On January 17th 2023, X41 and Gitlab published a report of the source code audit they performed on Git (funded by the OSTIF foundation).
This post is based on the (great) report available here and aims to investigate how Rust mitigates some of the vulnerabilities shown in this report, but also to put some light on what it doesn’t mitigate by itself, and how a programmer can address these issues using good practices.
Security
Security, cryptography, etc. Posts 
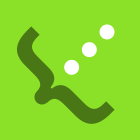
capnprotoRecent fuzz testing has uncovered a bug in capnproto-rust and capnproto-c++ that allows out-of-bounds memory to be accessed in certain situations.
fedora linuxFor the past two decades the RPM package manager software has relied upon its own OpenPGP parser implementation for dealing with package keys and signatures. With Fedora 38 they plan to have their RPM package shifted to use the Rust-written "Sequoia" parser instead.
Version 1.0. It’s here. After three and a half years of development, we are happy to announce the release of version 1.0 of Sequoia!
The release includes the low-level crate sequoia-openpgp, and a program to verify detached signatures geared towards software distribution systems called sqv.
Today I'm pleased to announce the initial release of auditable crate. It embeds the dependency tree into the compiled executable so you can check which crates exactly were used in the build. The primary motivation is to make it possible to answer the question "Do the Rust binaries we're actually running in production have any known vulnerabilities?" - and even enable third parties such as cloud providers to automatically do that for you.
We have just released version 0.19.0 of Sequoia. The release includes the low-level crate sequoia-openpgp, a program to verify detached signatures geared towards software distribution systems called sqv, and a commandline frontend for Sequoia implementing the Stateless OpenPGP Command Line Interface called sqop.
Rust has always been the programming language that reminds me the most of my game hacking days, and for good reasons. Rust is a natural fit for embedded systems like video game consoles – or rather emulators thereof. The compiler supports a high number of platforms and lets you drop down to C or assembly if necessary. Plus, the language lends itself well to implementing (reverse-engineered) cryptographic algorithms and other low-level shenanigans.
Given these benefits, it’s no surprise that I keep coming back to Rust. This time, I decided to revisit a pull request from five years ago, which has been lingering in my mind ever since. The ambiguous goal of the pull request is to port cb2util, one of my old crypto tools for PlayStation 2, from C to pure Rust.
The Rust Security Response Working Group was recently notified of a security issue affecting token generation in the crates.io web application, and while investigated that issue we discovered an additional vulnerability affecting crates.io API tokens.
We have no evidence of this being exploited in the wild, but out of an abundance of caution we opted to revoke all existing API keys.
Project Freta: free service from Microsoft Research for detecting evidence of OS and sensor sabotage, such as rootkits and advanced malware, in memory snapshots of live Linux systems.
In May and June 2020, Cure53 completed an audit of ring, webpki, and rustls. Their report (PDF) fully describes the audit, and makes for interesting reading.
First off, though, Dirkjan Ochtman (of the Quinn project) deserves a great deal of thanks for ultimately making this happen. We first discussed the possibility of an audit like this at RustFest Paris 2018. He worked with great determination for almost two years to secure a sponsor. Thanks Dirkjan!
The Cloud Native Computing Foundation (a part of the Linux Foundation) funded this audit, at the request of Buoyant who use rustls in the data plane of linkerd. So further thanks are due to Chris Aniszczyk of the Linux Foundation, and Oliver Gould of Buoyant for their support of these projects.
Finally, thanks to the staff at Cure53 for being a pleasure to work with.
Sequoia is a promising new OpenPGP library that’s written in Rust. As Rust has excellent
interoperability with C it also exposes itself as a C library in the sequoia_openpgp_ffi
crate. This would be the way that you would call this library from other programming languages,
as C often acts as the lowest common denominator.As Sequoia is making progress towards a 1.0 release, I thought that it would be time to help out by
trying to discover bugs in it by fuzzing, a technique where you generate random input to
functions and observe the execution flow in order to detect problems.
Crypto can be confusing, and there are very few examples of how to use the sodiumoxide crate. I needed to store some password hashes, and originally I was planning to use the ring crate and pbkdf2, but was encouraged to use sodiumoxide on the unofficial rust Discord channel.
I spent some time reviewing the documentation for sodiumoxide and while there were working and straight forward examples it wasn’t clear whether that was all that needed to be done to securely hash passwords, or if there were additional steps required when used in production.
I think I’ve found the answers to my initial questions and decided to write this post for my own reference and to help anyone else looking for help.
Webmaster's job is to protect the website from malicious traffic. One of the practices serving this purpose is the collection and analysis of the website's HTTP access logs. I have developed Rust library and command-line tools to help discern malicious traffic from actual visitors.
Almost 2 years ago when I joined Red Sift, I kicked off development on ingraind and its core, RedBPF, with the goal of building a better kind of security agent to monitor file access, network traffic and DNS queries in our infrastructure. We have shared our journey on this blog, and received a lot of helpful input from the Rust and Linux kernel community during this time.
Just under 1000 git commits later in the two repositories combined, we are happy to announce version 1.0.
This is just a post about something that grinds my gears a bit more than it reasonably should: I think the habit of applying for CVEs for Rust (and Rust ecosystem libraries) is silly at best and harmful at worst. I think it muddies the waters about what a vulnerability is, and paints an overly negative picture of Rust’s security situation that can only lead people to make inaccurate evaluations when contrasting it with other languages like C/C++.
Rust is a multi-paradigm language with a focus on memory safety.
Nevertheless, due to its versatility, the language possibly offers some constructions that, if not used properly, can introduce security problems, by making code misinterpreted by the programmer or a reviewer. In addition, as for every tool in the compilation or software verification field, the tools used to develop, compile and execute programs can expose certain features or configurations that, if misused, may lead to vulnerabilities.
Thus, the object of this document is to compile hints and recommendations to stay in a safe zone for secure applications development while taking advantage of the range of possibilities the Rust language can offer.
I went ahead and wrote a pure-Rust implementation of Skipjack this past weekend. You can find it on GitHub as skipjack.rs and on Cargo as skipjack.
In December 2019, MobileCoin engaged NCC Group to conduct a review of the AES/GCM and ChaCha20+Poly1305 implementations provided by the RustCrypto/AEADs crates. The intended usage context of these crates includes SGX enclaves, making timing-related side channel attacks relevant to this assessment. Two consultants provided five person-days of effort.
Today, FIDO security keys are reshaping the way online accounts are protected by providing an easy, phishing-resistant form of two-factor authentication (2FA) that is trusted by a growing number of websites, including Google, social networks, cloud providers, and many others. To help advance and improve access to FIDO authenticator implementations, we are excited, following other open-source projects like Solo and Somu, to announce the release of OpenSK, an open-source implementation for security keys written in Rust that supports both FIDO U2F and FIDO2 standards.
cargo-audit is a command-line utility which inspects Cargo.lock files and compares them against the RustSec Advisory Database, a community database of security vulnerabilities maintained by the Rust Secure Code Working Group.
This post describes the new features in the 0.11 release of cargo-audit.
httpBack in 2014 I was fetching frontpages of the top million websites to scan them for a particular vulnerability. Not only have I found 99,9% websites to be vulnerable to a trivial attack, I’ve also found that curl command was randomly crashing with a segmentation fault, indicating a likely vulnerability in libcurl — the HTTP client library that the whole world seems to depend on.
By that time I was already disillusioned in the security of software written in C and the willingness of maintainers to fix it, so I never followed up on the bug. However, this year I decided to repeat the test with software written in a language that’s less broken by design: Rust.
Here’s how 7 different HTTP clients fared.
fuzzingToday, on behalf of the Rust Fuzzing Authority, I’d like to announce new releases of the arbitrary, libfuzzer-sys, and cargo fuzz crates. Collectively, these releases better support writing fuzz targets that take well-formed instances of custom input types. This enables us to combine powerful, coverage-guided fuzzers with smart test case generation.
As a follow up to my post on distribution packaging, it was commented by Fraser Tweedale (@hackuador) that traditionally the “security” aspects of distribution packaging was a compelling reason to use distribution packages over “upstreams”. I want to dig into this further.
unsafeMy role at $work these days is to help guide a big company's investment in Rust toward success. This essay covers a slice of my experience as it pertains to unsafe code, and especially bugs in unsafe code.
When I was writing a fingerd daemon in Rust (why? because I could), one thing that took me a little while to figure out was how to drop root privileges after I bound to port 79.
One of the main selling points of Rust is memory safety. However, it is undermined every time people opt out of the checks and write an unsafe block.
A while ago I decided to check just how prevalent that is in widely used code, and I was astonished by what I've found: many popular and widely used Rust crates contain quite a few unsafe blocks, even when they're not doing anything inherently unsafe, and a surprising number of them can be converted into safe code without losing performance.
The thing about contributing to Servo is that you keep learning new things about the Web platform. Personally, I had never used messaging on the web when developing web applications, and I find it a fascinating idea.
Web-messaging enables developers to provide cross-site API’s without having to go through a server, all the while leveraging the client-side security model of the Web. And since it happens on the client, it could be more transparent to the end-user, and probably easier to block if necessary.
Implementing message-ports also raises interesting architectural questions. In an earlier Web(like, in 2017), an API like message-ports could have been implemented with some sort of cross-thread communication. In 2019 however, it’s going to have to go across process. Why? Something known as “Spectre”.
The most visible is that our Rust support is no longer optional. We’re convinced that Rust is a perfect match for Suricata, and we plan to increase its footprint in our code base steadily. By making it mandatory we’re able to remove parallel implementations and focus fully on making the Rust code better.
Fuzzing or fuzz testing is an automated software technique that involves providing semi-random data as input to the test program in order to uncover bugs and crashes. In this short tutorial we will discuss cargo-fuzz.
iotAzure IoT Edge is an open source, cross platform software project from the Azure IoT team at Microsoft that seeks to solve the problem of managing distribution of compute to the edge of your on-premise network from the cloud. This post explains some of the rationale behind our choice of Rust as the implementation programming language for the Security Daemon component in the product.
Everytime I try to learn a new programming language, I try by port my prependers (Linux.Zariche, Linux.Liora, Linux.Cephei). Despite the code simplicity , it gives me the chance to understand very useful things in a language, like error handling, file i/o, encryption, memory and a few of its core libraries.
This time, Rust is the language and I must say that I was impressed by its compiler and error handling, but the syntax is still not 100% clear to me (as you can see from my rudimentar code in Linux.Fe2O3) and I wish it had a built-in random library too. This code was written in less than 2 days, of course its not pretty, has lots of .unwrap() (already got great input from some people on Reddit to help me with that, will be addressed) so I apologise in advance.
Coverage-guided fuzzing and generation-based fuzzing are two powerful approaches to fuzzing. It can be tempting to think that you must either use one approach or the other at a time, and that they can’t be combined. However, this is not the case. In this blog post I’ll describe a method for combining coverage-guided fuzzing with structure-aware generators that I’ve found to be both effective and practical.
The Tari Labs mandated Quarkslab to perform a cryptographic and security assessment of the dalek libraries. One of the Tari Labs' projects is to implement the Tari protocol, a decentralised assets protocol. It relies on some of the dalek libraries, especially the cryptographic primitives, provided by subtle and curve25519-dalek. Moreover, the use of Bulletproofs [6], and its implementation by the authors of the dalek libraries, will allow them to enable efficient confidential transactions on the blockchain in a near future.
We only found some minor issues. We also provided recommendations on the usage of the libraries and third-party libraries.
A few years back I wrote down my thoughts on the problem of micropackages and trust scaling. In the meantime the problem has only gotten worse. Unfortunately my favorite programming language Rust is also starting to suffer from dependency explosion and how risky dependencies have become. Since I wrote about this last I have learned a few more things about this and I have some new ideas of how this could potentially be managed.
In this series, we have explored the need for proactive measures to eliminate a class of vulnerabilities and walked through some examples of memory safety issues we’ve found in Microsoft code that could have been avoided with a different language. Now we’ll peek at why we think that Rust represents the best alternative to C and C++ currently available.
Go and read the excellent blog post from Cloudflare on their recent outage if you haven’t already. I am not going to talk about most of it, just a few small points that especially interest me right now, which are definitely not the most important things from the outage point of view. This post got a bit long so I split it up, so this is part one.
Today we released a tool, siderophile, that helps Rust developers find fuzzing targets in their codebases. Siderophile trawls your crate’s dependencies and attempts to finds every unsafe function, expression, trait method, etc. It then traces these up the callgraph until it finds the function in your crate that uses the unsafety. It ranks the functions it finds in your crate by badness—the more unsafety a function makes use of, the higher its badness rating.
This post explains how to test Rust code using fuzzers. Parsers are good target for fuzzers, especially because they usually are functions that only takes bytes as input.
On Wednesday, Vincent launched a new key server at keys.openpgp.org! What makes this launch special is that keys.openpgp.org is running Hagrid —“The Keeper of Keys”—a new verifying key server, which is written in Rust and based on Sequoia. Even though the launch didn’t receive much media attention, 700 people have already verified their keys in the 48 hours since the announcement.
The Rust team was recently notified of a security vulnerability affecting manual implementations of Error::type_id and their interaction with the Error::downcast family of functions in the standard library. If your code does not manually implement Error::type_id your code is not affected.
The following are observations from when I started testing my own pure-Rust crypto library, including its dependencies, for constant-time execution. Starting with a short introduction to dudect and how it can be used to test code for timing-based side-channel vulnerabilities. Then discussing the process of discovering a short-circuit that resulted in variable-time execution, in dalek-cryptography’s subtle library and how this seems to relate to Rust codegen option opt-level.
Noise Explorer is an online engine for reasoning about Noise Protocol Framework Handshake Patterns. Noise Explorer allows you to design and validate Noise Handshake Patterns, to generate cryptographic models for formal verification and to explore a compendium of formal verification results for the most popular and relevant Noise Handshake Patterns in use today.
Today we are happy to release the source code of a project we’ve been working on for the past few months. It is called BoringTun, and is a userspace implementation of the WireGuard® protocol written in Rust.
Commonly used user space network drivers such as DPDK or Snabb currently have effectivelyfull access to the main memory via the unrestricted Direct Memory Access (DMA) capabilities of the PCI Express (PCIe) device they are controlling. This can be a security issue, as the driver can use the PCIe devices DMA access to read and / or write to main memory. In this thesis, support for using the IOMMU via the vfio-pci driver from the Linux kernel for the user space network driver ixy was implemented in C and Rust and the IOMMU and its impact on the drivers were investigated.
Know exact library versions used to build your Rust executable. Audit binaries for known bugs or security vulnerabilities in production, at scale, with zero bookkeeping.
Since our first release in 2002, there have been 69 security bugs in Firefox’s style component. If we'd had a time machine and could have written this component in Rust from the start, 51% wouldn't have happened. That said, Rust is not foolproof. Developers still need to be aware of correctness bugs and data leakage attacks.
I will show how to create various zero knowledge proofs using the Bulletproofs implementation from dalek-cryptography. The examples will be i) proving knowledge of factors of a given number without revealing the factors, ii) a range proof, i.e. prove that you know a value x such that a ≤ x ≤ b without revealing x, iii) prove that the value you have is non-zero without revealing it (without using above range proof), iv) Set membership, i.e given a set S, prove that you know an element contained in the set without revealing the element, v) Similarly, set non-membership without revealing the value of the absent element. The examples can be adapted with little effort such that they can be used in ZK-SNARK implementations like libsnark or bellman.
Rust is an open source programming language which combines security, modernity and performance. As well, it is gradually being adopted in a large number of projects. To support developers, ANSSI offers a new "Guide to develop secure applications with Rust". This guide is intended to be a living document and it’s open to all contributions from the community. The object of this document is to provide hints and recommendations for secure applications development using the Rust programming language, that allow users to benefits of the good level of trust the Rust language already provides.
Checklists are a simple yet effective component of security and safety procedures in various fields, from flight safety and surgery to network security, and of course cryptography. So here’s a couple of things you want to check when starting the audit of a crypto software written in Rust.
After the recent breach, I was curious to check my passwords against the list, but I’m a bit paranoid, so, rather than paste my passwords into the Have I Been Pwned website, I wanted to download the big text file and check my passwords against it offline, nice and safely.
I use a password manager called KeePassXC, so all of my passwords are stored in an encrypted file – a KeePass database – and I use a program called KeePassXC, a free and open-source password manager, to manage them (I wrote a beginner’s user guide to KeePassXC a while back if you’re interested!). So ideally, to check my passwords against the big list, I’d have a tool that checks all the passwords in a given KeePass database against the entire HaveIBeenPwned list of passwords, preferably against the downloaded file (i.e. “offline”), rather than the API. In other words something similar to 1Password’s Watchtower feature, but preferably offline.
After poking around a bit I decided to write it myself in Rust, with this script and this crate as useful references. Medic is a Rust CLI that can perform a variety of “health” checks on a KeePass database.
Secure enclaves provide an operating environment for code which is secure from interference by outside parties, including root users, malware, and the OS. This environment is built on three key pillars: Fully isolated execution, Sealing, Remote attestation. The Fortanix Enclave Development platform lets you write complete applications inside an enclave. The Fortanix EDP is fully integrated with the Rust compiler. Rust code that doesn't link to native libraries and that doesn't use processes or files should compile out of the box.
Memory safety violations can cause programs to crash unexpectedly and can be exploited to alter intended behavior--languages can manage this multiple ways.
The Rust programming language’s rich type system and ownership model guarantee memory-safety and thread-safety — and eliminate many classes of bugs and security vulnerabilities at compile-time.
The goals and 2019 roadmap of Rust Secure Code Working Group
The task that I have for now is to add client authentication via X509 client certificate. That is both obvious and non obvious, unfortunately. I’ll admit that I’m enjoying exploring Rust features, so I don’t know how idiomatic this code is, but it is certainly dense.
The next interesting step in my Rust network protocol exercise is to implement TLS. I haven’t looked at that yet, so it is going to be interesting.
After a few weeks of reverse-engineering, internal dogfooding, and API design discussion, we're finally publishing our gbl crate for good.
The library implements a parser and writer for GBL firmware update containers, which are used to perform secure OTA updates for certain microcontrollers.
I’d like to inform you about the recent progress on your favorite OpenPGP implementation. The Sequoia project made their first release at RustConf Rome during Neal’s talk (video, slides) about our experiences with Rust. The release includes only the low-level openpgp crate, which we renamed to sequoia-openpgp to avoid a namespace collision. In the weeks prior to the release, we moved a lot of code around, and refined our API. For example, we introduced a crypto module and moved all low-level crypto primitives there.
On October 16, 2017, we made the first commit to the Sequoia repository. Just over a year and a thousand commits later, Sequoia’s low-level API is nearly feature complete, and is already usable. For instance, a port of the p≡p engine to Sequoia is almost finished, and the code is significantly simpler than the version using the current OpenPGP library. We’ve also made experimental ports of other software that use OpenPGP, and written some new software to further validate the completeness and ergonomics of the API.
Mundane is a cryptography library written in Rust and backed by BoringSSL. It aims to be difficult to misuse, ergonomic, and performant (in that order). It was originally created to serve the cryptography needs of Fuchsia, but we’ve decided to split it off as a general-purpose crate.
Merlin is a small Rust library that performs the Fiat-Shamir transformation in software, maintaining a STROBE-based transcript of the proof protocol and allowing the prover to commit messages to the transcript and compute challenges bound to all previous messages. It also provides a transcript-based RNG for use by the prover, generalizing “deterministic” and “synthetic” nonces to arbitrarily complex zero-knowledge protocols.
I have recently discovered a zero-day vulnerability in a fairly popular and well-designed Rust crate. In this article I’m going to discuss how I did it and why it wasn’t discovered earlier, and introduce a new tool, libdiffuzz, that I’ve created for the job. A recently discovered vulnerability in Rust standard library makes a cameo appearance.
orion is another attempt at cryptography implemented in pure Rust. Its main focus is usability. This is in part achieved by providing a thorough documentation of the library. High-level abstractions are also provided, which are an attempt at guiding the users towards safe usage of the lower-level functionality of the library.
The Rust team was recently notified of a security vulnerability affecting the standard library’s str::repeat function. When passed a large number this function has an integer overflow which can lead to an out of bounds write. If you are not using str::repeat, you are not affected.
In this post we’ll go over how to get the postgres crate and r2d2_postgres working with openssl for connection pooling with TLS.
The rust community has fortunately adopted the OpenSSL bindings as the approach of choice, and the rust-openssl crate makes it easy to both bundle and consume the openssl bindings from rust in a cross-platform manner. What it doesn’t do is make encryption and decryption any easier than OpenSSL itself does.
Enter the cryptostream crate. Released on github and on crates.io under the MIT public license, cryptostream finally provides an easy and transparent way to add encryption and decryption to pipelines involving objects implementing Read or Write, making encryption (or decryption) as easy as creating a new cryptostream object, passing in an existing Read/Write impl, and then reading/writing from/to the cryptostream instead.
Rust is a new systems programming language that prides itself on memory safety and speed. The gist of it is that if you write code in Rust, it goes as fast as C or C++, but you will not get mysterious intermittent crashes in production or horrific security vulnerabilities, unlike in the latter two.
That is, until you explicitly opt in to that kind of thing. Uh oh.
This is the the last of three posts on the course I regularly teach, CS 330, Organization of Programming Languages. The first two posts covered programming language styles and mathematical concepts. This post covers the last 1/4 of the course, which focuses on software security, and related to that, the programming language Rust.
Xori is an automation-ready disassembly and static analysis library that consumes shellcode or PE binaries and provides triage analysis data.
The fastest formulas for elliptic curve operations were published by Hisil, Wong, Carter, and Dawson in their 2008 paper Twisted Edwards Curves Revisited. Their paper also describes a parallel version of their formulas, designed to execute four streams of instructions on four independent processors. Until now, these parallel formulas don’t seem to have been implemented in software. But a closer look reveals that slightly modifying the formulas allows the expensive instructions to be executed in uniform, making a vectorized SIMD implementation possible.
I implemented this strategy in Rust, targeting 256-bit wide AVX2 operations. The resulting implementation performs double-base scalar multiplication faster than other Ed25519 implementations I tested, and is even faster than FourQ without endomorphisms
Security advisory database for Rust crates published through https://crates.io.