some things I liked and some things I didn't. Some things were easy and some were hard. And there were plenty of libraries that were a joy to use.
Rust has been on my radar for some time and I aspire to become proficient with the Rust Programming Language in 2019. Initially, my interest in Rust was sparked by the memory ownership model. With WASM support going mainstream I thought I’d give Rust a deeper look and have enjoyed my experience so far. This post is a living post that will continue to be revised and appended as I learn more about Rust.
If you have made up your mind to learn this modern programming language in 2019, this is a small attempt for new learners to have an unofficial learning path for getting to know and mastering Rust. I am still learning Rust and I will update post as and when required. This is iteration 1.
I previously wrote about Supernova, and my experience contributing to a project in a language I had never used before. Although my PR is still WIP status (I need to find time to get a solid grasp on Date/Time stuff in Rust, specifically using the chrono crate), I picked up some smaller issues in the interim to stay involved.
My most recent PR’s involved working off of a previous PR which introduced code linting with clippy, and taking it one step further by introducing rustfmt to help ensure consistent coding style in our CI pipeline.
A journey from hating Rust to loving it more than any other language.
How I decided to challenge myself and solve coding tasks using Rust
This is an article and a tutorial about stumbling and failing. It is about trying hard, and giving up - just to start all over again. All for the one goal - becoming the master of coding in Rust.
Welcome Back! This is the fifth post of Learning Rust series. Rust is a well designed language and safety is one of the main focus area of it. Its design decisions have been taken to prevent errors and major issues of systems programs like data races, null pointer exceptions, sensitive data leakage through exceptions and etc. So today, we are going to talk more about the concepts behind Error Handling in Rust.
I’ve got two days of Advent of Code 2018 under my belt and four stars to show for it! But I’ll be the first to admit that I had plenty of help, so I thought it only fair that I write out some of the things I’ve already learned about Rust.
Usually I take about a week to learn a new language so I can start doing some real work with it. After all a programming language (at least the high level and dynamic ones) is just assignment, calculation, branching, looping and reuse (and in certain cases, concurrency/parallelism, not gonna dive deep in defining the difference though). Well, that was true until I started learning Rust, partly for my own leisure. I still don’t feel comfortable writing a complete Rust code. Though I really like the language.
Learning Rust is hard for everyone, but it’s even worse for me because I’ve been working with Ruby during past ten years. Switching to Rust from Ruby is leaving an anything-goes hippie commune to a summer school for delinquent programmers run by a sadistic and unforgiving teacher. Why would anyone use a compiler like this? The answer is simple: to learn how to write better code.
I recently decided to put serious effort into learning the Rust programming language. I saw it coming up frequently in interesting projects (e.g. ripgrep) and kept hearing good things about it. My hesitation to picking up Rust since its 1.0 release in 2015 came from two fronts. First, I’m completely invested in Go because of InfluxDB. Second, I heard that it was not the easiest thing to learn. While I don’t normally shy away from difficult tasks, I was hesitant because I believe that many developer tools that take off do so because they are easy to use or give developers significant productivity gains. More often than not, I want to invest my time in tools that I think have longevity that will get some critical mass in the market.
Although I didn’t start writing this blog when I started writing in Rust, I remember clearly why I started writing in Rust. From what I had been doing in C#, none of it utilized multiple threads. Multi-threaded programming had always been a sore topic for me. Things like thread synchronization and message passing never really made sense to me. I learned Rust mainly due to its promises for ease of programming for multiple threads. Once hearing that things like parts of Firefox were written using it, and the Mozilla themselves were very strongly invested in Rust, I had more reason to start writing in it. I’d assume that if Mozilla were to invest so much in a programming language, it must have some sort of benefits over your usual C and C++ programming languages. Even further than that, I’ve heard of some game studios adopting Rust in their games and I was pretty much hooked at that point.
In this tutorial, we are going to create a simple command-line todo app. By the end of this tutorial, you should have a basic understanding of Rust programming language, building command-line apps in Rust, and performing file-system operations in Rust.
Understanding your compiler internally allows you to use it effectively. Walk through how programming languages and compilers work in this chronological synopsis. Lots of links, example code, and diagrams have been composed to aid in your understanding.
Understanding Compilers — For Humans (Version 2) is a successor to my second article on Medium, with over 21 thousand views. I am so glad I could make a positive impact on people’s education, and I am excited to bring a complete rewrite based on the input I received from the original article. I chose Rust as this work’s primary language.
The other day, a friend of mine who is learning Rust asked if Rust is a pass-by-value or a pass-by-reference language. For the unfamiliar, pass-by-value means that when passing an argument to a function it gets copied into the new function so that the value in the calling function and the value in the called function are two separate values. Changes to one will not in turn result in the same change to the other. Pass-by-reference, on the other hand, means that when passing an argument to a function, it doesn’t copy the value but rather the argument is merely a transparent reference to the original value. This means changes to the value in the called function change the value in the calling function since they are the same value.
At work I’m seeing more and more embedded software; over the past few years in, among others, coffee machines, forklifts, and cars. Embedded software needs to be fast and extremely efficient with hardware resources. In some cases it not even acceptable to have a tiny break for some garbage collection. So, typical tech stacks for backend development can’t be used, never mind anything that uses browser technologies. Unsurprisingly, almost all embedded software is written in C++, and, in fact, that is also what I used recently for a personal project with a micro-controller.
Looking through the remaining choices, I went past Golang, which uses garbage collection, and set my eyes on Rust. In this post I’ll describe my first impressions, some of the frustrating moments, but also the extremely impressive performance on a larger piece of code.
One of my newer hobbies recently has been learning and toying around with Rust. Recently, as part of this learning process, I've started implementing an IP address lookup service as a small side project. During the course of implementing this project I ran into what turned out to be a bit of a hurdle to tackle, specifically performing reverse dns resolution asynchronously.
My hope is that through exploring this problem, I can aid others in understanding these concepts which can be initially difficult grasp. This is especially so as the behavior and function of Futures vary wildly between various languages and runtimes.
I had been working mostly in Scala for a while, then took a diversion into Swift and Objective C. I wanted to learn another language after that, and had all but decided on Clojure. But Rust kept nagging at me — there was something about it. So I watched some videos, then read the book, and then started the Rust track at Exercism.io.
Writing the same password generator in two different languages to learn more Rust.
Demystifying one of Rust’s most powerful feature.
The language rust has been popping up on my twitter feed and my personal life more and more. It’s been promoted and presented as the ultra safe language, so naturally I decided to check it out. The upcoming series of posts “Journey into rust” will describe and document my experiences using rust, hopefully explaining certain concepts that rust does differently. This will all be written from a C++ programmers standpoint that was thought writing Object Oriented code. I encourage you the reader to think critically and correct where necessary.
On to the actual first post! After reading “the Rust Programming Language” I wanted to get my hands dirty and actually write some code. I like graphical applications and using low level graphics API’s so I decided to implement a cellular automation in rust. But just implementing cellular automation isn’t very exciting, is it? What if we could do this on the GPU…And off I went on my journey to create Conway’s game of life in rust.
When I started learning Rust, the module system did not at first seem to be a shining beacon of intuitive design. The Rust documentation is phenomenal, but there are definitely some areas that I found difficult to follow; this being one such topic. So I thought I might take a stab at writing up a guide that I think would have helped me through the awkward growing pains a bit quicker.
Today, let’s share some lessons learned from contributing to Servo, which is a great way to learn Rust.
Learning Rust to make a Mocking SpongeBob text generator
It's funny how universe aligns things, just few days ago I stumbled upon Rust koans. Already familiar way of learning and exercising, patented by Ruby programmers, where you correct tests and make them work. Also whole method of learning was similar to reading 'Little Schemer' fairly popular book among fellow Lispers. So I'll use this blog post to summarize few early impressions about Rust, and let me tell you straight away, I am loving it so far!
ownership-and-borrowingIt’s still not problem.
ownership-and-borrowingIt’s not my problem.
In this post, I’m building an ls clone. It’s pretty similar to the last post’s pwd clone. It’s called rls.
This post is the second in a series of posts where I share my experience learning Rust. I’m building out a conspiracy theories API to help me get more familiar with Rust and to have a little fun. Since I am new to Rust, I welcome any and all feedback, especially from developers who have been using Rust for quite some time, leave a comment below or contact me on Twitter. With that out of the way, it is time to put your foil lined hat on and start storing the conspiracies in a database.
But recently I decided to give Rust a go. And I must admit that I’m extremely surprised. Rust, like OCaml is a pretty low level language (it is a system programming language). After six years without seeing any pointers, I can’t say that I was happy. But the Rust compiler, by being extremely safe actually makes that easy.
If you have ever written software, you have undoubtedly asked yourself, "What language should I write this in?" It's a valid question. Does your code need to be as fast as possible? Will it be running on the Web? Will the code be on the back end or the front? All languages have their niches, and Rust is no different.
Rust is a statically typed compiled language that fills the roles that most users use C or C++ for. Unlike C or C++, though, Rust also encroaches on territory that C# and the Java™ language have dominated for much of this century: Rust is a language that is memory safe and operating system agnostic, meaning that it can run on any computer. Essentially, you get all the speed and low-level benefits of a systems language without the pesky garbage collection of those latter languages I mentioned. Excited? Yeah, me, too. Welcome to Rust!
A project used for a meetup talk about getting started with rust & wasm
Fix a series to broken Rust programs to gain a deeper understanding of Rust.
Introduction to the Rust language, standard library and ecosystem
Hello everyone! Today subject was hard to decide on. But as the previous one was pretty tedious, I decided to go a subject more easy to speak of. So, today we are going to talk about enum in Rust!
The tower of hanoi algorithm is practice for recursive function.
Rust is a new age programming language that tries to fight our bad practices in coding. It is a Systems Programming Language, which runs…
The aim of this tutorial is to take you to a place where you can read and write enough Rust to fully appreciate the excellent learning resources available online, in particular The Book. It’s an opportunity to try before you buy, and get enough feeling for the power of the language to want to go deeper.
A recent Stack Overflow survey found that almost 80% of respondents loved using or wanted to develop with the Rust language. That's an incredible number! So, what's so good about Rust? This article explores the high points of this C-like language and illustrates why it should be next on your list of languages to learn.
Hello everyone! You were waiting for it, and now you are going to get it! Ladies and gentlemen, today we are going to talk about reference and lifetime!
After dabbing in Go and Crystal, I figured I'd give Rust a try. Of course I used Vim along the way. Here are some notes I compiled after my first session.
Next up in Rust for Java Devs we have structs. They are used to hold data within a logical unit that can be passed to other functions or execute their own methods on the values that they store. Now this sounds very familiar… Java objects do the same thing. For example if you took a POJO (Plain Old Java Object) you also pass it to other methods or execute the object’s own methods. In this nature they are alike, but they do have their differences. In this post we will look into creating structs, retrieving their values, defining their own methods and how to execute them.
In this post of Rust for Java Devs we will look at creating functions in Rust. Functions in Rust do not differ that much from Java methods but there are a few little differences. There is also the topic of Rust’s lifetimes compared to Java’s garbage collection that one could argue separates the two from each other a bit more, but for this post I will focus on the structure of writing a function in Rust. Due to the similarities between the two, I believe most Java developers will have no problem in figuring out what arguments a Rust function takes, what it outputs and where the output is returned from.
Experience a sampler of Rust. You’ll get set up, then solve the first Project Euler problem in Rust. This is a language you can’t miss.
Recently, a new language called Rust has come into the spotlight, aiming to be a ‘blazingly fast’ language meant for systems programming…
Lately I’ve been working with Windows-specific APIs, so I needed to get a Windows dev environment. I’ve mostly used Linux for many years now, so for me programming on Windows feels very foreign. Getting to a point where I started being productive took a number of steps, so here they are, if only for me to find them next time.
Lifetimes are a interesting subject: a lot of people seem to gain a day-to-day familiarity with them, without fully understanding what they are. Maybe, they are truly Rust's Monads. Let's talk about what they are, where you encounter them and then how to get competent with them.